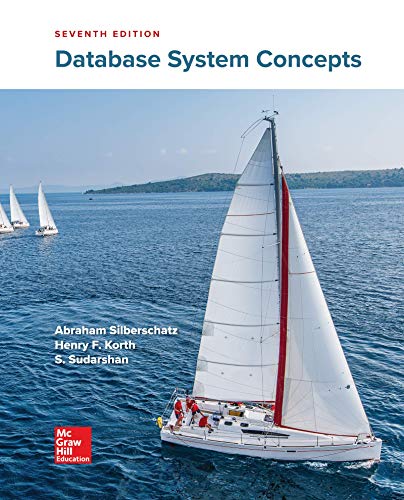
Concept explainers
Hello! Please help me with my Java hw
The three java files (source code) pasted below associated with this question.
QUESTION (using Eclipse and default Junit package that came with it):
Create a Junit 4 test case for the Hourly class. Your test should use these methods from class org.junit.Assert:
- assertEquals to test the getJob() method
- assertEquals to test the getPayRate() method
- assertTrue to test the hours attribute
Admin.java
package ch44extra;
import java.time.LocalDate;
public class Admin extends Person {
private String dept;
private String office;
private double salary;
//private double budget;removed to simplify class
public Admin(String name, LocalDate date, int id, String dept, String office, double salary) {
super(name, date, id);
this.dept = dept;
this.office = office;
this.salary = salary;
}
public Admin() {
super();
// TODO Auto-generated constructor stub
}
public String getDept() {
return dept;
}
public void setDept(String dept) {
this.dept = dept;
}
public String getOffice() {
return office;
}
public void setOffice(String office) {
this.office = office;
}
public double getSalary() {
return salary;
}
public void setSalary(double salary) {
this.salary = salary;
}
@Override
public void pay() {
double pay = salary / 12;
System.out.printf("Annual Salary pays $%,.2f monthly\n",pay);
}
@Override
public String toString() {
Strings = super.toString();
s += "\nAdmin dept=" + dept + ", office=" + office + ", salary=" + String.format("$%,.0f",salary);
returns;
}
}
Hourly.java
package ch44extra;
import java.time.LocalDate;
public class Hourly extends Person {
private double payRate;
private double hours;
private String job;
public Hourly(String name, LocalDate date, int id, double payRate, double hours, String job) {
super(name, date, id);
this.payRate = payRate;
this.hours = hours;
this.job = job;
}
public double getPayRate() {
return payRate;
}
public void setPayRate(double payRate) {
this.payRate = payRate;
}
public double getHours() {
return hours;
}
public void setHours(double hours) {
this.hours = hours;
}
public String getJob() {
return job;
}
public void setJob(String job) {
this.job = job;
}
@Override// implements abstract method pay inherited from Person
public void pay() {
double pay = payRate * hours;
System.out.printf("%.2f hours @ $%.2f = $%.2f weekly\n",hours,payRate,pay);
}
@Override
public String toString() {
return super.toString() + "\n" + "payRate=" + String.format("$%.2f",payRate) + ", hours=" + hours + ", job=" + job;
}
}
Person.java
package ch44extra;
import java.time.LocalDate;
// abstract because it contains the abstract method pay
public abstract class Person implements Comparable<Person> {
protected String name;
protected LocalDate date;
protected int id; // deleted salary field, added id
public Person(String name, LocalDate date, int id) {
super();
this.name = name;
this.date = date;
this.id = id;
}
public Person() {
}
// getters not needed since attributes are now protected
// abstract method, so Person class must be abstract
public abstract void pay(); // no braces, no body
@Override
public int compareTo(Person arg0) {
return date.compareTo(arg0.date);
}
@Override
public String toString() {
return "Name=" + name + ", hired=" + date + ", id number=" + id ;
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- In Java. The prompts/output and input examples are in the blue box in screenshot.arrow_forwardJava: According to this diagram, what are the methods that the StagBeetle class can use? (Select all that apply) Group of answer choices: A: hornSize B: fly() C: emitPheromone() D: eat()arrow_forwardCreate "People.java" Class for a "Person" with following functionality. People object should have following attributes/fields.. (make them private) their name as String, their address in next String and their phone number in 7 digit integer Put all this information in “People" object by doing the following Create a default Blank constructor - (Name should be "No Name", Address should be "000 Street, 000 City, North Pole, Earth", and Phone number should be 1234567) Create a full constructor Create accessors for all fields Create mutators for all fields (use proper this.) Create toString method for Peoplearrow_forward
- Hello! I am having trouble with my intro to JAVA course. I have basic understanding of it, I am failing to get the gets/sets and the DEMONSTRATION part of the assignment. TIA for the helparrow_forwardQuestion 1 Create a package named Lab6Q. Follow the parts outlined below: Part A: Add a public class called MyMethod in the Lab6Q package. Just like Math class, this will be your class that will have all your public-static methods, which you will call with the aid of the class name, as you do for Math method calls. Add and define the following public-static methods: • public static double myPow(double x, int y) – this method will accept a real number x as base and an integer number y as power, and return x'. Here instead of using the Math.pow() method you need to define this method (Hint: this method has been solved in the handout) • public static double mySin(double x) – this method will accept a real number (in radian value) and return the sin of that real number. Here instead of using Math.sin() method, you will define this method using the following numerical expression: N (-1)" :2 (2n + 1)! x(2n+1) sin x = n=0 Where, n and N are integer values an0! = 1 for n = 0, and n! = 1 x 2 x…arrow_forwardCreate a Java instance class named Rectangle that has two integer fields named length and width. Include two onstructors: a default constructor to initialize each of the fields to 1 and a second constructor that takes two rguments. Define a getter method for each of the fields.arrow_forward
- Hello! Need help with my Java Homework Please use Eclipse and Add a comments in each program to explain what your code is doing so I can understand it and learn. I really appreciate the help! Examine carefully the UML class diagram Attached: NOTE: Class Reptile was missing a toString() method. It has now been added. class Pet has an attribute of type java.util.Date. no specific dates are required for this attribute. compareTo(Dog) compares Dogs by weight. Create executable class TestPet as follows: create at least one Reptile pet and display it create an array of at least four Dog pets sort the array of Dogs by weight use a foreach loop to fully display all data for all dogs sorted by weight (see sample output) Sample Output Reptile name = Slinky, rock python, M Must be caged, crawls or slithers Not much sound, maybe a hiss, acquired Fri Feb 03 17:06:54 EST 2017 All dogs sorted by weight Dog name = Pedro, chihuahua, M Walks on a leash, weight 14 Barks or howls, acquired Fri…arrow_forwardI need this in Java please ASAP!arrow_forward19 We have been dealing with colors as tuples or lists. We could also create a Color class. This would allow us to create new color objects and pass them around. For each method below, write and implementation that matches the content of the method docstring. Recall that individual color channels must have a value between 0 and 255. class Color: """ This class provides a representation of a color """ definit_(self, red, green, blue): Initialize the color with red, green, and blue values 663939arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
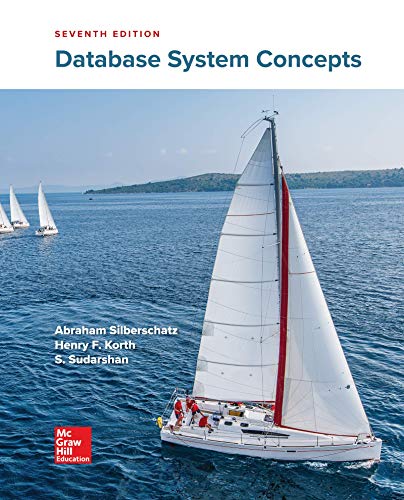
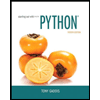
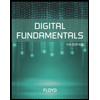
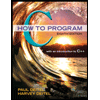
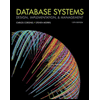
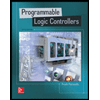