4.4 Increment/decrement, Arithmetic, Relational and Assigment Operators #include using namespace std; int main () { int a = 2, b = 3, c = 4.2, m, n = 2.4; float d = 2.2, e = 5.5, f; b = a = 5; a += b - n; c *= ++b + a / c--; f = d / e + c + 12 + b--; a = a + b + c + d + e + f; m = ++b + n-- % 7 + (a >= b || c > a); cout << "a = "<< a << endl; cout << "b = "<< b << endl; cout << "c = "<< c << endl; cout << "d = "<< d << endl; cout << "e = "<< e << endl; cout << "f = "<< f << endl; cout << "m = "<< m << endl; cout << "n = "<< n << endl; } 4.5 Increment/decrement, Arithmetic, Relational and Assigment Operators #include using namespace std; int main () { int a = 2, b = 3, c = 4.2, m, n = 2.4; float d = 2.2, e = 5.5, f; b = a = 5; cout << "b = "<< b << endl; a += b - n; c *= ++b + a / c--; cout << "c = "<< c << endl; f = d / e + c + 12 + b--; cout << "d = "<< d << endl; cout << "f = "<< f << endl; a = a + b + c + d + e + f; cout << "e = "<< e << endl; cout << "a = "<< a << endl; m = ++b + n-- % 7 + (a >= b || c > a); cout << "m = "<< m << endl; cout << "n = "<< n << endl<
4.4 Increment/decrement, Arithmetic, Relational and Assigment Operators
#include <iostream>
using namespace std;
int main ()
{
int a = 2, b = 3, c = 4.2, m, n = 2.4;
float d = 2.2, e = 5.5, f;
b = a = 5;
a += b - n;
c *= ++b + a / c--;
f = d / e + c + 12 + b--;
a = a + b + c + d + e + f;
m = ++b + n-- % 7 + (a >= b || c > a);
cout << "a = "<< a << endl;
cout << "b = "<< b << endl;
cout << "c = "<< c << endl;
cout << "d = "<< d << endl;
cout << "e = "<< e << endl;
cout << "f = "<< f << endl;
cout << "m = "<< m << endl;
cout << "n = "<< n << endl;
}
4.5 Increment/decrement, Arithmetic, Relational and Assigment Operators
#include <iostream>
using namespace std;
int main ()
{
int a = 2, b = 3, c = 4.2, m, n = 2.4;
float d = 2.2, e = 5.5, f;
b = a = 5;
cout << "b = "<< b << endl;
a += b - n;
c *= ++b + a / c--;
cout << "c = "<< c << endl;
f = d / e + c + 12 + b--;
cout << "d = "<< d << endl;
cout << "f = "<< f << endl;
a = a + b + c + d + e + f;
cout << "e = "<< e << endl;
cout << "a = "<< a << endl;
m = ++b + n-- % 7 + (a >= b || c > a);
cout << "m = "<< m << endl;
cout << "n = "<< n << endl<<endl;
cout << "a = "<< a << endl;
cout << "b = "<< b << endl;
cout << "c = "<< c << endl;
cout << "d = "<< d << endl;
cout << "e = "<< e << endl;
cout << "f = "<< f << endl;
cout << "m = "<< m << endl;
cout << "n = "<< n << endl;
}
Task
Explain why output program 4.4 and program 4.5 are different?.
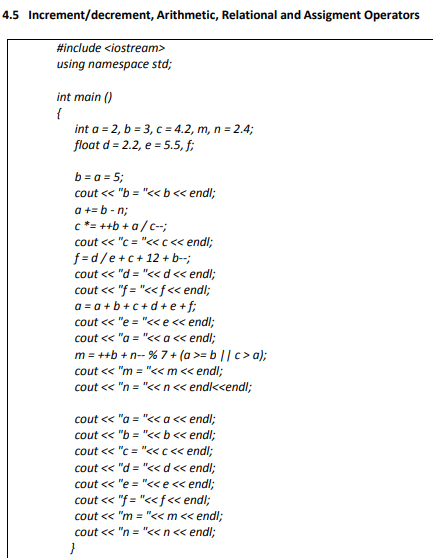
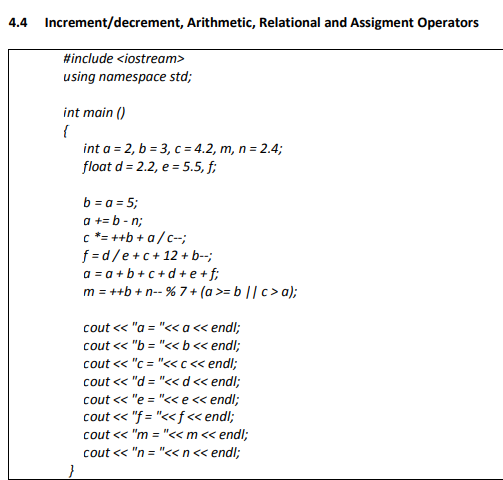

Step by step
Solved in 2 steps with 2 images

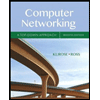
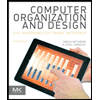
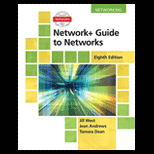
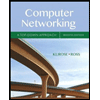
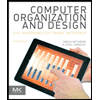
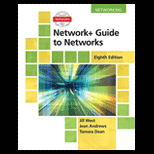
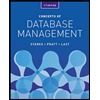
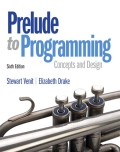
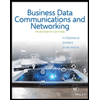