3.1 Create an Employee objects project. Create an Employee class and use the provided UML diagram to code the instance fields, constructors and methods. 3.1.1 Both constructors must increment the static numberOfEmp field by one. Both constructors must call the setEmpCode() method to compile a code for the employee. Both constructors must call the setCommission() method. 3.1.2 setEmpCode() method: The empCode must be constructed in the following way: First character is the type of contract Next three characters a random 3 digits Add a backslash and the number of objects value to the code NOTE: You have to use the Random class in your code (See Ch 9). Do NOT use the Math.random() method. Example of code: S781/1 Explanation: Short term contracts (S), 781 is the 3-digit random value, first employee object. 3.1.3 setCommission() method: Assign a basic amount to the commission instance field according to the type of contract. If the contract is short term, the employee receives an amount of R1000. If the contract is long term, the employee receives an amount of R4000. 3.1.4 updateComm() method: All short term contract staff get 2.5% commission on sales, while long term contract staff gets 4% commission on sales. The method receives the sales amount, calculates the commission and add the commission to the current commission instance field's current value. 3.1.5 toString() method must compile a string to display the empCode and commission formatted in table format. EmpCode Commission S781/1 R 1000.00
UML specifications
-empCode:string
-typeContract:char
-commission:double
-numberofEmp:int
+Employee()
+Employee(tyleContract:char)
+getEmpCode:string
+getCommission():double
+getNumberOfEmp():int
+setEmpCode()
+setEmpContract():int
+setCommission()
+updateComm(amount:double)
+toString():string
3.1 Create an Employee objects project.
Create an Employee class and use the provided UML diagram to code the instance fields, constructors and methods.
3.1.1
- Both constructors must increment the static numberOfEmp field by one.
- Both constructors must call the setEmpCode() method to compile a code for the employee.
- Both constructors must call the setCommission() method.
3.1.2 setEmpCode() method:
- The empCode must be constructed in the following way:
- First character is the type of contract
- Next three characters a random 3 digits
- Add a backslash and the number of objects value to the code
- NOTE: You have to use the Random class in your code (See Ch 9). Do NOT use the Math.random() method.
- Example of code: S781/1
- Explanation: Short term contracts (S), 781 is the 3-digit random value, first employee object.
3.1.3 setCommission() method:
- Assign a basic amount to the commission instance field according to the type of contract.
- If the contract is short term, the employee receives an amount of R1000.
- If the contract is long term, the employee receives an amount of R4000.
3.1.4 updateComm() method:
- All short term contract staff get 2.5% commission on sales, while long term contract staff gets 4% commission on sales.
- The method receives the sales amount, calculates the commission and add the commission to the current commission instance field's current value.
3.1.5 toString() method must compile a string to display the empCode and commission formatted in table format.
EmpCode Commission
S781/1 R 1000.00
3.2 Create a testEmployees class. Do the following:
- Declare an array called arrEmp of 10 Employee type objects in the main method.
- Write a static method called fillArray() that receives the arrEmp array. Use a while loop to enter the information (type of contract) of a number of new employees. Quit input by entering the character X instead of the type of contract. For each employee create an object and save the object in the array. Return the array to the main method.
- Call the static fillArray() method from the main method.
- Write a static method called displayArray() to receive the array and display a numbered list of the information of the employees using a for-loop and the toString() method.
- Call the static displayArray() method from the main method.
- Write a static method called updateEmpCommission() to receive the array and update the commission of some of the employees as follows:
- Use a loop to update a number of employees' commission.
- Use a sentinel value -1 to control the loop.
- Display the list of employees.
- Ask the user to enter a number from the list. If the number is not -1, then enter a sales amount.
- Call the updateComm() method to update the commission of the employee.
- If the user enters -1 instead of a number from the list, the loop must be terminated.

Step by step
Solved in 4 steps with 1 images

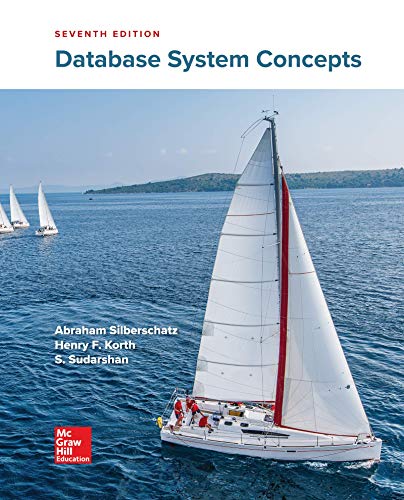
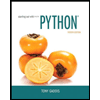
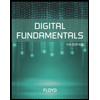
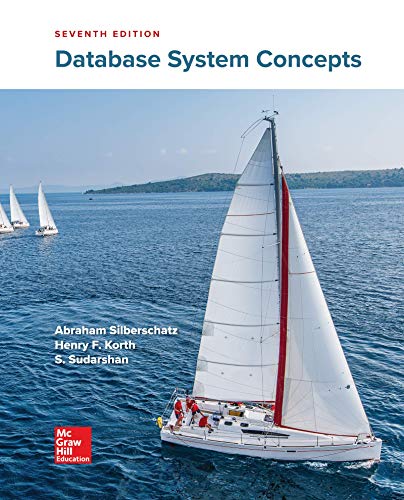
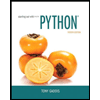
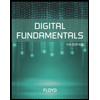
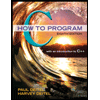
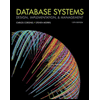
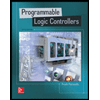