7. Implement the fix_capitalization() function. fix_capitalization() has a string parameter and returns an updated string, where lowercase letters at the beginning of sentences are replaced with uppercase letters. fix_capitalization() also returns the number of letters that have been capitalized. Call fix_capitalization() in the execute_menu() function, and then output the number of letters capitalized followed by the edited string. Hint 1: Look up and use Python functions.islower() and .upper() to complete this task. Hint 2: Create an empty string and use string concatenation to make edits to the string. Example: Number of letters capitalized: 3 Edited text: We'll continue our quest in space. There will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians, more tea chers in space. Nothing ends here; our hopes and our journeys continue!
Python please
code I have so far:
def print_menu(usr_str: str):
aamenu_options = ['c', 'w', 'f', 'r', 's', 'q']
print('\nMENU')
print('c - Number of non-whitespace characters')
print('w - Number of words')
print('f - Fix capitalization')
print('r - Replace punctuation')
print('s - Shorten spaces')
print('q - Quit\n')
aachoice = input('Choose an option: ').lower()
while aachoice not in aamenu_options:
print('Invalid choice')
aachoice = input('Choose an option:')
if aachoice == 'c':
print('Number of non-whitespace characters:', get_num_of_non_WS_characters(usr_str))
elif aachoice == 'w':
print('Number of words:', get_num_of_words(usr_str))
elif aachoice == 'f':
usr_str, count = fix_capitalization(usr_str)
print('Number of letters capitalized:', usr_str)
print('Edited text:', count)
elif aachoice == 'r':
usr_str = replace_punctuation(usr_str)
print('Edited text:', usr_str)
elif aachoice == 's':
usr_str = shorten_space(usr_str)
print('Edited text:', usr_str)
return aachoice, usr_str
def get_num_of_non_WS_characters(usr_str:str):
count = 0
for i in usr_str:
if not i.isspace():
count +=1
return count
def get_num_of_words(usr_str: str):
num_words = len(usr_str.split())
return num_words
def fix_capitalization(usr_str):
count = 0
usr_str = list(usr_str)
if usr_str[0].islower():
usr_str[0] = usr_str[0].upper()
count += 1
for i in range(len(usr_str) - 3):
if usr_str[i] == '.' and usr_str[i + 3].islower():
usr_str[i + 3] = usr_str[i + 3].upper()
count += 1
return count, ''.join(usr_str)
def replace_punctuation(usr_str: str, **kwargs):
exclamation_count = 0
semicolon_count = 0
result = ''
for i in usr_str:
if i == '!':
i = '.'
exclamation_count += 1
elif i == ';':
i = ','
semicolon_count += 1
result += i
print('Punctuation replaced', exclamation_count)
print('Exclamation Count:', semicolon_count)
return result
def shorten_space(usr_str:str):
result = ''
for i in range(len(usr_str) - 1):
if not (usr_str[i].isspace() and usr_str[i + 1].isspace()):
result += usr_str[i]
return result
def main():
usr_str = input('Enter a sample text: \n')
print('\nYou entered:', usr_str)
choice = ''
while choice != 'q':
choice, usr_str = print_menu(usr_str)
if __name__ == '__main__':
main()
input given:
we'll continue our quest in space. there will be more shuttle flights and more shuttle crews and, yes; more volunteers, more civilians, more teachers in space. nothing ends here; our hopes and our journeys continue!
error:
my f/fix capitalization is not giving out the correct output.
mine is giving out 1 and only fixing the first letter, while the correct one should be three and fix three letters
first photo(with the green and blue text) is my output, and the second photo is the correct output

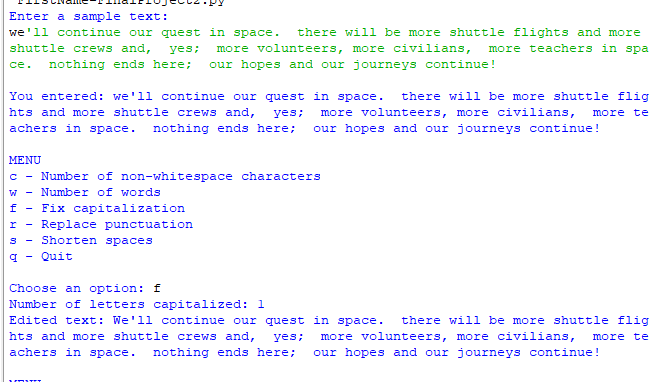

Step by step
Solved in 4 steps with 2 images

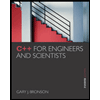
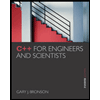