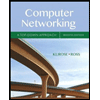
Write a program that inserts the following numbers into an initially empty BST such that the tree
produced is equivalent to binary search tree.
50 30 25 75 82 28 63 70 4 43 74
Hint: Calculate the mean value in method and put that value at the root and recursively build the
left and right subtree.
A. Implement a function which, given a node in this binary search tree, prints out the
maximum depths of its left and right subtrees.
B. Implement a function to find the maximum value of all the nodes in the tree.
C. Implement a function that balance the tree using appropriate rotation type and return the
root value.
Note: You are not allowed to use any built-in Data Structure classes to implement above
scenario

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 14 images

- The purpose of this assignment is to practice (JAVA): Implement a binary search tree structure Demonstrate tree traversals Analyze and compare algorithms for efficiency using Big-O notation For this project, you will implement a binary search tree and use it to store a large text file (JAVA). Your program should read text from a file and insert each word in a binary search tree. Do not store duplicate text. Once the tree is populated, print the contents of the tree using an in-order traversal. Next, allow the user to enter words to search for. For each word entered, your program should report the number of elements inspected and whether or not the word was located. Next, allow the user to enter words to remove from the tree. After each removal, print the contents of the tree. Provide an analysis of your remove and search algorithms using Big-O notation. Be sure to provide justification for your claims. (Look at image attached for sample run)arrow_forwardRewrite the definition of the function searchNode of the class B-tree provided (bTree.h) by using a binary search. Write a C++ code to ask the user to enter a list of positive integers ending with -999, build a b- tree of order 5 using the positive integers, and display the tree contents. Also, ask the user to enter a number to search and display if the number is found in the tree. bTree.harrow_forwardWrite a code to implement binary search tree. Implement all the methods, find, insert, delete, minimum, max, find successor. Test out all the methods.arrow_forward
- Write a function f1 that takes the root of a binary tree as a parameter and returns the sum of the nodes which are in the left subtree of the root. You must not consider the value of the root in the final sum.arrow_forward4. Write a recursive algorithm in pseudocode that finds the lowest common ancestor (LCA) of two given nodes in a binary tree T. The lowest common ancestor is defined between two nodes p and q as the lowest node in T that has both p and q as descendants (where we allow a node to be a descendant of itself). If either p or q is null, the LCA is null. For this problem, Nodes have left, right, and parent references as well as a field called level which stores the level of the node in the tree. In the sample tree below, node 5 is on level 0, while nodes 4 and 6 are on level 1. Write your solution here. 5 <- level 0 4 6 < level 1 function lowest_common_ancestor (Node P, Node q)arrow_forwardhow to Traversing a Binary Search Tree. To demonstrate how this method works, implement a program that inserts a series of numbers into a BST.as given in figure.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
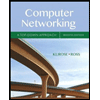
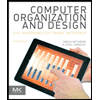
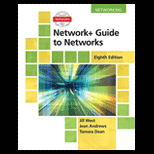
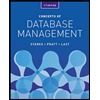
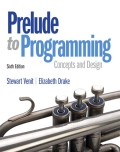
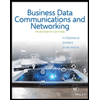