1. Given the two binary trees below: 4 (15) (18) 16 (20 5) 17 Write a method called swapSubtrees, which swaps all of the left and right subtrees in the above binary trees. Adc this method to the class Binary Tree and create a program to test this method for these 2 trees. Show the original trees and the resulting trees. Note: To test your algorithm, first create a binary search tree. - Write a method called singleParent, which returns the number of nodes in a binary tree that have only one child for the 2 trees given in the above. Add this method to the class Binary Tree and create a program to test this method. Note: To test your algorithm, first create a binary search tree. 2. Start with an empty heap, and enter ten items with priorities 1 through 10. Draw the resulting heap. - Now remove three entries from the heap that you created in the above exercise. Draw the resulting heap.
1. Given the two binary trees below: 4 (15) (18) 16 (20 5) 17 Write a method called swapSubtrees, which swaps all of the left and right subtrees in the above binary trees. Adc this method to the class Binary Tree and create a program to test this method for these 2 trees. Show the original trees and the resulting trees. Note: To test your algorithm, first create a binary search tree. - Write a method called singleParent, which returns the number of nodes in a binary tree that have only one child for the 2 trees given in the above. Add this method to the class Binary Tree and create a program to test this method. Note: To test your algorithm, first create a binary search tree. 2. Start with an empty heap, and enter ten items with priorities 1 through 10. Draw the resulting heap. - Now remove three entries from the heap that you created in the above exercise. Draw the resulting heap.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Dont use others answers please!!
follow the directions on the photo
will leave you feedback!!
Thank you!
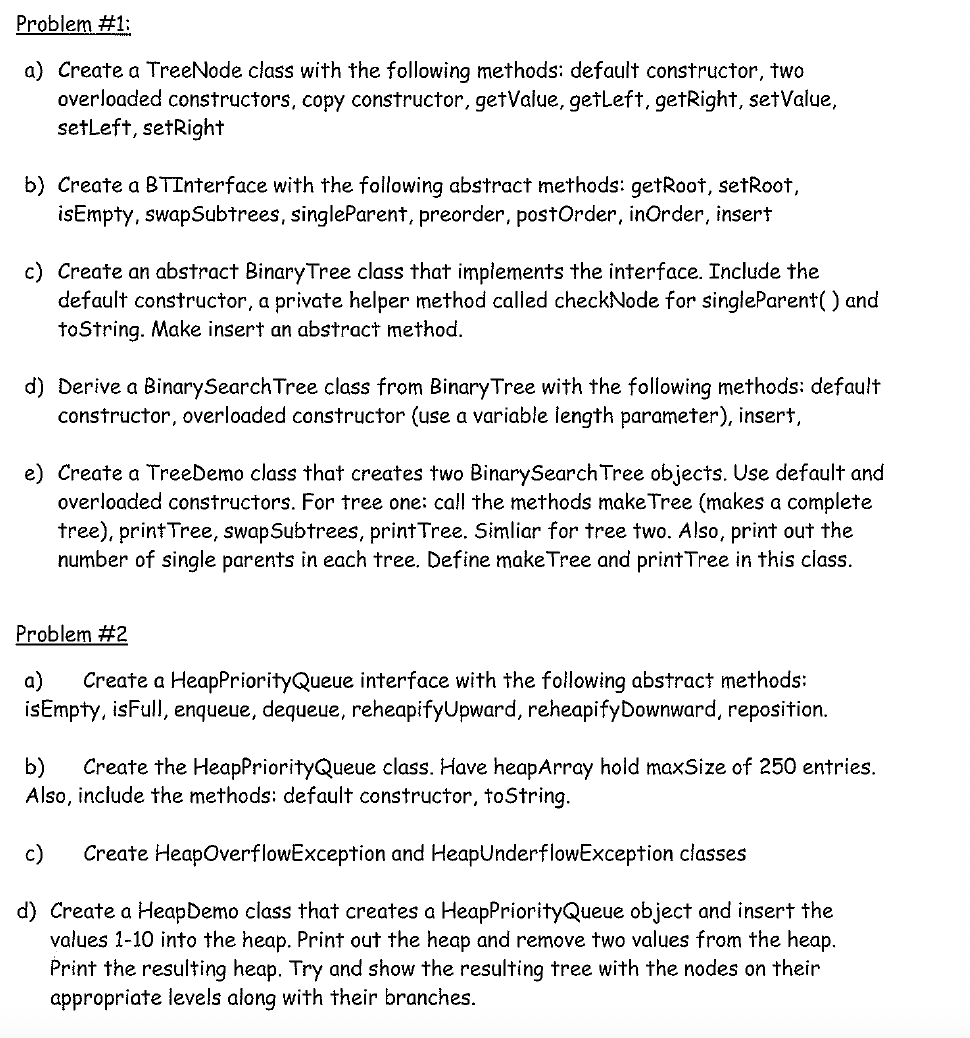
Transcribed Image Text:Problem #1:
a) Create a TreeNode class with the following methods: default constructor, two
overloaded constructors, copy constructor, getValue, getleft, getRight, setValue,
setLeft, setRight
b) Create a BTInterface with the following abstract methods: getRoot, setRoot,
isEmpty, swapSubtrees, singleParent, preorder, postOrder, inOrder, insert
c) Create an abstract BinaryTree class that implements the interface. Include the
default constructor, a private helper method called checkNode for singleParent() and
toString. Make insert an abstract method.
d) Derive a BinarySearch Tree class from BinaryTree with the following methods: default
constructor, overloaded constructor (use a variable iength parameter), insert,
e) Create a Treedemo class that creates two BinarySearch Tree objects. Use default and
overloaded constructors. For tree one: call the methods make Tree (makes a complete
tree), print Tree, swapSubtrees, printTree. Simliar for tree two. Also, print out the
number of single parents in each tree. Define make Tree and print Tree in this class.
Problem #2
a)
Create a HeapPriorityQueue interface with the following abstract methods:
isEmpty, isFull, enqueue, dequeue, reheapifyUpward, reheapifyDownward, reposition.
b)
Create the HeapPriorityQueue class. Have heapArray hold maxSize of 250 entries.
Also, include the methods: default constructor, toString.
c)
Create HeapOverflowException and HeapUnderflowException classes
d) Create a HeapDemo class that creates a HeapPriorityQueue object and insert the
values 1-10 into the heap. Print out the heap and remove two values from the heap.
Print the resulting heap. Try and show the resulting tree with the nodes on their
appropriate levels along with their branches.
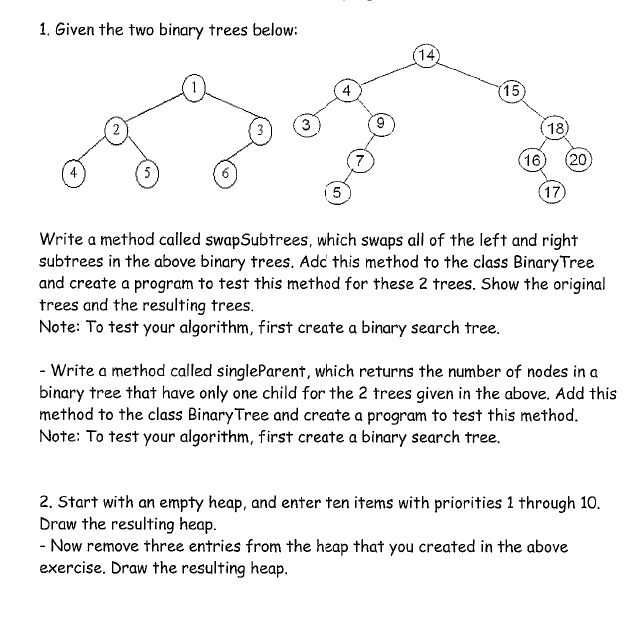
Transcribed Image Text:1. Given the two binary trees below:
14)
4
(15)
(18)
16)
(20)
(17
Write a method called swapSubtrees, which swaps all of the left and right
subtrees in the above binary trees. Adc this method to the class Binary Tree
and create a program to test this method for these 2 trees. Show the original
trees and the resulting trees.
Note: To test your algorithm, first create a binary search tree.
- Write a method called singleParent, which returns the number of nodes in a
binary tree that have only one child for the 2 trees given in the above. Add this
method to the class Binary Tree and create a program to test this method.
Note: To test your algorithm, first create a binary search tree.
2. Start with an empty heap, and enter ten items with priorities 1 through 10.
Draw the resulting heap.
- Now remove three entries from the heap that you created in the above
exercise. Draw the resulting heap.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
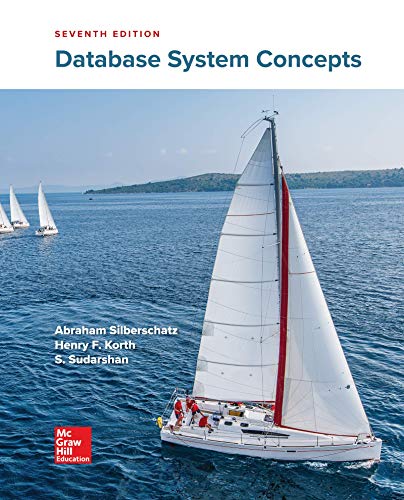
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
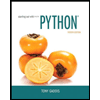
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
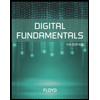
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
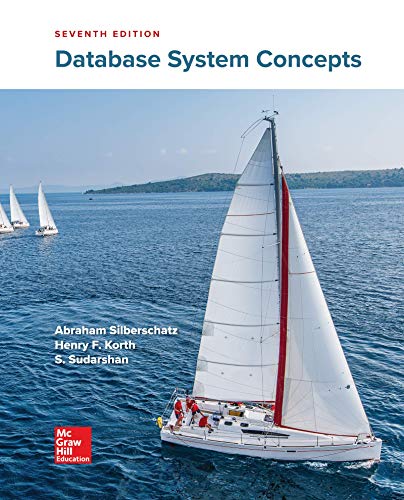
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
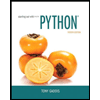
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
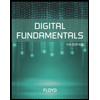
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
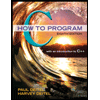
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
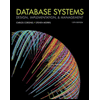
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
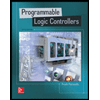
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education