2. Printing binary Write a function void printBin(int value) that will print an integer as a binary number. Hint: You want to print a 1 or a O based on the high order bit, then you need to move the next to high order bit into the high order bit. You will need to explicitly count the number of bits you have printed. Write a main method that calls printBin multiple times with a few different values to test it. You should try at least a positive number, a negative number and O. Your submission should answer the following questions about this program: • There are at least two approaches to testing the high order bit. Describe one that you did NOT use in your code.
2. Printing binary Write a function void printBin(int value) that will print an integer as a binary number. Hint: You want to print a 1 or a O based on the high order bit, then you need to move the next to high order bit into the high order bit. You will need to explicitly count the number of bits you have printed. Write a main method that calls printBin multiple times with a few different values to test it. You should try at least a positive number, a negative number and O. Your submission should answer the following questions about this program: • There are at least two approaches to testing the high order bit. Describe one that you did NOT use in your code.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Need help with part 2
I was already helped with part one and they told me to repost to get help with part 2 so that's what I'm doing
All parts need to be included in the same C file so the new code needs to be added to the existing code
#include <stdio.h>
int main(){
unsigned int x = 1;
char *ccc = (char*)&x;
if (*ccc){
printf("This is Little endian");
}
else{
printf("This is Big endian");
}
getchar();
return 0;
}
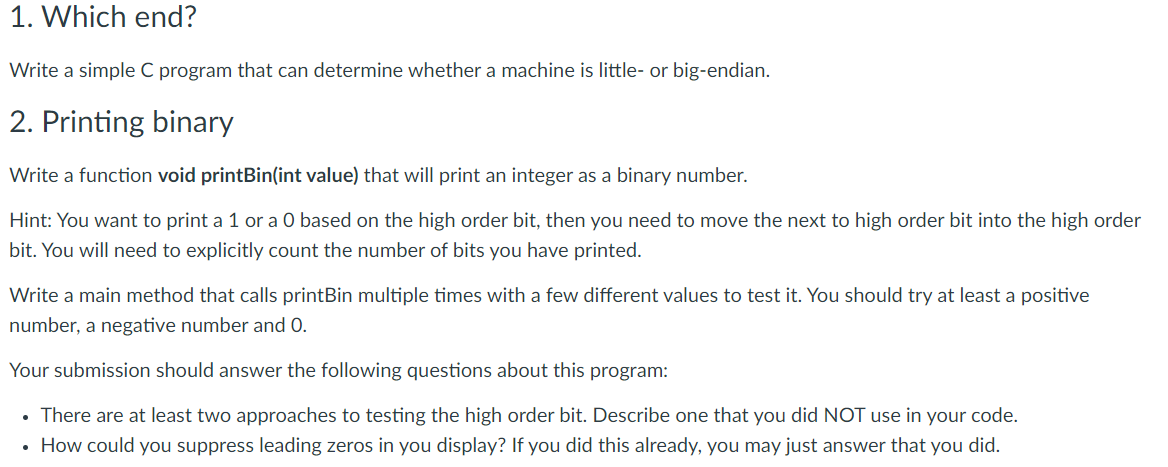
Transcribed Image Text:1. Which end?
Write a simple C program that can determine whether a machine is little- or big-endian.
2. Printing binary
Write a function void printBin(int value) that will print an integer as a binary number.
Hint: You want to print a 1 or a O based on the high order bit, then you need to move the next to high order bit into the high order
bit. You will need to explicitly count the number of bits you have printed.
Write a main method that calls printBin multiple times with a few different values to test it. You should try at least a positive
number, a negative number and 0.
Your submission should answer the following questions about this program:
There are at least two approaches to testing the high order bit. Describe one that you did NOT use in your code.
• How could you suppress leading zeros in you display? If you did this already, you may just answer that you did.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
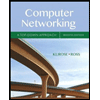
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
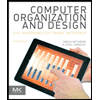
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
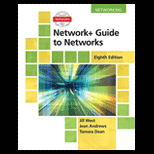
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
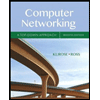
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
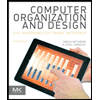
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
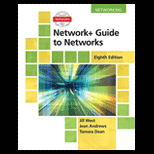
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
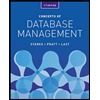
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
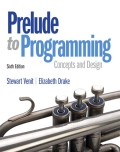
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
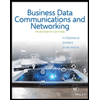
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY