A company pays its employees on a weekly basis. The employees are of four types: Salariedemployeesarepaidafixedweeklysalaryregardlessofthenumberofhoursworked, hourly employees are paid by the hour and receive overtime pay (i.e., 1.5 times their hourly salary rate) for all hours worked in excess of 40 hours, commission employees are paid a percentage of their sales and base-salaried commission employees receive a base salary plus a percentage of their sales. For the current pay period, the company has decided to reward salaried-commission employees by adding 10% to their base salaries. The company wants to write an application that performs its payroll calculations polymorphically. Create an EMPLOYEE class having First Name, Last Name, socialSecurityNumber(SSN); Provide a default Constructor. Provide overloaded parameterized Constructors. Provide getters, and setters for the data members. In addition there is method earnings which will return the earning of employees. For now you just simply prints a statement “calculate salary”. Class SalariedEmployee extends class Employee having weeklySalary as a member and overrides method earnings. The class includes a constructor that takes a first name, a last name, a social security number and a weekly salary as arguments; a set method to assign a new non negative value to instance variable weeklySalary; a get method to return weeklySalary; a method earnings to calculate a SalariedEmployee’s earnings; and a method toString, which returns a String including the employee’s type, name and SSN. Class HourlyEmployee also extends Employee and have hourlyWage and hoursWorked as data members. The class includes a constructor that takes as arguments a first name, a last name, a social security number, an hourlyWage and the hoursWorked. Declare set method s that assign new values to instance variables wage and hours, respectively. Method setWage ensures that wage is non negative, and method setHours ensures that hours is between 0 and 168 (the total number of hours in a week) inclusive. Class HourlyEmployee also includes get methods to return the values of wage and hours, respectively; a method earnings to calculate an HourlyEmployee’s earnings; and a method toString, which returns a String containing the employee’s type ("hourly employee: ")and the employee-specific information. The HourlyEmployee constructor, like the SalariedEmployee constructor, passes the first name, last name and social security number. Class CommissionEmployee having commissionRate and grossSales as data members extends class Employee. The class includes a constructor that takes a first name, a last name, a social security number, a sales amount and a commission rate. The constructor also passes the first name, last name and SSN along with its own data members. Class have set methods to assign new values to instance variables commissionRate and grossSales, respectively, get methods that retrieve the values of these instance variables; an override method earnings to calculate a CommissionEmployee’s earnings; and method toString which returns the employee’s type, namely, "commission employee: " and employee-specific information. To test our Employee hierarchy, the application. Creates an object of each of the three classes SalariedEmployee, HourlyEmployee, CommissionEmployee. The program manipulates these objects non polymorphically, via variables of each object’s own type. Then using polymorphically, an array of Employee type will be created having each type of employee as at each index of array. E.g. employees[ 0 ] = salariedEmployee; employees[ 1 ] = hourlyEmployee; employees[ 2 ] = commissionEmployee Finally, the program polymorphically determines and outputs the type of each object, its earnings and other details in the Employee array.
A company pays its employees on a weekly basis. The employees are of four types: Salariedemployeesarepaidafixedweeklysalaryregardlessofthenumberofhoursworked, hourly employees are paid by the hour and receive overtime pay (i.e., 1.5 times their hourly salary rate) for all hours worked in excess of 40 hours, commission employees are paid a percentage of their sales and base-salaried commission employees receive a base salary plus a percentage of their sales. For the current pay period, the company has decided to reward salaried-commission employees by adding 10% to their base salaries. The company wants to write an application that performs its payroll calculations polymorphically.
Create an EMPLOYEE class having First Name, Last Name, socialSecurityNumber(SSN);
- Provide a default Constructor.
- Provide overloaded parameterized Constructors.
- Provide getters, and setters for the data members.
- In addition there is method earnings which will return the earning of employees. For now you just simply prints a statement “calculate salary”.
Class SalariedEmployee extends class Employee having weeklySalary as a member and overrides method earnings. The class includes a constructor that takes a first name, a last name, a social security number and a weekly salary as arguments; a set method to assign a new non negative value to instance variable weeklySalary; a get method to return weeklySalary; a method earnings to calculate a SalariedEmployee’s earnings; and a method toString, which returns a String including the employee’s type, name and SSN.
Class HourlyEmployee also extends Employee and have hourlyWage and hoursWorked as data members. The class includes a constructor that takes as arguments a first name, a last name, a social security number, an hourlyWage and the hoursWorked. Declare set method s that assign new values to instance variables wage and hours, respectively. Method setWage ensures that wage is non negative, and method setHours ensures that hours is between 0 and 168 (the total number of hours in a week) inclusive.
Class HourlyEmployee also includes get methods to return the values of wage and hours, respectively; a method earnings to calculate an HourlyEmployee’s earnings; and a method toString, which returns a String containing the employee’s type ("hourly employee: ")and the employee-specific information. The HourlyEmployee constructor, like the SalariedEmployee constructor, passes the first name, last name and social security number.
Class CommissionEmployee having commissionRate and grossSales as data members extends class Employee.
The class includes a constructor that takes a first name, a last name, a social security number, a sales amount and a commission rate. The constructor also passes the first name, last name and SSN along with its own data members.
Class have set methods to assign new values to instance variables commissionRate and grossSales, respectively, get methods that retrieve the values of these instance variables; an override method earnings to calculate a CommissionEmployee’s earnings; and method toString which returns the employee’s type, namely, "commission employee: " and employee-specific information.
To test our Employee hierarchy, the application.
Creates an object of each of the three classes SalariedEmployee, HourlyEmployee, CommissionEmployee. The program manipulates these objects non polymorphically, via variables of each object’s own type.
Then using polymorphically, an array of Employee type will be created having each type of employee as at each index of array. E.g.
employees[ 0 ] = salariedEmployee;
employees[ 1 ] = hourlyEmployee;
employees[ 2 ] = commissionEmployee
Finally, the program polymorphically determines and outputs the type of each object, its earnings and other details in the Employee array.

Trending now
This is a popular solution!
Step by step
Solved in 8 steps with 15 images

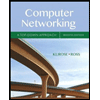
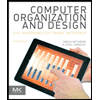
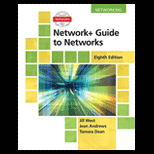
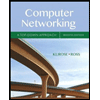
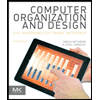
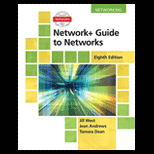
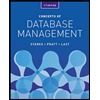
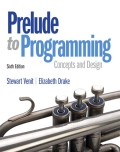
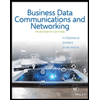