Sample run 1: Function Call int size = 5; string input_strings [size] = {"clefairy", "meowth", "snorlax"}; int num_elements = 3; int count = 1; string string_to_insert = "charizard"; string string_to_find = "meowth"; // updating num_elements with the updated value returned by secondPlace num_elements = secondPlace (input_strings, string_to_insert, string_to_find, num_elements, size, count); // print num_elements cout << "Function returned value: " << num_elements << endl; // print array contents for(int i = 0; i < size; i++) { } cout <
Sample run 1: Function Call int size = 5; string input_strings [size] = {"clefairy", "meowth", "snorlax"}; int num_elements = 3; int count = 1; string string_to_insert = "charizard"; string string_to_find = "meowth"; // updating num_elements with the updated value returned by secondPlace num_elements = secondPlace (input_strings, string_to_insert, string_to_find, num_elements, size, count); // print num_elements cout << "Function returned value: " << num_elements << endl; // print array contents for(int i = 0; i < size; i++) { } cout <
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 5SA
Related questions
Question
Write in C++
Now, what if Sam wants to insert Eevee after Pikachu but he doesn't know where Pikachu is located in his list. Let's help him search for a Pokemon by its name and insert another Pokemon directly after it. Write a function secondPlace() that takes six parameters and inserts a new Pokemon into a list right after another specified pokemon. Once you find the specified Pokemon, use the insertAfter() function from the previous sub-question to add the new Pokemon. The function should return the new number of strings in the array.
Note
- The same Pokemon may appear in the list multiple times (Sam has a bad memory). Assume that Sam wants every instance of the Pokemon he is searching for to be followed by the Pokemon he is attempting to insert.
- If the array is already full or if the number of strings that are going to be added to the array plus the current number of strings exceeds the total size of the array, the array should remain unchanged.
- Assume all inputs are lowercase alphabets.
Function specifications
- Name: secondPlace()
- Parameters (Your function should accept these parameters IN THIS ORDER):
- input_strings string: The array containing strings
- string_to_insert string: the string to insert
- string_to_find string: the string to find (target string)
- num_elements int: The number of elements that are currently stored in the array
- arr_size int: The number of elements that can be stored in the array
- count int: The number of the target strings present in the array
- Return Value: int:
- The number of elements in the array after the new strings are inserted into the array
- The original number of elements in the array if the new pokemon cannot be inserted into the array
![Sample run 1: Function Call
int size = 5;
string input_strings [size]
int num_elements = 3;
int count = 1;
string string_to_insert = "charizard";
string string_to_find = "meowth";
// updating num_elements with the updated value returned by secondPlace
num_elements = secondPlace (input_strings, string_to_insert, string_to_find, num_elements, size, count);
// print num_elements
cout << "Function returned value: " << num_elements << endl;
// print array contents
for(int i = 0; i < size; i++)
{
}
=
Output
{"clefairy", "meowth", "snorlax"};
cout <<input_strings[i] << endl;
Function returned value: 4
clefairy
meowth
charizard
snorlax
Q₁](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb1d14486-aab8-45fa-9185-3d15b7af8b4b%2F152adfc0-62ba-4b18-bdd8-43861fcce8bc%2Fjifm1en_processed.png&w=3840&q=75)
Transcribed Image Text:Sample run 1: Function Call
int size = 5;
string input_strings [size]
int num_elements = 3;
int count = 1;
string string_to_insert = "charizard";
string string_to_find = "meowth";
// updating num_elements with the updated value returned by secondPlace
num_elements = secondPlace (input_strings, string_to_insert, string_to_find, num_elements, size, count);
// print num_elements
cout << "Function returned value: " << num_elements << endl;
// print array contents
for(int i = 0; i < size; i++)
{
}
=
Output
{"clefairy", "meowth", "snorlax"};
cout <<input_strings[i] << endl;
Function returned value: 4
clefairy
meowth
charizard
snorlax
Q₁
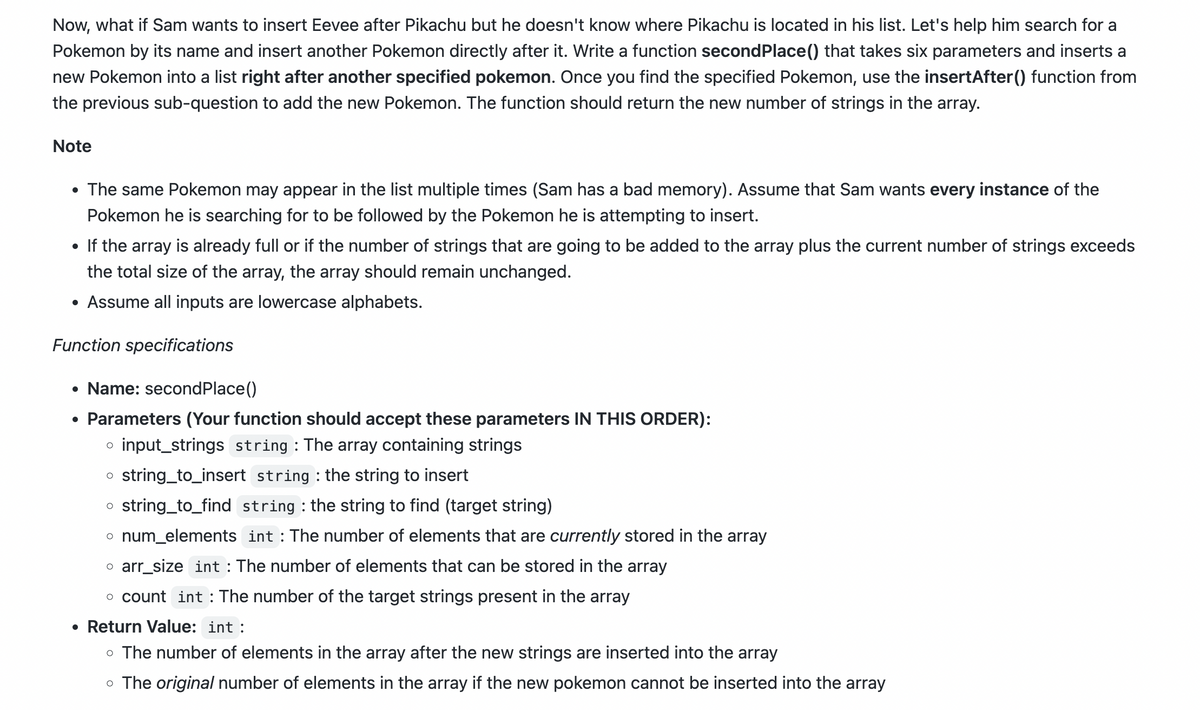
Transcribed Image Text:Now, what if Sam wants to insert Eevee after Pikachu but he doesn't know where Pikachu is located in his list. Let's help him search for a
Pokemon by its name and insert another Pokemon directly after it. Write a function secondPlace() that takes six parameters and inserts a
new Pokemon into a list right after another specified pokemon. Once you find the specified Pokemon, use the insertAfter() function from
the previous sub-question to add the new Pokemon. The function should return the new number of strings in the array.
Note
• The same Pokemon may appear in the list multiple times (Sam has a bad memory). Assume that Sam wants every instance of the
Pokemon he is searching for to be followed by the Pokemon he is attempting to insert.
• If the array is already full or if the number of strings that are going to be added to the array plus the current number of strings exceeds
the total size of the array, the array should remain unchanged.
• Assume all inputs are lowercase alphabets.
Function specifications
• Name: secondPlace()
• Parameters (Your function should accept these parameters IN THIS ORDER):
input_strings string: The array containing strings
string_to_insert string the string to insert
string_to_find string the string to find (target string)
o num_elements int: The number of elements that are currently stored in the array
o arr_size int: The number of elements that can be stored in the array
o count int: The number of the target strings present in the array
O
O
O
• Return Value: int :
• The number of elements in the array after the new strings are inserted into the array
• The original number of elements in the array if the new pokemon cannot be inserted into the array
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
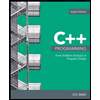
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
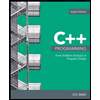
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning