Java: Create UML (Unified Modeling Language) for the below code: fix the errors if needed: public class JukeBox { private CDPlayer cdPlayer; private SetcdCollection; private SongSelector ts; private User user; public JukeBox(CDPlayer cdPlayer, User user, Set cdCollection, SongSelector ts) { this.cdPlayer=cdPlayer; this.user=user; this.cdCollection=cdCollection; this.ts=ts; } public Song getCurrentSong() { return ts.getCurrentSong(); } public void setUser(User u) { this.user=u; } } // Like a real CD player, the CDP layer class supports storing just one CD at a time. The CDs that are not in play are stored in the jukebox. class CDPlayer { private Playlist p; private CD c; private Song s; /* Constructors. */ public CDPlayer(CD c, Playlist p, Song S) { this.c=c; this.p=p; this.s=s; } public CDPlayer(Playlist p) { this.p=p; } public CDPlayer(CD c) { this.c=c; } /* Playsong */ public playSong getPlaySong() { return playSong; } public void playSong(Song s) { this.s=s; // needs implementation } /* Getters and setters */ public Playlist getPlaylist() { return p; } public void setPlaylist(Playlist p) { this.p=p; } public CD getCD() { return c; } public void setCD(CD c) { this.c=c; } } // public class Playlist { private Song song; private Queuesongs; public Playlist(Song song, Queue queue) { this.songs=queue; } public Song getNextSongToPlay() { return queue.peek(); } public void queueUpSong(Song song) { queue.add(song); } public void removeSong(Song song) { queue.remove(song); } } class CD { /* data for id, artist, songs*/ private int id; private String artist; private String songs; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getArtist() { return artist; } public void setArtist(String artist) { this.artist = artist; } public String getSongs() { return songs; } public void setSongs(String song) { this.songs = songs; } // play the music public void play() { } } class Song { /* data for id, CD (could be null), title, length, etc */ private int id; private String title; private String album; public int getId() { return id; } public void setId(int id) { this.id = id; } public String getTitle() { return title; } public void setTitle(String title) { this.title = title; } public String getAlbum() { return album; } public void setAlbum(String album) { this.album = album; } // play the music public void play() { } } public class User { private String name; private long ID; public User(String name, long iD) { this.name=name; this.ID=iD; } public String getName() { return name; } public void setName(String name) { this.name=name; } public long getID() { return ID; } public void setID(long iD) { ID=iD; } public User getUser() { return this; } public static User addUser(String name, long iD) { return new User(name, iD); }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Java: Create UML (Unified Modeling Language) for the below code:
fix the errors if needed:
public class JukeBox {
private CDPlayer cdPlayer;
private Set<CD>cdCollection;
private SongSelector ts;
private User user;
public JukeBox(CDPlayer cdPlayer, User user, Set<CD> cdCollection, SongSelector ts) {
this.cdPlayer=cdPlayer;
this.user=user;
this.cdCollection=cdCollection;
this.ts=ts;
}
public Song getCurrentSong() {
return ts.getCurrentSong();
}
public void setUser(User u) {
this.user=u;
}
}
// Like a real CD player, the CDP layer class supports storing just one CD at a time. The CDs that are not in play are stored in the jukebox.
class CDPlayer {
private Playlist p;
private CD c;
private Song s;
/* Constructors. */
public CDPlayer(CD c, Playlist p, Song S) {
this.c=c;
this.p=p;
this.s=s;
}
public CDPlayer(Playlist p) {
this.p=p;
}
public CDPlayer(CD c) {
this.c=c;
}
/* Playsong */
public playSong getPlaySong() {
return playSong;
}
public void playSong(Song s) {
this.s=s;
// needs implementation
}
/* Getters and setters */
public Playlist getPlaylist() {
return p;
}
public void setPlaylist(Playlist p) {
this.p=p;
}
public CD getCD() {
return c;
}
public void setCD(CD c) {
this.c=c;
}
}
//
public class Playlist {
private Song song;
private Queue<Song>songs;
public Playlist(Song song, Queue<Song> queue) {
this.songs=queue;
}
public Song getNextSongToPlay() {
return queue.peek();
}
public void queueUpSong(Song song) {
queue.add(song);
}
public void removeSong(Song song) {
queue.remove(song);
}
}
class CD {
/* data for id, artist, songs*/
private int id;
private String artist;
private String songs;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getArtist() {
return artist;
}
public void setArtist(String artist) {
this.artist = artist;
}
public String getSongs() {
return songs;
}
public void setSongs(String song) {
this.songs = songs;
}
// play the music
public void play() {
}
}
class Song {
/* data for id, CD (could be null), title, length, etc */
private int id;
private String title;
private String album;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAlbum() {
return album;
}
public void setAlbum(String album) {
this.album = album;
}
// play the music
public void play() {
}
}
public class User {
private String name;
private long ID;
public User(String name, long iD) {
this.name=name;
this.ID=iD;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name=name;
}
public long getID() {
return ID;
}
public void setID(long iD) {
ID=iD;
}
public User getUser() {
return this;
}
public static User addUser(String name, long iD) {
return new User(name, iD);
}
}

Step by step
Solved in 3 steps with 1 images

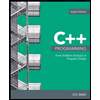
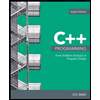