2. Dog Years File: dog_years.py In Prac 3 you wrote a program to calculate a dog's age in dog years. Rewrite this program using a function for the conversion. Write a main function that repeatedly asks the user for an age in human years, then displays it in dog years until the user enters a negative number. Don't do any error checking on this age. Here's the calculation part already: if human_years <= 2: dog_years = human_years * 10.5 else: dog_years = 21 + 4 * (human_years - 2)
2. Dog Years
File: dog_years.py
In Prac 3 you wrote a program to calculate a dog's age in dog years.
Rewrite this program using a function for the conversion.
Write a main function that repeatedly asks the user for an age in human years, then displays it in dog years until the user enters a negative number.
Don't do any error checking on this age.
Here's the calculation part already:
if human_years <= 2:
dog_years = human_years * 10.5
else:
dog_years = 21 + 4 * (human_years - 2)
3. Seconds Display
File: seconds.py
In Prac 2 you wrote a program to calculate and display deep sleep time/percentage.
In that program, you displayed seconds as minutes and seconds, example:
Total sleep in seconds: 161
Deep sleep in seconds : 62
Deep sleep : 1m 2s
Total sleep: 2m 41s
Percentage : 38.50931677018634%
Note: the original program from prac 2 was about sleep, but this question is not related to that context.
The technique for figuring out minutes and seconds from just seconds is the same, so you can copy your work, but please change any references to the old context.
Write a simple program using a function that takes in a number of seconds and returns a string that can be used to display that value in minutes and seconds.
Notice that this function DOES NOT PRINT.
We want to use the string in different ways, so this function's job is simply to create/return a formatted string.
Write a main program that displays a bunch of different seconds values in minutes and seconds using a loop.
Example output:
Note: Think about how this was generated...
There's no user input; it starts at 0, goes to ? in steps of ?
Try and do the same in your program.
0 seconds is 0m 0s
635 seconds is 10m 35s
1270 seconds is 21m 10s
1905 seconds is 31m 45s
2540 seconds is 42m 20s
3175 seconds is 52m 55s
To help you understand SRP even more, let's think about what this function should return.
Should it return something like?
635 seconds is 10m 35s
Well, what if we wanted to use the same function for different kinds of tasks?
Let's do that now...
Add another small part to this same program - after the loop - that asks the user for their favourite duration in seconds, then prints it in minutes and seconds, like
Favourite duration in seconds: 639
You love 10m 39s
So... if our function returned something like 635 seconds is 10m 35s, then we could NOT use it for this task, even though it's really similar.
Do we need a second function that returns something like You love ...?
NO! That would be repeating ourselves, and we know... DRY.
So... we need to remember SRP.
This function has one job, and it's not printing or returning n seconds is ..., it's ONLY the bit that formats the seconds (argument) in minutes and seconds...
which we can now reuse in different situations!
SRP leads to function reusability and helping us not repeat ourselves

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

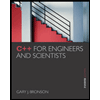
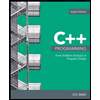
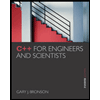
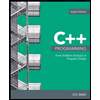