10.5 LAB: Count probations (EO) Students are put on probation if their GPA is below 2.0. Complete the Course class by implementing the countProbation() method, which returns the number of students with a GPA below 2.0. Given classes: Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here) Class Student represents a classroom student, which has three fields: first name, last name, and GPA. Hint: Refer to the Student class to explore the available methods that can be used for implementing the countProbation() method. Ex: If the following students and their GPA values are added to a course: Henry Cabot with 3.2 GPA Brenda Stern with 1.2 GPA Lynda Robison with 3.3 GPA Jake Flynn with 1.8 GPA then the countProbation() method returns 2 and the program output is: Probation count: 2 Course.java import java.util.ArrayList; public class Course { private ArrayList roster; // Collection of Student objects public Course() { roster = new ArrayList(); } public int countProbation() { /* Type your code here */ } public void addStudent(Student s) { roster.add(s); } // main public static void main(String args[]) { Course cis162 = new Course(); String first; // first name String last; // last name double gpa; // grade point average first = "Henry"; last = "Cabot"; gpa = 3.2; cis162.addStudent(new Student(first, last, gpa)); // Add 1st student first = "Brenda"; last = "Stern"; gpa = 1.2; cis162.addStudent(new Student(first, last, gpa)); // Add 2nd student first = "Lynda"; last = "Robison"; gpa = 3.3; cis162.addStudent(new Student(first, last, gpa)); // Add 3rd student first = "Jake"; last = "Flynn"; gpa = 1.8; cis162.addStudent(new Student(first, last, gpa)); // Add 4th student int prob = cis162.countProbation(); System.out.println("Probation count: " + prob); // Expect: 2 } } Student.java // Class representing a student public class Student { private String first; // first name private String last; // last name private double gpa; // grade point average // Student class constructor public Student(String first, String last, double gpa) { this.first = first; // first name this.last = last; // last name this.gpa = gpa; // grade point average } public double getGPA() { return gpa; } public String getLast() { return last; } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
10.5 LAB: Count probations (EO)
Students are put on probation if their GPA is below 2.0. Complete the Course class by implementing the countProbation() method, which returns the number of students with a GPA below 2.0.
Given classes:
- Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here)
- Class Student represents a classroom student, which has three fields: first name, last name, and GPA.
Hint: Refer to the Student class to explore the available methods that can be used for implementing the countProbation() method.
Ex: If the following students and their GPA values are added to a course:
Henry Cabot with 3.2 GPA
Brenda Stern with 1.2 GPA
Lynda Robison with 3.3 GPA
Jake Flynn with 1.8 GPA
then the countProbation() method returns 2 and the program output is:
Probation count: 2
Course.java
import java.util.ArrayList;
public class Course {
private ArrayList<Student> roster; // Collection of Student objects
public Course() {
roster = new ArrayList<Student>();
}
public int countProbation() {
/* Type your code here */
}
public void addStudent(Student s) {
roster.add(s);
}
// main
public static void main(String args[]) {
Course cis162 = new Course();
String first; // first name
String last; // last name
double gpa; // grade point average
first = "Henry";
last = "Cabot";
gpa = 3.2;
cis162.addStudent(new Student(first, last, gpa)); // Add 1st student
first = "Brenda";
last = "Stern";
gpa = 1.2;
cis162.addStudent(new Student(first, last, gpa)); // Add 2nd student
first = "Lynda";
last = "Robison";
gpa = 3.3;
cis162.addStudent(new Student(first, last, gpa)); // Add 3rd student
first = "Jake";
last = "Flynn";
gpa = 1.8;
cis162.addStudent(new Student(first, last, gpa)); // Add 4th student
int prob = cis162.countProbation();
System.out.println("Probation count: " + prob); // Expect: 2
}
}
Student.java
// Class representing a student
public class Student {
private String first; // first name
private String last; // last name
private double gpa; // grade point average
// Student class constructor
public Student(String first, String last, double gpa) {
this.first = first; // first name
this.last = last; // last name
this.gpa = gpa; // grade point average
}
public double getGPA() {
return gpa;
}
public String getLast() {
return last;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

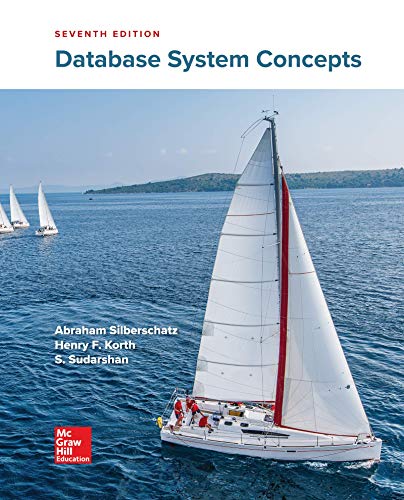
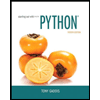
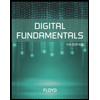
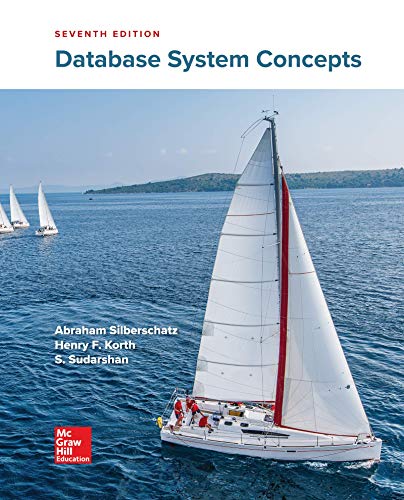
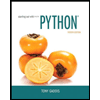
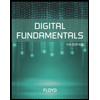
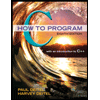
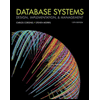
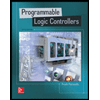