1. Display Function Implement a function to display the contents of the patient_list array. Add code to call this function. Note that there are multiple places where this function needs to be called. Look for the // TODO comments to find the correct locations. 2. Sorting the array by Age Implement the code to sort the contents of the patient_list array based on the value stored in the age field. To do this you will need to implement code that relies on the qsort function from the C Standard library A function that compares two patient elements, based on the value stored in the age field. A call to the qsort function, which includes the array to be sorted, the number of elements in the array, the size of each array element and the function used to compare the array elements. Add code to call the qsort function, using the age comparison function that you implemented. This code should be placed just under the appropriate // TODO comment in main(). Sorting the array by Balance Due Implement the code to sort the contents of the patient_list array based on the value stored in the balance field. To do this you will need to implement code that relies on the qsort function from the C Standard library (see http://www.cplusplus.com/reference/cstdlib/qsort/). As shown in the reference, this code requires two parts: A function that compares two patient elements, based on the value stored in the balance field. A call to the qsort function, which includes the array to be sorted, the number of elements in the array, the size of each array element and the function used to compare the array elements. Add code to call the qsort function, using the balance comparison function that you implemented. This code should be placed just under the appropriate // TODO comment in main(). Sorting the array by Patient Name Implement the code to sort the contents of the patient_list array based on the value stored in the name field. To do this you will need to implement code that relies on the qsort function from the C Standard library (see http://www.cplusplus.com/reference/cstdlib/qsort/). As shown in the reference, this code requires two parts: A function that compares two patient elements, based on the value stored in the name field. Because name data is stored in an array of characters, you cannot use the relational operators (<, >, <=, … ) to do the comparison, instead you should use a function from the C Standard Library to determine the order of the names, see (http://www.cplusplus.com/reference/cstring/strncmp/). A call to the qsort function, which includes the array to be sorted, the number of elements in the array, the size of each array element and the function used to compare the array elements. Add code to call the qsort function, using the name comparison function that you implemented. This code should be placed just under the appropriate // TODO comment in main(). OUTPUT IN IMAGES #include #include #include using namespace std; // Declaring a new struct to store patient data struct patient { int age; char name[20]; float balance; }; // TODO: // IMPLEMENT A FUNCTION THAT COMPARES TWO PATIENTS BY AGE // THE FUNCTION RETURNS AN INTEGER AS FOLLOWS: // -1 IF THE AGE OF THE FIRST PATIENT IS LESS // THAN THE SECOND PATIENT'S AGE // 0 IF THE AGES ARE EQUAL // 1 OTHERWISE // TODO: // IMPLEMENT A FUNCTION THAT COMPARES TWO PATIENTS BY BALANCE DUE // THE FUNCTION RETURNS AN INTEGER AS FOLLOWS: // -1 IF THE BALANCE FOR THE FIRST PATIENT IS LESS // THAN THE SECOND PATIENT'S BALANCE // 0 IF THE BALANCES ARE EQUAL // 1 OTHERWISE // TODO: // IMPLEMENT A FUNCTION THAT COMPARES TWO PATIENTS BY NAME // THE FUNCTION RETURNS AN INTEGER AS FOLLOWS: // -1 IF THE NAME OF THE FIRST PATIENT GOES BEFORE // THE SECOND PATIENT'S NAME // 0 IF THE AGES ARE EQUAL // 1 OTHERWISE // // HINT: USE THE strncmp FUNCTION // (SEE http://www.cplusplus.com/reference/cstring/strncmp/) // The main program int main() { int total_patients = 5; // Storing some test data struct patient patient_list[5] = { {25, "Juan Valdez ", 1250}, {15, "James Morris ", 2100}, {32, "Tyra Banks ", 750}, {62, "Maria O'Donell", 375}, {53, "Pablo Picasso ", 615} }; cout << "Patient List: " << endl; // TODO: // IMPLEMENT THE CODE TO DISPLAY THE CONTENTS // OF THE ARRAY BEFORE SORTING cout << endl; cout << "Sorting..." << endl; // TODO: // CALL THE qsort FUNCTION TO SORT THE ARRAY BY PATIENT AGE cout << "Patient List - Sorted by Age: " << endl; // TODO: // DISPLAY THE CONTENTS OF THE ARRAY // AFTER SORTING BY AGE cout << endl; cout << "Sorting..." << endl; // TODO: // CALL THE qsort FUNCTION TO SORT THE ARRAY BY PATIENT BALANCE cout << "Patient List - Sorted by Balance Due: " << endl; // TODO: // DISPLAY THE CONTENTS OF THE ARRAY // AFTER SORTING BY BALANCE cout << endl; cout << "Sorting..." << endl; // TODO: // CALL THE qsort FUNCTION TO SORT THE ARRAY BY PATIENT NAME cout << "Patient List - Sorted by Name: " << endl; // TODO: // DISPLAY THE CONTENTS OF THE ARRAY // AFTER SORTING BY NAME cout << endl;
1. Display Function
- Implement a function to display the contents of the patient_list array.
- Add code to call this function. Note that there are multiple places where this function needs to be called. Look for the // TODO comments to find the correct locations.
2. Sorting the array by Age
- Implement the code to sort the contents of the patient_list array based on the value stored in the age field. To do this you will need to implement code that relies on the qsort function from the C Standard library
- A function that compares two patient elements, based on the value stored in the age field.
- A call to the qsort function, which includes the array to be sorted, the number of elements in the array, the size of each array element and the function used to compare the array elements.
- Add code to call the qsort function, using the age comparison function that you implemented. This code should be placed just under the appropriate // TODO comment in main().
Sorting the array by Balance Due
- Implement the code to sort the contents of the patient_list array based on the value stored in the balance field. To do this you will need to implement code that relies on the qsort function from the C Standard library
(see http://www.cplusplus.com/reference/cstdlib/qsort/). As shown in the reference, this code requires two parts: - A function that compares two patient elements, based on the value stored in the balance field.
- A call to the qsort function, which includes the array to be sorted, the number of elements in the array, the size of each array element and the function used to compare the array elements.
- Add code to call the qsort function, using the balance comparison function that you implemented. This code should be placed just under the appropriate // TODO comment in main().
Sorting the array by Patient Name
- Implement the code to sort the contents of the patient_list array based on the value stored in the name field. To do this you will need to implement code that relies on the qsort function from the C Standard library
(see http://www.cplusplus.com/reference/cstdlib/qsort/). As shown in the reference, this code requires two parts: - A function that compares two patient elements, based on the value stored in the name field. Because name data is stored in an array of characters, you cannot use the relational operators (<, >, <=, … ) to do the comparison, instead you should use a function from the C Standard Library to determine the order of the names, see (http://www.cplusplus.com/reference/cstring/strncmp/).
- A call to the qsort function, which includes the array to be sorted, the number of elements in the array, the size of each array element and the function used to compare the array elements.
- Add code to call the qsort function, using the name comparison function that you implemented. This code should be placed just under the appropriate // TODO comment in main().
OUTPUT IN IMAGES
#include <iostream>
#include <cstdlib>
#include <cstring>
using namespace std;
// Declaring a new struct to store patient data
struct patient {
int age;
char name[20];
float balance;
};
// TODO:
// IMPLEMENT A FUNCTION THAT COMPARES TWO PATIENTS BY AGE
// THE FUNCTION RETURNS AN INTEGER AS FOLLOWS:
// -1 IF THE AGE OF THE FIRST PATIENT IS LESS
// THAN THE SECOND PATIENT'S AGE
// 0 IF THE AGES ARE EQUAL
// 1 OTHERWISE
// TODO:
// IMPLEMENT A FUNCTION THAT COMPARES TWO PATIENTS BY BALANCE DUE
// THE FUNCTION RETURNS AN INTEGER AS FOLLOWS:
// -1 IF THE BALANCE FOR THE FIRST PATIENT IS LESS
// THAN THE SECOND PATIENT'S BALANCE
// 0 IF THE BALANCES ARE EQUAL
// 1 OTHERWISE
// TODO:
// IMPLEMENT A FUNCTION THAT COMPARES TWO PATIENTS BY NAME
// THE FUNCTION RETURNS AN INTEGER AS FOLLOWS:
// -1 IF THE NAME OF THE FIRST PATIENT GOES BEFORE
// THE SECOND PATIENT'S NAME
// 0 IF THE AGES ARE EQUAL
// 1 OTHERWISE
//
// HINT: USE THE strncmp FUNCTION
// (SEE http://www.cplusplus.com/reference/cstring/strncmp/)
// The main program
int main()
{
int total_patients = 5;
// Storing some test data
struct patient patient_list[5] = {
{25, "Juan Valdez ", 1250},
{15, "James Morris ", 2100},
{32, "Tyra Banks ", 750},
{62, "Maria O'Donell", 375},
{53, "Pablo Picasso ", 615}
};
cout << "Patient List: " << endl;
// TODO:
// IMPLEMENT THE CODE TO DISPLAY THE CONTENTS
// OF THE ARRAY BEFORE SORTING
cout << endl;
cout << "Sorting..." << endl;
// TODO:
// CALL THE qsort FUNCTION TO SORT THE ARRAY BY PATIENT AGE
cout << "Patient List - Sorted by Age: " << endl;
// TODO:
// DISPLAY THE CONTENTS OF THE ARRAY
// AFTER SORTING BY AGE
cout << endl;
cout << "Sorting..." << endl;
// TODO:
// CALL THE qsort FUNCTION TO SORT THE ARRAY BY PATIENT BALANCE
cout << "Patient List - Sorted by Balance Due: " << endl;
// TODO:
// DISPLAY THE CONTENTS OF THE ARRAY
// AFTER SORTING BY BALANCE
cout << endl;
cout << "Sorting..." << endl;
// TODO:
// CALL THE qsort FUNCTION TO SORT THE ARRAY BY PATIENT NAME
cout << "Patient List - Sorted by Name: " << endl;
// TODO:
// DISPLAY THE CONTENTS OF THE ARRAY
// AFTER SORTING BY NAME
cout << endl;
return 0;
}
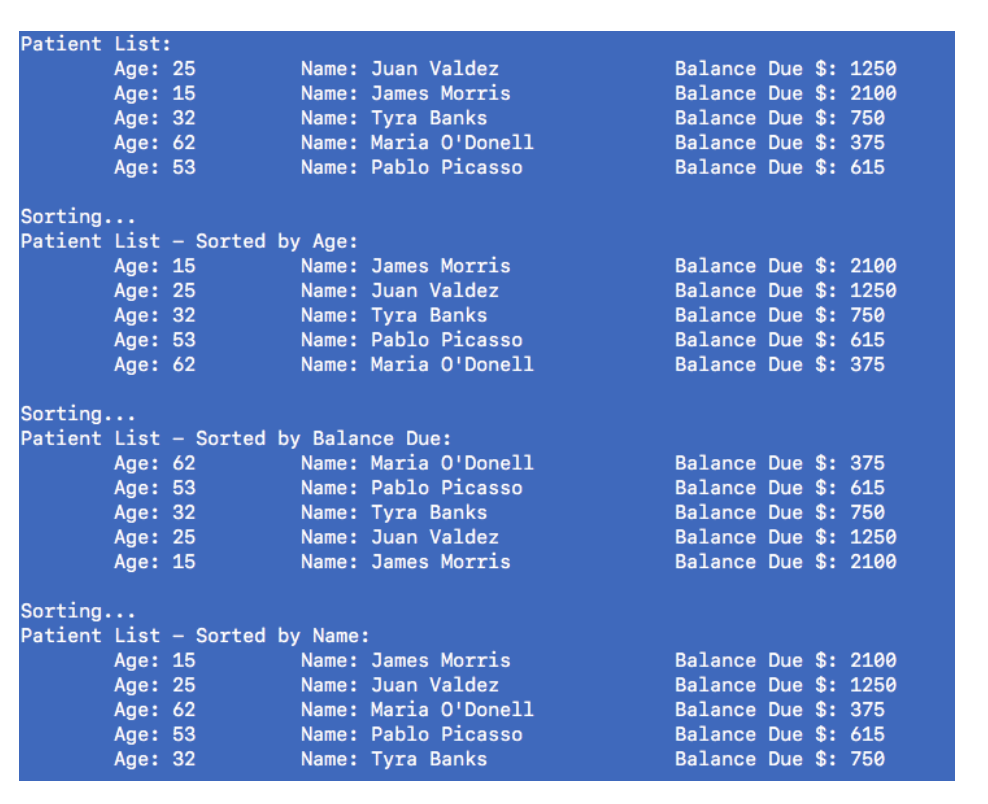

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images
