6.15 LAB: JavaScript password strength Write a function called isStrongPassword() in script.js that has a single password parameter. The function should return true only if all the following conditions are true: • The password is at least 8 characters long. • The password does not contain the string "password". Hint: Use indexOf() to search for "password". • The password contains at least one uppercase character. Hint: Call the string method charCodeAt(index) to get the Unicode value of each character in the password. If a character code is between 65 and 90 (the Unicode values for A and Z), then an uppercase character is found. If any of the above conditions are false, isStrongPassword () should return false. Below are example calls to isStrongPassword(): // false - Too short // false - Contains "password" // false - No uppercase characters isstrongPassword ("Qwerty") ; isStrongPassword ("passwordQwerty") isstrongPassword ("qwerty123") isstrongPassword("Qwerty123") // true To test your code in your web browser, call isstrongPassword() from the JavaScript console.
6.15 LAB: JavaScript password strength Write a function called isStrongPassword() in script.js that has a single password parameter. The function should return true only if all the following conditions are true: • The password is at least 8 characters long. • The password does not contain the string "password". Hint: Use indexOf() to search for "password". • The password contains at least one uppercase character. Hint: Call the string method charCodeAt(index) to get the Unicode value of each character in the password. If a character code is between 65 and 90 (the Unicode values for A and Z), then an uppercase character is found. If any of the above conditions are false, isStrongPassword () should return false. Below are example calls to isStrongPassword(): // false - Too short // false - Contains "password" // false - No uppercase characters isstrongPassword ("Qwerty") ; isStrongPassword ("passwordQwerty") isstrongPassword ("qwerty123") isstrongPassword("Qwerty123") // true To test your code in your web browser, call isstrongPassword() from the JavaScript console.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
I am having trouble with javascript code. I just don't know where to start (I found most of the chapter confusing). I have included image of question and my javascript. Please explain as clear as possible (step by step) so that I can understand the process completely.
Once the code is written...I go to chrome (my web browser), bring up the console and call the function...can you explain these steps as well. Thank you
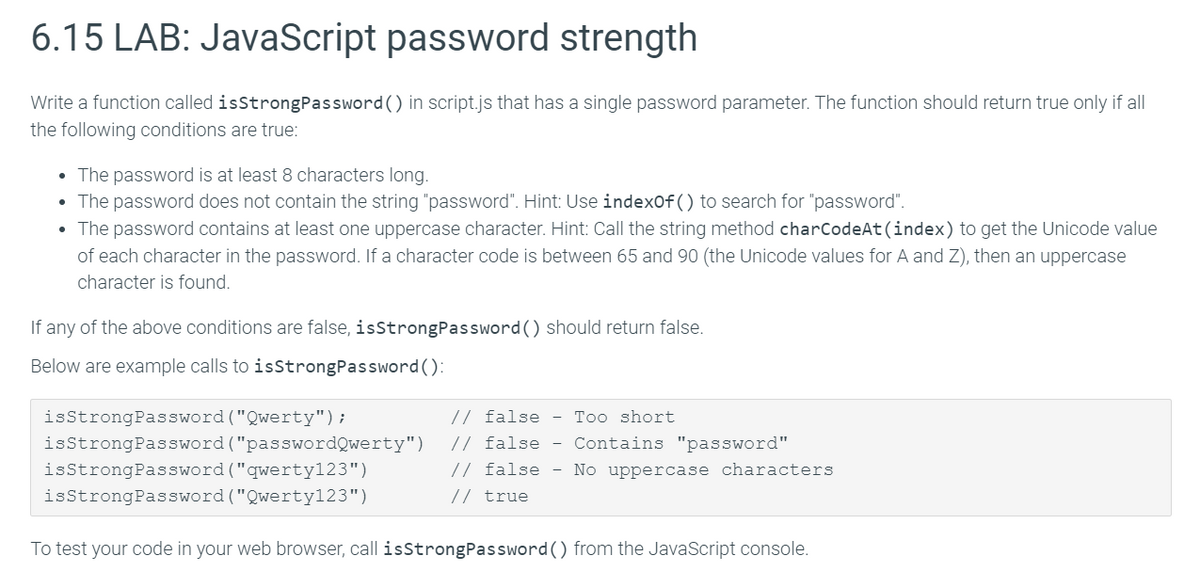
Transcribed Image Text:6.15 LAB: JavaScript password strength
Write a function called isStrongPassword() in script.js that has a single password parameter. The function should return true only if all
the following conditions are true:
• The password is at least 8 characters long.
• The password does not contain the string "password". Hint: Use indexof() to search for "password".
• The password contains at least one uppercase character. Hint: Call the string method charCodeAt(index) to get the Unicode value
of each character in the password. If a character code is between 65 and 90 (the Unicode values for A and Z), then an uppercase
character is found.
If any of the above conditions are false, isstrongPassword() should return false.
Below are example calls to isStrongPassword():
isstrongPassword ("Qwerty");
// false - Too short
// false - Contains "password"
// false - No uppercase characters
isStrongPassword ("passwordQwerty")
isstrongPassword ("qwerty123")
isStrongPassword ("Qwerty123")
// true
To test your code in your web browser, call isStrongPassword() from the JavaScript console.
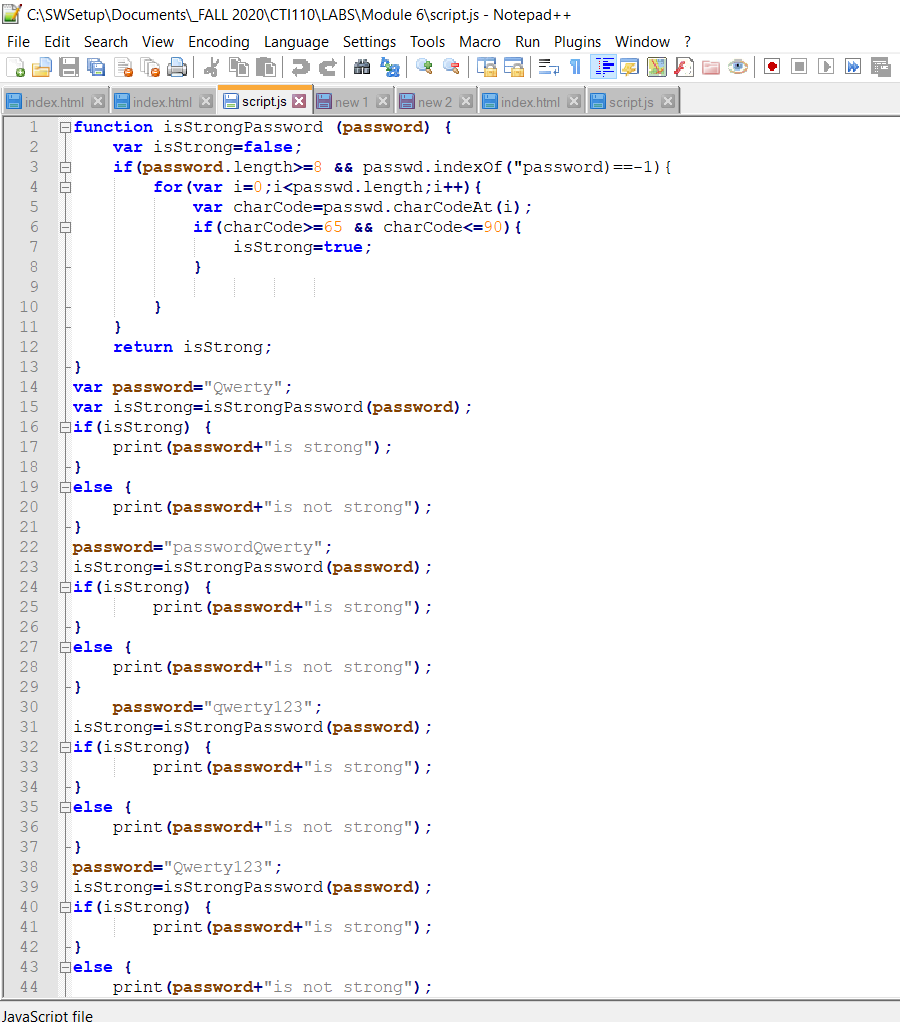
Transcribed Image Text:I C:\SWSetup\Documents\_FALL 2020\CTI110\LABS\Module 6\script.js - Notepad++
File Edit Search View Encoding Language Settings Tools Macro Run Plugins Window ?
O D
E index.html X B index.html X E scriptjs X E new 1 XE new 2 XE index.html X E scriptjs X
Efunction isstrongPassword (password) {
var isStrong=false;
if (password.length>=8 && passwd.indexof ("password) ==-1) {
1
for (var i=0;i<passwd.length;i++) {
var charcode=passwd.charCodeAt (i) ;
if (charCode>=65 && charCode<=90) {
isstrong=true;
}
4
7
8
10
}
11
}
return isStrong;
12
13
14
var password="Qwerty";
var isstrong=isStrongPassword (password) ;
Hif(isstrong) {
print (passwordt"is strong");
15
16
17
18
19
Helse {
20
print (password+"is not strong");
21
}
22
password="passwordQwerty";
isstrong=isstrongPassword (password) ;
Hif(isstrong) {
23
24
25
print (password+"is strong");
26
Belse {
print (password+"is not strong");
27
28
29
}
30
password="qwerty123";
isstrong=isstrongPassword (password) ;
Hif(isStrong) {
31
32
33
print (password+"is strong");
34
Eelse {
print (password+"is not strong");
}
35
36
37
38
password="Qwerty123";
isstrong=isStrongPassword (password) ;
Bif(isstrong) {
39
40
41
print (passwordt"is strong");
42
43
Helse {
44
print (password+"is not strong");
JayaScript file
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Recommended textbooks for you
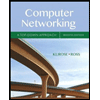
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
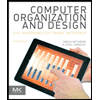
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
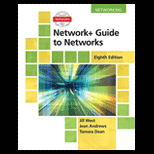
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
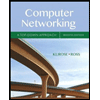
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
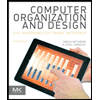
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
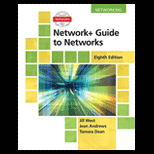
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
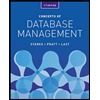
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
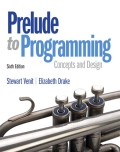
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
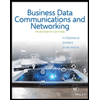
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY