2) You must implement the pieces of the below classes on the following pages. You only should implement the functions that are underlined and followed by a "TODO: implement" comment. An example main function is provided to help you test your solution. You may assume the other functions have been implemented correctly, and you may use them. class Point { public: Point(); Point (double, double); double getX() const;
2) You must implement the pieces of the below classes on the following pages. You only should implement the functions that are underlined and followed by a "TODO: implement" comment. An example main function is provided to help you test your solution. You may assume the other functions have been implemented correctly, and you may use them. class Point { public: Point(); Point (double, double); double getX() const;
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter12: Points, Classes, Virtual Functions And Abstract Classes
Section: Chapter Questions
Problem 34SA
Related questions
Question
100%
C++
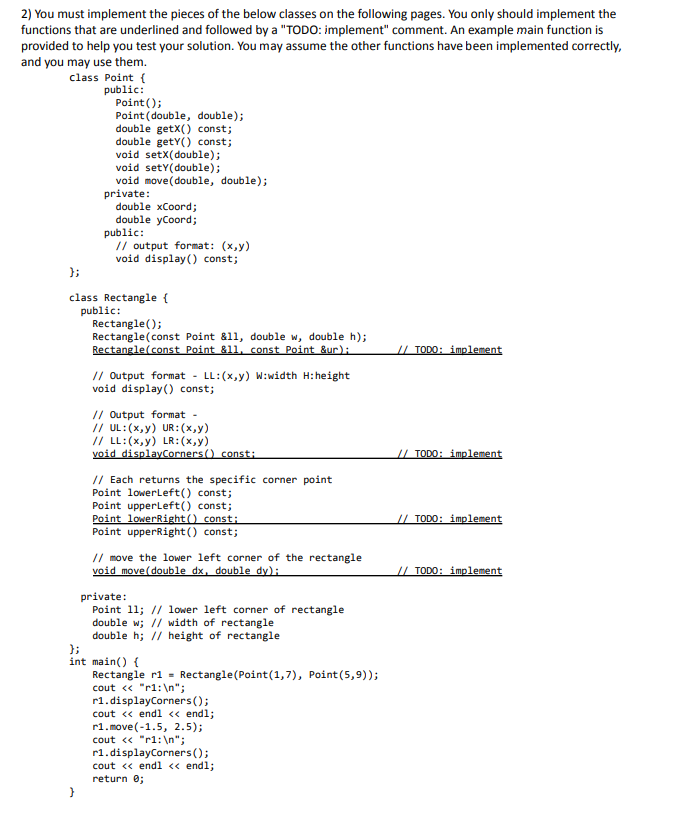
Transcribed Image Text:2) You must implement the pieces of the below classes on the following pages. You only should implement the
functions that are underlined and followed by a "TODO: implement" comment. An example main function is
provided to help you test your solution. You may assume the other functions have been implemented correctly,
and you may use them.
class Point {
public:
Point();
Point (double, double);
double getX() const;
double getY() const;
void setX(double);
void setY(double);
void move(double, double);
private:
double xCoord;
double yCoord;
public:
// output format: (x,y)
void display() const;
};
class Rectangle {
public:
Rectangle();
Rectangle(const Point &11, double w, double h);
Rectangle(const Point &11, const Point &ur):
// TODO: implement
// Output format - LL:(x,y) W:width H:height
void display() const;
// Output format -
// UL:(x,y) UR:(x,y)
// LL:(x,y) LR:(x,y)
void displayCorners() const:
/I TODO: implement
// Each returns the specific corner point
Point lowerLeft() const;
Point upperLeft() const;
Point lowerRight() const;
Point upperRight() const;
/ TODO: implement
// move the lower left corner of the rectangle
void move(double dx, double dy):
// TODO: implement
private:
Point 11; // lower left corner of rectangle
double w; // width of rectangle
double h; // height of rectangle
};
int main() {
Rectangle ri - Rectangle(Point(1,7), Point(5,9));
cout « "r1: \n";
r1.displayCorners();
cout « endl « endl;
r1.move(-1.5, 2.5);
cout « "r1:\n";
r1.displayCorners();
cout « endl « endl;
return 0;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
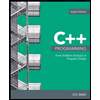
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
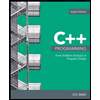
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning