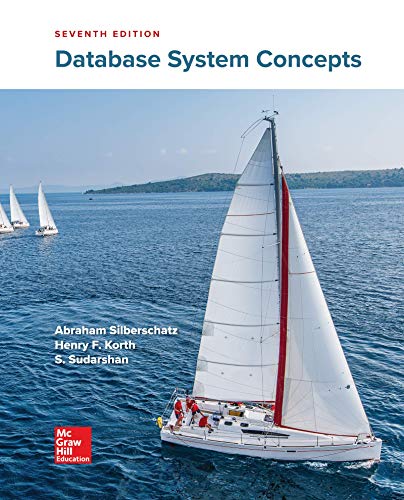
create an array of 30 random numbers that range between 1
and 100. And yet again, write a function that will receive a number from the user
and determine if that number exists in the array or not. But this time, start by
SORTING your input list. After a sort, the list in problem 1 is as follows:
[2, 2, 3, 5, 12, 14, 14, 15, 23, 36, 39, 41, 44, 44, 45, 48,
49, 50, 52, 52, 59, 71, 81, 82, 88, 89, 89, 93, 96, 97]
Approach:
Implement a method called findC(x, A, i, j), where x is the number we are
looking for in array A, the first index of the array is i and the last index is j. We want
to determine whether x exists in A anywhere between index i and index j. Your first
call to this method will therefore look like this: findC(x, A, 0, A.length-1).
In the body of your function, compare x with the item that is in the middle of the
array, as you did before. As before, call the middle of index of the array mid. But this
time, if x<=a[mid], recursively call your function to search ONLY the first half of the
array, i.e. between index i and mid. If x>a[mid], recursively call your function to
search ONLY the second half of the array, i.e. from mid+1 to j.
If you call findC(x, A, i, j) when i is equal to j, note that there is only one
element in the range. In this case, compare x with a[i] (or a[j]). If they are equal,
return true, otherwise return false. If you call the function when j<i, that also means
x does not exist in A and you should return false.
Writing any explicit loop in your code results a 0 for this question. Remember to provide
pre- and post- conditions. How many recursive calls will you need to search the entire
list? Do you think this implementation will run more quickly than your other
implementations? Why or why not?
Now copy the trace table below in your Word file and trace your function
findC(x,A,i,j) for when A, x, i, and j are initially [1, 6, 10, 14, 77, 82, 100],
77, 0, and 7, respectively. As a guideline, we have populated the tables with the
values for the first 2 calls and the corresponding returned values for each of thosecalls.
Call# | X | A | Value Returened to this Call |
1 | 77 | [1, 6, 10, 14, 77, 82, 100] | TRUE |
2 | 77 | [77, 82, 100] | TRUE |
to generate a solution
a solution
- Now, write another function that prints the values of any array in reverse order. (The function should not change the original array). As an example, if a [] = {1, 2, 3, 4, 5}; The function would print the values in reverse as: 5, 4, 3, 2, 1arrow_forwardThe function interleave_lists in python takes two parameters, L1 and L2, both lists. Notice that the lists may have different lengths. The function accumulates a new list by appending alternating items from L1 and L2 until one list has been exhausted. The remaining items from the other list are then appended to the end of the new list, and the new list is returned. For example, if L1 = ["hop", "skip", "jump", "rest"] and L2 = ["up", "down"], then the function would return the list: ["hop", "up", "skip", "down", "jump", "rest"]. HINT: Python has a built-in function min() which is helpful here. Initialize accumulator variable newlist to be an empty list Set min_length = min(len(L1), len(L2)), the smaller of the two list lengths Use a for loop to iterate k over range(min_length) to do the first part of this function's work. On each iteration, append to newlist the item from index k in L1, and then append the item from index k in L2 (two appends on each iteration). AFTER the loop…arrow_forwardWrite a function that will accept a list of integers and return the sum of the contents of the list(……if the list contains 1,2,3 it will return 6…..)arrow_forward
- ///////1/////. Given an array of integers, write a PHP function to find the maximum element in the array. PHP Function Signature: phpCopy code function findMaxElement($arr) { // Your code here } Example: Input: [10, 4, 56, 32, 7] Output: 56 You can now implement the findMaxElement function to solve this problem. Let me know answer. //. .arrow_forwardTo determine if two lists of integers are equivalent, you must write a function that accepts the lists as input and returns true or false (i.e. contain the same values). The greatest value of each list must be shown if the lists are dissimilar and the procedure is effective.arrow_forwardIn python We have a list (named data) of numbers sorted in ascending order, for example: data = [2, 4, 5, 9, 10, 12, 13, 17, 18, 20] Write a function (named DataVar(data)), which take a list (data) as input parameter and performs the following steps on the list: 1) it finds the mean m of the data (for the given example, it is m = (2 + 4 + 5 + 9 + 10 + 12 + 13 + 17 + 18 + 20)/10 =110/10 = 11). 2) then, it finds the difference of data and m. Essentially, it finds for all data: Diff = data-m For the given example, Diff = [-9, -7, -6, -2, -1, 1, 2, 6, 7, 9]. 3) then, it finds the sum of Diff and 7. Essentially, it finds for all data: Sums = Diff+7 For the given example, Sums = [-2, 0, 1, 5, 6, 8, 9, 13, 14, 16]. 4) then, it finds the square of all the elements of Sums, such that Squares = square of the elements of Sums. For the given example, Squares = [4, 0, 1, 25, 36, 64, 81, 169, 196, 256]. 5) it finds the average of Squares and stores it in result, and returns it. For the given…arrow_forward
- I am very confused on how to answer this and any thing will help. Create a function that accepts a single list. Inside the function, have nested for loops (a for loop inside a for loop). The outer for loop iterates through every item in the list. The inner loop iterates through every subitem in item, and prints it. Test the function by passing it the list [ [0, 1, 2], [3, 4, 5], [6, 7, 8] ].arrow_forwardthis code should be in python: write a function that receives a list as its only parameter. Inside the function remove all the duplicate items from the list and returns a new list. For Example: If we call the function with [“dad”, “aunt”, “mom”, “sister”, “mom”] as its input, the returned value should be [“dad”, “aunt”, “mom”, “sister”]. The order of the items inside the returned list does not matter.arrow_forwardshould be in python: write a function that receives a list as its only parameter. Inside the function remove all the duplicate items from the list and returns a new list and the input should be given by user. For Example: If we call the function with [“dad”, “aunt”, “mom”, “sister”, “mom”] as its user input, the returned value should be [“dad”, “aunt”, “mom”, “sister”]. The order of the items inside the returned list does not matter.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
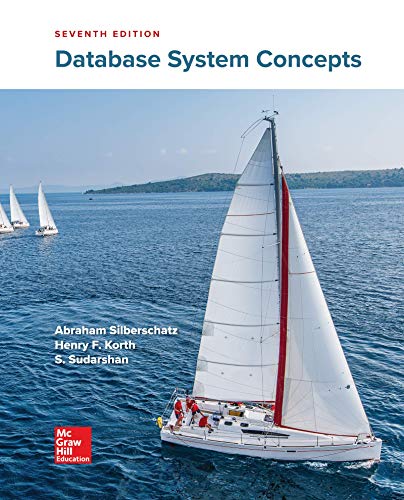
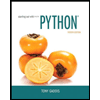
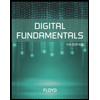
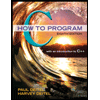
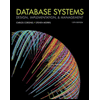
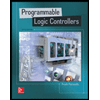