1. Write a function named "isBoundedArray" that accepts an array of integers and its size. The function will check the array to see whether the first and the last elements in the given array are the smallest and largest in the list, or the largest and smallest in the list. If they are, it will return true. Otherwise, it will return false. Requirement: please use only pointer notation in the function. No array notation.
1. Write a function named "isBoundedArray" that accepts an array of integers
and its size. The function will check the array to see whether the first and the last elements
in the given array are the smallest and largest in the list,
or the largest and smallest in the list.
If they are, it will return true. Otherwise, it will return false.
Requirement: please use only pointer notation in the function. No array notation.
For example, the following arrays will return true
int numList0[] = {10} ;
int numList1[] = {10, 20, 30, 40} ;
int numList2[] = {40, 30, 20, 10} ;
int numList3[] = {10, 30, 20, 40} ;
int numList4[] = {40, 20, 30, 10} ;
And these arrays will return false
int numList5[] = {10, 40, 20, 30} ;
int numList6[] = {40, 20, 30, 50} ;
2. Write a function named “isBinaryString” that accepts a C-string (an array of characters
with a NULL terminating character)and returns true
if that C-string contains a valid binary number and false otherwise.
A valid binary string is defined as a string
that must contain only '0' and '1' with the optional 'b' or 'B' at the end.
Please note that when 'b' or 'B' is at the end, it must precede with at least a '0' or '1'.
For example, "1010" or "10b" will return true
"" or "b" or "b1" will return false.
The requirement is that you cannot use any string function such as strlen or string class and its method.
This is an exercise on pointers and you are required to do the check
by examining each character in the C-string using pointers.
This function works with C-string and not string class.
It also means that you should not pass the size of the string to the function as a parameter.
Please use only pointer notation. Please do not use or refer the string class in this function.
The following C-string will return true
char s0[] = {'0', '\0'} ;
char s1[] = {'1', '0', '\0'} ;
char s2[] = {'1', 'b', '\0'} ;
char s3[] = {'1', '0', '1', '0', '\0'} ;
char s4[] = {'1', '0', '1', '0', 'b', '\0'} ;
And the following C-string will return false
char s5[] = {'\0'} ;
char s6[] = {'b', '\0'} ;
char s7[] = {'1', 'b', '0', '\0'} ;
char s8[] = {'1', '0', '1', '2', '\0'} ;

Step by step
Solved in 4 steps with 5 images

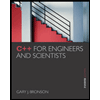
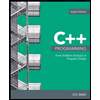
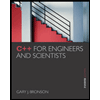
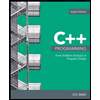