1). Write a java abstract class called Solid and you will derive two classes – Cylinder and Sphere – from Solid. The class Solid Member variables type whose data type is String. For example, the type could be sphere, cube, parallelepiped. 2). The methods should include: A default or null constructor A constructor that initializes the value of type Getters and Setters An abstract method to find volume whose signature is public abstract double findVolume(); An abstract method to find surface area whose signature is public abstract double findSurfaceArea() a toString method that returns a string like the following Solid Type: Sphere an equals method that returns true if two Solid objects have the same type and false otherwise. 3). The class Cylinder - Cylinder will extend Solid Member variables: Cylinder inherits type from Solid radius of type double height of type double Methods: a default or null constructor a constructor that initializes type, radius, and height. type will always be “Cylinder”. Remember to pass type to the super class implement the findVolume method. The volume of a cylinder is given by pr 2h where r = radius and h = height implement the findSurfaceArea method. The surface area of a cylinder is 2pr 2 + 2p In Java, p is given by Math.PI a toString method that creates a String like the following. Be sure to use super.toString!! Solid Type : Cylinder Radius : 10 Height 5.1 An equals method that returns true provided super.equals returns true and the two Cylinders have the same height and the two Cylinders have the same radius 4). The class Sphere – Sphere will also extend Solid Member variables radius of type double Sphere inherits type from Solid Method: a default constructor a constructor that initializes type and radius. type will always be “Sphere” Implement findVolume and findSurfaceArea. For a sphere volume = 4.0/3pr 3 surface area = 4pr 2 5). A Test Program: a). Write a method called menu with the signature public static int menu() b). The menu should print all of the choices and ask the user to enter a choice. c). Then menu should declare to read the integer representing the user's choice. This int should be returned to the user. do { choice = menu(); ,,, ,,, } while(choice !=5; e). In the test program, create an ArrayList of Solid. Write Java code that implements all of the options in the following menu Enter a sphere. Enter a cylinder. Print the toString for all Solids. Print volume and surface area for all solids in the list. QUIT
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
1). Write a java abstract class called Solid and you will derive two classes – Cylinder and Sphere – from Solid. The class Solid Member variables type whose data type is String. For example, the type could be sphere, cube, parallelepiped.
2). The methods should include:
- A default or null constructor
- A constructor that initializes the value of type
- Getters and Setters
- An abstract method to find volume whose signature is public abstract double findVolume();
- An abstract method to find surface area whose signature is public abstract double findSurfaceArea()
- a toString method that returns a string like the following Solid Type: Sphere
- an equals method that returns true if two Solid objects have the same type and false otherwise.
3). The class Cylinder - Cylinder will extend Solid Member variables:
- Cylinder inherits type from Solid
- radius of type double
- height of type double
Methods:
- a default or null constructor
- a constructor that initializes type, radius, and height. type will always be “Cylinder”. Remember to pass type to the super class
- implement the findVolume method. The volume of a cylinder is given by pr 2h where r = radius and h = height
- implement the findSurfaceArea method. The surface area of a cylinder is 2pr 2 + 2p In Java, p is given by Math.PI
- a toString method that creates a String like the following. Be sure to use super.toString!! Solid Type : Cylinder Radius : 10 Height 5.1
- An equals method that returns true provided
- super.equals returns true and
- the two Cylinders have the same height and
- the two Cylinders have the same radius
4). The class Sphere – Sphere will also extend Solid Member variables
- radius of type double
- Sphere inherits type from Solid
Method:
a default constructor
- a constructor that initializes type and radius. type will always be “Sphere”
- Implement findVolume and findSurfaceArea. For a sphere
- volume = 4.0/3pr 3
- surface area = 4pr 2
5). A Test Program:
a). Write a method called menu with the signature public static int menu()
b). The menu should print all of the choices and ask the user to enter a choice.
c). Then menu should declare to read the integer representing the user's choice. This int should be returned to the user.
do {
choice = menu();
,,,
,,,
} while(choice !=5;
e). In the test program, create an ArrayList of Solid. Write Java code that implements all of the options in the following menu
- Enter a sphere.
- Enter a cylinder.
- Print the toString for all Solids.
- Print volume and surface area for all solids in the list.
- QUIT
Unlock instant AI solutions
Tap the button
to generate a solution
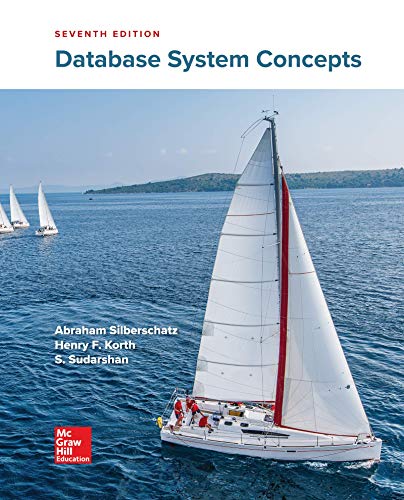
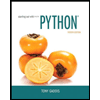
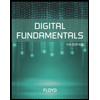
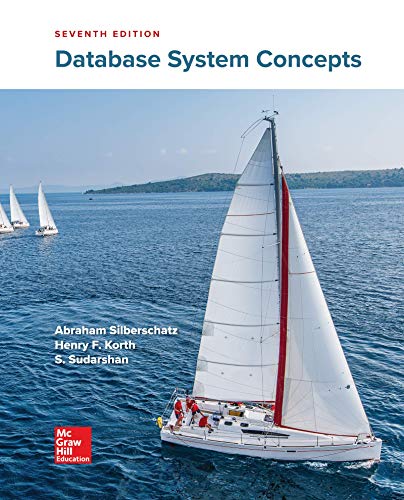
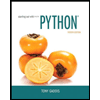
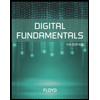
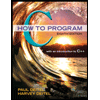
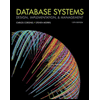
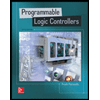