2. Functions Parameters Values Practice I by CodeChum Admin With No and Return Create a program that accepts an integer input, n. Call the hello() function for n times. An initial code is provided for you. Just fill in the blanks.
2. Functions Parameters Values Practice I by CodeChum Admin With No and Return Create a program that accepts an integer input, n. Call the hello() function for n times. An initial code is provided for you. Just fill in the blanks.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
I need help. In C language please.
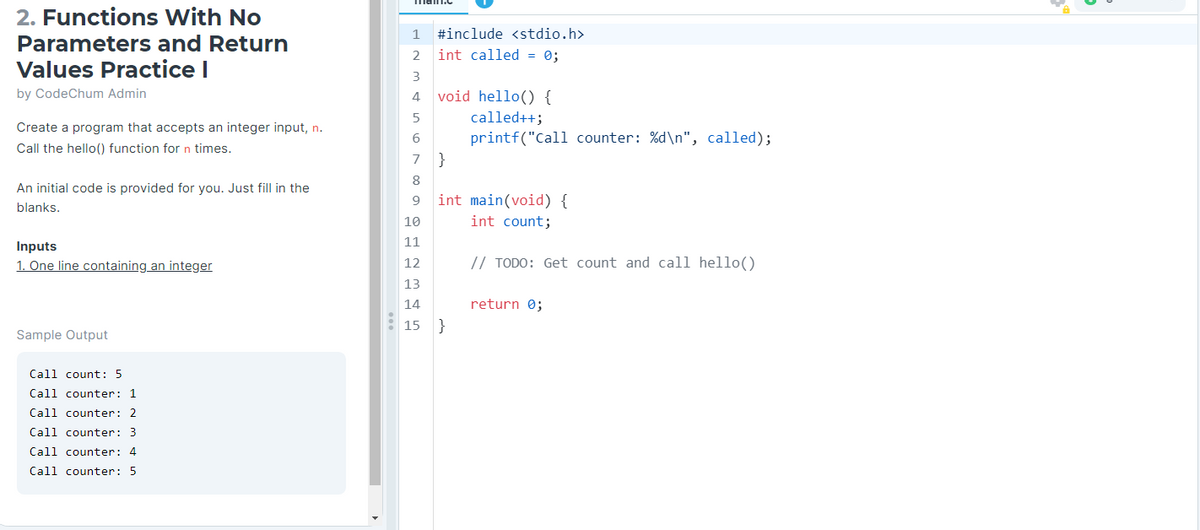
Transcribed Image Text:2. Functions With No
Parameters and Return
Values Practice I
by CodeChum Admin
Create a program that accepts an integer input, n.
Call the hello() function for n times.
An initial code is provided for you. Just fill in the
blanks.
Inputs
1. One line containing an integer
Sample Output
Call count: 5
Call counter: 1
Call counter: 2
Call counter: 3
Call counter: 4
Call counter: 5
4
1
2
#include <stdio.h>
int called = 0;
3
4 void hello() {
5
called++;
6
printf("Call counter: %d\n", called);
7 }
8
9
int main(void) {
int count;
10
11
12
13
14
15 }
// TODO: Get count and call hello()
return 0;
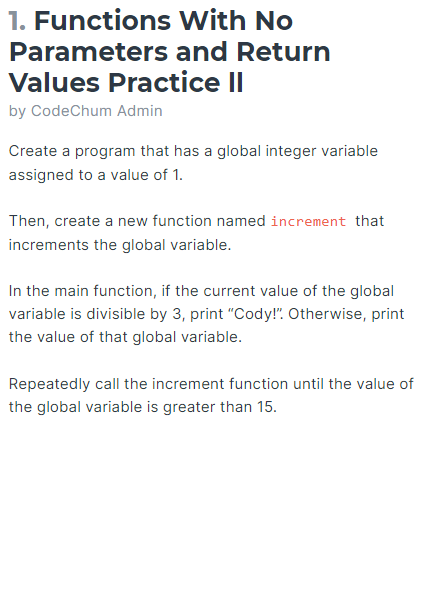
Transcribed Image Text:1. Functions
Parameters
With No
and Return
Values Practice II
by CodeChum Admin
Create a program that has a global integer variable
assigned to a value of 1.
Then, create a new function named increment that
increments the global variable.
In the main function, if the current value of the global
variable is divisible by 3, print "Cody!". Otherwise, print
the value of that global variable.
Repeatedly call the increment function until the value of
the global variable is greater than 15.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 4 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
In c language please
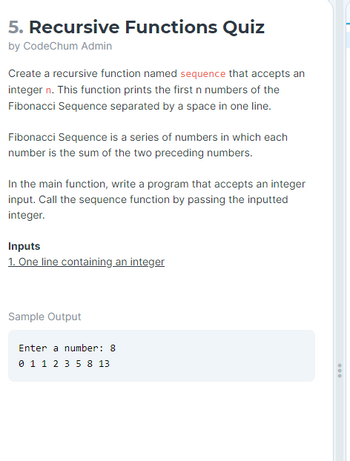
Transcribed Image Text:5. Recursive Functions Quiz
by CodeChum Admin
Create a recursive function named sequence that accepts an
integer n. This function prints the first n numbers of the
Fibonacci Sequence separated by a space in one line.
Fibonacci Sequence is a series of numbers in which each
number is the sum of the two preceding numbers.
In the main function, write a program that accepts an integer
input. Call the sequence function by passing the inputted
integer.
Inputs
1. One line containing an integer
Sample Output
Enter a number: 8
0 1 1 2 3 5 8 13
...
Solution
Follow-up Question
In C language please
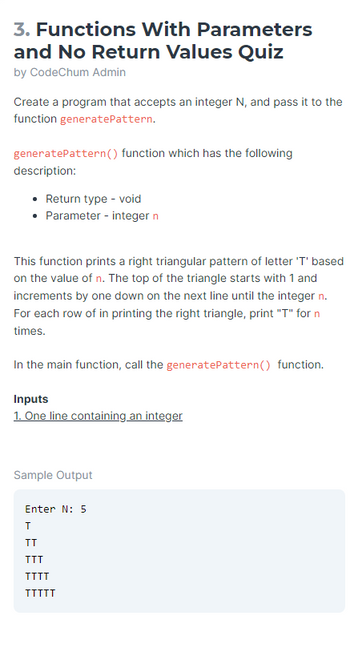
Transcribed Image Text:3. Functions With Parameters
and No Return Values Quiz
by CodeChum Admin
Create a program that accepts an integer N, and pass it to the
function generatePattern.
generatePattern () function which has the following
description:
• Return type - void
• Parameter - integer n
This function prints a right triangular pattern of letter 'T' based
on the value of n. The top of the triangle starts with 1 and
increments by one down on the next line until the integer n.
For each row of in printing the right triangle, print "T" for n
times.
In the main function, call the generate Pattern() function.
Inputs
1. One line containing an integer
Sample Output
Enter N: 5
T
TT
TTT
TTTT
TTTTT
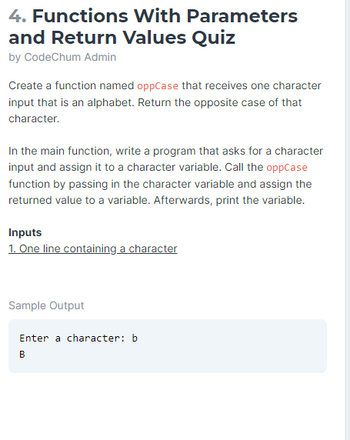
Transcribed Image Text:4. Functions With Parameters
and Return Values Quiz
by CodeChum Admin
Create a function named oppCase that receives one character
input that is an alphabet. Return the opposite case of that
character.
In the main function, write a program that asks for a character
input and assign it to a character variable. Call the oppCase
function by passing in the character variable and assign the
returned value to a variable. Afterwards, print the variable.
Inputs
1. One line containing a character
Sample Output
Enter a character: b
B
Solution
Follow-up Question
in C language pwease
![1. Functions with 2D Arrays
Practice I
by CodeChum Admin
Write a function named display Elements that takes a two-
dimensional array, the size of its rows and columns, then
prints every element of a two-dimensional array. Separate
every row by a new line and every column by a space.
In the main function, call the display Elements function and
pass in the required parameters.
Sample Output
164
728
59 3
...
main.c
1 #include <stdio.h>
2
3 int main(void) {
4
5
6
7
8
9
10
11 }
int array[][3]
{1, 6, 4},
{7, 2, 8},
{5, 9, 3}
};
return 0;
=
{](https://content.bartleby.com/qna-images/question/fe0536b9-ed76-4376-b062-0d17d510f0c1/432ef2ff-9da3-4de4-9cfd-87a66216e9e3/tuk6szo_thumbnail.png)
Transcribed Image Text:1. Functions with 2D Arrays
Practice I
by CodeChum Admin
Write a function named display Elements that takes a two-
dimensional array, the size of its rows and columns, then
prints every element of a two-dimensional array. Separate
every row by a new line and every column by a space.
In the main function, call the display Elements function and
pass in the required parameters.
Sample Output
164
728
59 3
...
main.c
1 #include <stdio.h>
2
3 int main(void) {
4
5
6
7
8
9
10
11 }
int array[][3]
{1, 6, 4},
{7, 2, 8},
{5, 9, 3}
};
return 0;
=
{
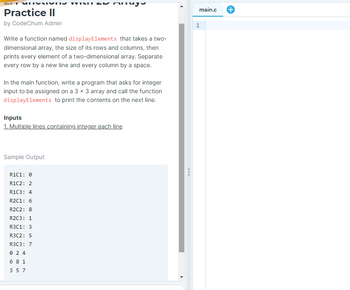
Transcribed Image Text:Practice II
by CodeChum Admin
Write a function named display Elements that takes a two-
dimensional array, the size of its rows and columns, then
prints every element of a two-dimensional array. Separate
every row by a new line and every column by a space.
VIXI
In the main function, write a program that asks for integer
input to be assigned on a 3 x 3 array and call the function
display Elements to print the contents on the next line.
Sample Output
Anay
Inputs
1. Multiple lines containing integer each line
R1C1: 0
R1C2: 2
R1C3: 4
R2C1: 6
R2C2: 8
R2C3: 1
R3C1: 3
R3C2: 5
R3C3: 7
024
681
3 5 7
main.c
1
+
Solution
Follow-up Question
in c language please
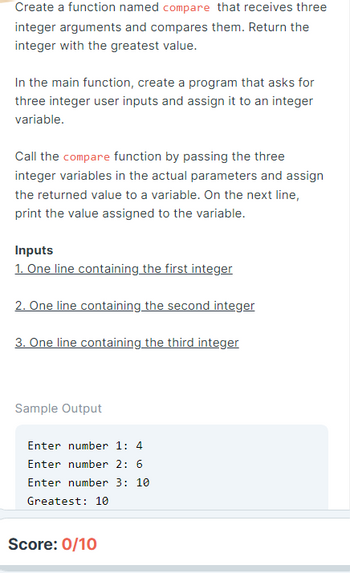
Transcribed Image Text:Create a function named compare that receives three
integer arguments and compares them. Return the
integer with the greatest value.
In the main function, create a program that asks for
three integer user inputs and assign it to an integer
variable.
Call the compare function by passing the three
integer variables in the actual parameters and assign
the returned value to a variable. On the next line,
print the value assigned to the variable.
Inputs
1. One line containing the first integer
2. One line containing the second integer
3. One line containing the third integer
Sample Output
Enter number 1: 4
Enter number 2: 6
Enter number 3: 10
Greatest: 10
Score: 0/10
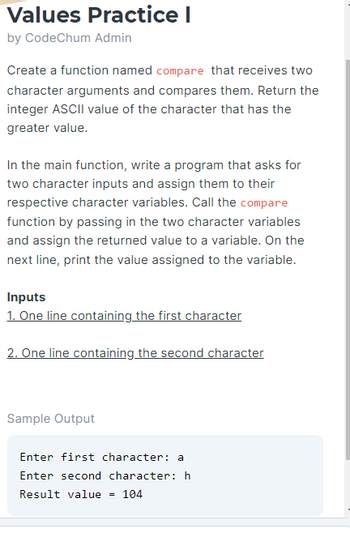
Transcribed Image Text:Values Practice I
by CodeChum Admin
Create a function named compare that receives two
character arguments and compares them. Return the
integer ASCII value of the character that has the
greater value.
In the main function, write a program that asks for
two character inputs and assign them to their
respective character variables. Call the compare
function by passing in the two character variables
and assign the returned value to a variable. On the
next line, print the value assigned to the variable.
Inputs
1. One line containing the first character
2. One line containing the second character
Sample Output
Enter first character: a
Enter second character: h
Result value = 104
Solution
Follow-up Question
in C language please
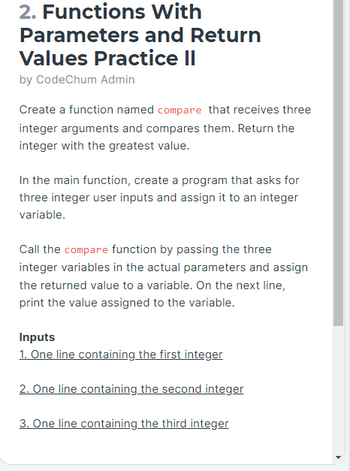
Transcribed Image Text:2. Functions
Parameters
Values Practice II
by CodeChum Admin
With
and Return
Create a function named compare that receives three
integer arguments and compares them. Return the
integer with the greatest value.
In the main function, create a program that asks for
three integer user inputs and assign it to an integer
variable.
Call the compare function by passing the three
integer variables in the actual parameters and assign
the returned value to a variable. On the next line,
print the value assigned to the variable.
Inputs
1. One line containing the first integer
2. One line containing the second integer
3. One line containing the third integer
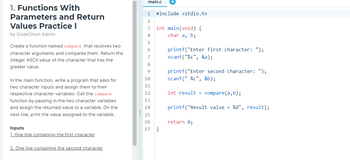
Transcribed Image Text:1. Functions With
Parameters and Return
Values Practice I
by CodeChum Admin
Create a function named compare that receives two
character arguments and compares them. Return the
integer ASCII value of the character that has the
greater value.
In the main function, write a program that asks for
two character inputs and assign them to their
respective character variables. Call the compare
function by passing in the two character variables
and assign the returned value to a variable. On the
next line, print the value assigned to the variable.
Inputs
1. One line containing the first character
2. One line containing the second character
main.c
1 #include <stdio.h>
2
3
4
5
6
8
9
10
11
12
13
14
15
16
17
int main(void) {
char a, b;
}
printf("Enter first character: ");
scanf("%c", &a);
printf("Enter second character: ");
scanf("%c", &b);
int result = compare(a,b);
printf("Result value = %d", result);
return 0;
Solution
Follow-up Question
C language
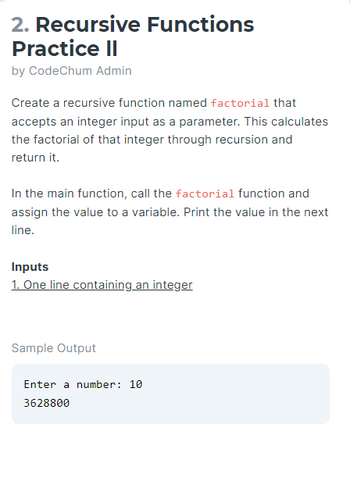
Transcribed Image Text:2. Recursive Functions
Practice II
by CodeChum Admin
Create a recursive function named factorial that
accepts an integer input as a parameter. This calculates
the factorial of that integer through recursion and
return it.
In the main function, call the factorial function and
assign the value to a variable. Print the value in the next
line.
Inputs
1. One line containing an integer
Sample Output
Enter a number: 10
3628800
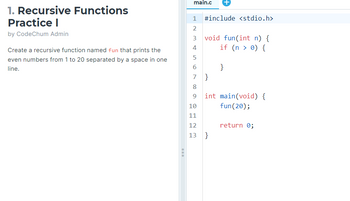
Transcribed Image Text:1. Recursive Functions
Practice I
by CodeChum Admin
Create a recursive function named fun that prints the
even numbers from 1 to 20 separated by a space in one
line.
main.c
1 #include <stdio.h>
2
3
4
5
6
7
8
9
10
11
void fun(int n) {
if (n > 0) {
}
}
int main(void) {
fun (20);
12
13 }
return 0;
Solution
Follow-up Question
in C language please. Thank you
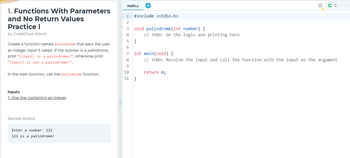
Transcribed Image Text:1. Functions With Parameters
and No Return Values
Practice I
by CodeChum Admin
Create a function named palindrome that asks the user
an integer input if called. If the number is a palindrome,
print "{input} is a palindrome!", otherwise print
"{input} is not a palindrome!".
In the main function, call the palindrome function.
Inputs
1. One line containing an integer
Sample Output
Enter a number: 121
121 is a palindrome!
main.c +
1 #include <stdio.h>
2
3
4
void palindrome (int number) {
5}
6
7
8
9
// TODO: Do the logic and printing here
int main(void) {
// TODO: Receive the input and call the function with the input as the argument
10
11 }
return 0;
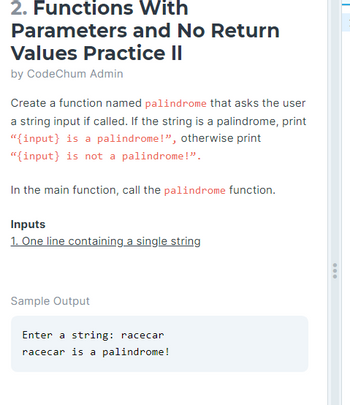
Transcribed Image Text:2. Functions
Parameters
Values Practice II
by CodeChum Admin
With
and No Return
Create a function named palindrome that asks the user
a string input if called. If the string is a palindrome, print
"{input) is a palindrome!", otherwise print
"{input} is not a palindrome!".
In the main function, call the palindrome function.
Inputs
1. One line containing a single string
Sample Output
Enter a string: racecar
racecar is a palindrome!
...
Solution
Recommended textbooks for you
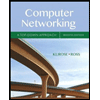
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
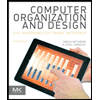
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
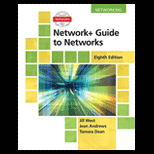
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
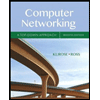
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
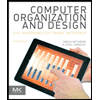
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
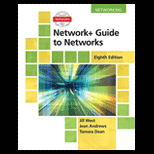
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
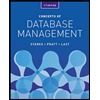
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
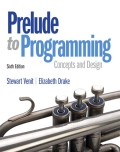
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
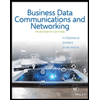
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY