I need help Implement the operators for class Login and class Person. Please help me out
I need help Implement the operators for class Login and class Person. Please help me out
Chapter9: Using Classes And Objects
Section: Chapter Questions
Problem 1CP: In previous chapters, you have created programs for the Greenville Idol competition. Now create a...
Related questions
Question
I need help Implement the operators for class Login and class Person.
Please help me out
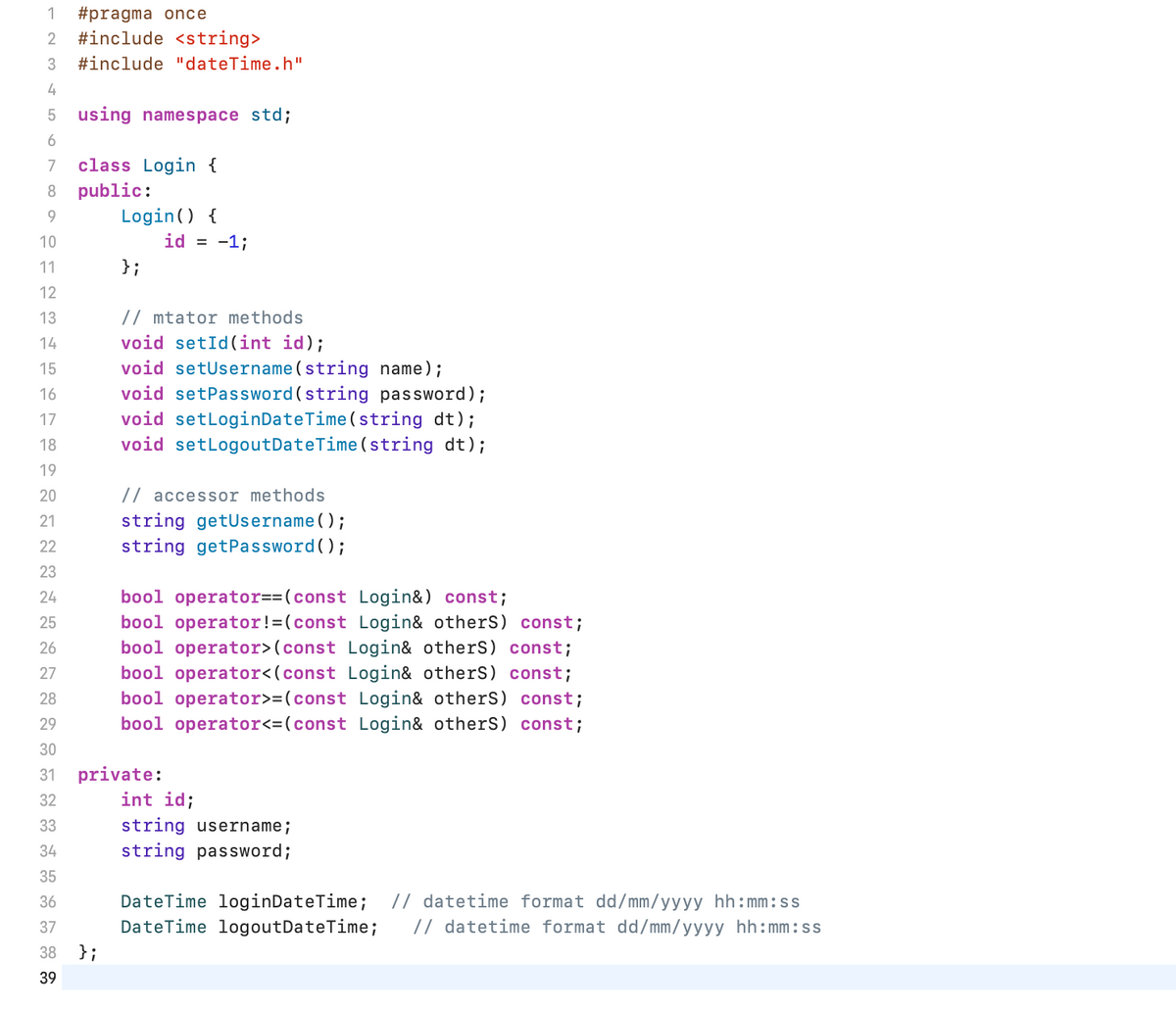
Transcribed Image Text:1 #pragma once
2 #include <string>
3 #include "dateTime.h"
4
5 using namespace std;
6
7
8 public:
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
class Login {
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
Login() {
};
};
id = -1;
// mtator methods
void setId(int id);
void setUsername (string name);
void setPassword (string password);
void setLoginDateTime (string dt);
void setLogoutDateTime (string dt);
// accessor methods
string getUsername();
string getPassword();
private:
bool operator==(const Login&) const;
bool operator!=(const Login& others) const;
bool operator>(const Login& others) const;
bool operator<(const Login& others) const;
bool operator>=(const Login& others) const;
bool operator<= (const Login& others) const;
int id;
string username;
string password;
DateTime loginDateTime; // datetime format dd/mm/yyyy hh:mm:ss
DateTime logoutDateTime; // datetime format dd/mm/yyyy hh:mm:ss
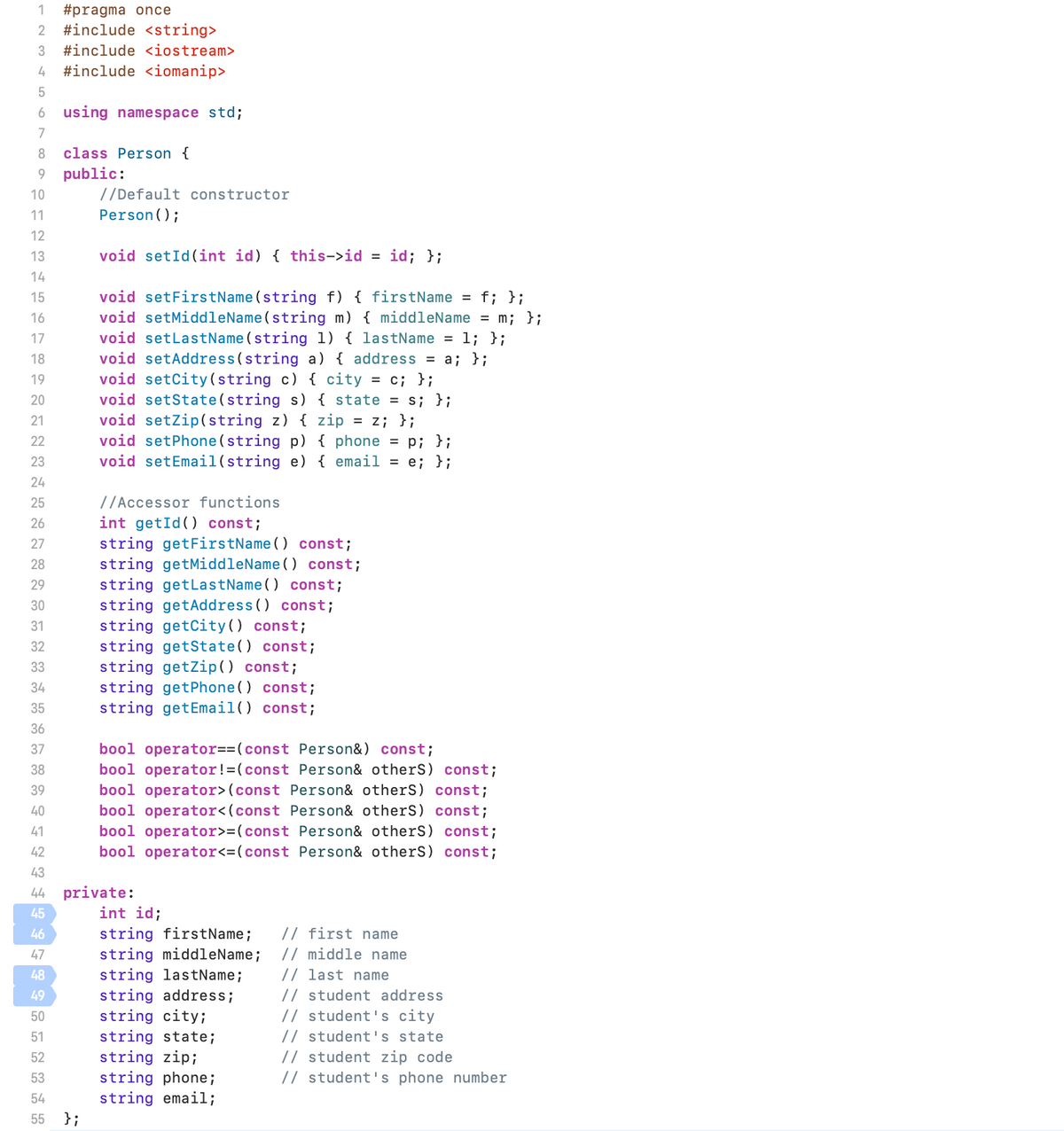
Transcribed Image Text:1 #pragma once
2 #include <string>
3 #include <iostream>
4 #include <iomanip>
5
6 using namespace std;
7
8 class Person {
9 public:
10
11
12
13
14
15
16
PFANN≈⇓⇓✿~✿252FF I F F F F F¶¶ÅNNA
17
18
19
20
21
23
24
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
45
46
47
44 private:
48
49
50
51
52
53
//Default constructor
Person();
54
55 };
void setId(int id) { this->id = id; };
void setFirstName(string f) { firstName = f; };
void setMiddleName(string m) { middleName = m; };
void setLastName (string 1) { lastName = 1; };
void setAddress (string a) { address = a; };
void setCity (string c) { city = c; };
void setState(string s) { state = s; };
void setZip(string z) { zip = z; };
void setPhone(string p) { phone = p; };
void setEmail (string e) { email = e; };
//Accessor functions
int getId() const;
string getFirstName() const;
string getMiddleName() const;
string getLastName() const;
string getAddress() const;
string getCity () const;
string getState() const;
string getZip() const;
string getPhone() const;
string getEmail() const;
bool operator==(const Person&) const;
bool operator !=(const Person& otherS) const;
bool operator> (const Person& otherS) const;
bool operator<(const Person& otherS) const;
bool operator>=(const Person& otherS) const;
bool operator<=(const Person& otherS) const;
int id;
string firstName; // first name
string middleName; // middle name
string lastName; // last name
string address; // student address
string city;
// student's city
string state;
// student's state
string zip;
// student zip code
string phone;
string email;
// student's phone number
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
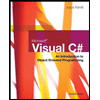
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
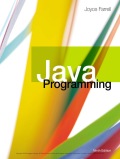
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
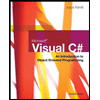
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
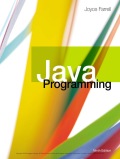
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT