4. Complete the quick sort method by supplying code for the following methods: a. insertionSort (int A[]) (ref. line 21). This function is called to perform a simple sort on an array of integers when the problem size makes using quicksort inefficient. b. swapReferences ( int [ ] A, int i, int j ) (ref. lines 26, 28, 30, 33, 46 and 50). This function is called to perform a swap of two elements, at positions i and i, in an array A.
Can i get help with this missing code, java
1 class QuicksortTester
2 {
3
4 /**
5 * Quicksort
6 */
7
8 public void quicksort( int [ ] a )
9 {
10 quicksort( a, 0, a.length - 1 );
11 }
12
13 /**
14 * Internal quicksort method that makes recursive calls.
15 * Uses median-of-three partitioning and a cut-off.
16 */
17
public void quicksort( int [ ] a, int low, int high )
19 {
20 if( low + CUTOFF > high )
21 insertionSort( a );
22 else
23 { // Sort low, middle, high
24 int middle = ( low + high ) / 2;
25 if( a[ middle ].compareTo( a[ low ] ) < 0 )
26 swapReferences( a, low, middle );
27 if( a[ high ].compareTo( a[ low ] ) < 0 )
28 swapReferences( a, low, high );
29 if( a[ high ].compareTo( a[ middle ] ) < 0 )
30 swapReferences( a, middle, high );
31
32 // Place pivot at position high - 1
33 swapReferences( a, middle, high - 1 );
34 int pivot = a[ high - 1 ];
35
36 // Begin partitioning
37 int i, j;
38 for( i = low, j = high - 1; ; )
39 {
40 while( a[ ++i ].compareTo( pivot ) < 0 )
41 ;
42 while( pivot.compareTo( a[ --j ] ) < 0 )
43 ;
44 if( i >= j )
45 break;
46 swapReferences( a, i, j );
47 }
48
49 // Restore pivot
50 swapReferences( a, i, high - 1 );
51
52 quicksort( a, low, i - 1 ); // Sort small elements
53 quicksort( a, i + 1, high ); // Sort large elements
54 }
55 }
56
57
58 // insert code here for question 4
59
![4. Complete the quick sort method by supplying code for the following methods:
a. insertionSort(int A[]) (ref. line 21). This function is called to perform
a simple sort on an array of integers when the problem size makes using
quicksort inefficient.
b. swapReferences ( int [ ] A, int i, int j ) (ref. lines 26, 28, 30,
33, 46 and 50). This function is called to perform a swap of two elements, at
positions i and j, in an array A.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F13f42eda-4233-4463-985e-07142dfa1519%2F77f7ab53-5031-449f-a0c1-3ad2a77c3b2c%2Fv6mw8dw_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

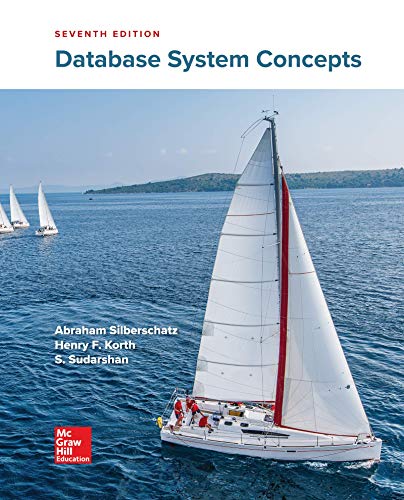
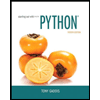
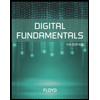
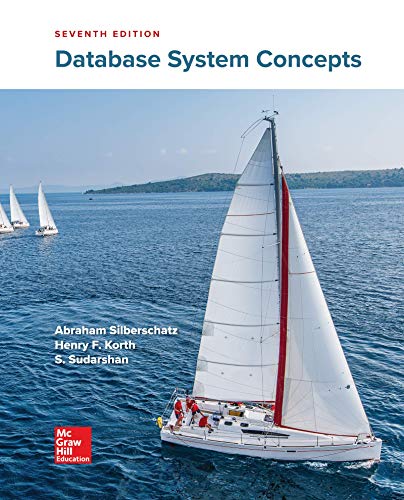
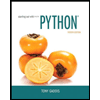
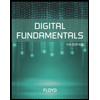
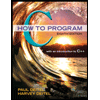
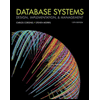
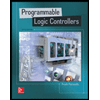