a) In the Hi-Lo game, the player begins with a score of 1000. The player is prompted for the number of points to risk and a second prompt asks the player to choose either High or Low. The player’s choice of either High or Low is compared to random number between 1 and 13, inclusive. If the number is between 1 and 6 inclusive, then it is considered “low”. A number between 8 and 13 inclusive is “high”. The number 7 is neither high nor low, and the player loses the points at risk. If the player had guessed correctly, the points at risk are doubled and added to the total points. For a wrong choice, the player loses the points at risk. Create a HiLo application based on this specification. Application output should look similar to: b) Modify the application to allow the player to continue until there are 0 points left. At the end of the game, display the number of guesses the user took before running out of points. This is my code I just need part b import java.util.Random; import java.util.Scanner; public class HiLoGame { public static void main(String[] args) { final int MAX_POINT = 1000; int point = MAX_POINT; //Main() method contains rules System.out.println("High Low Game."); System.out.println("RULES."); System.out.println("1. Numbers 1 through 6 are low ."); System.out.println("2. Numbers 8 through 13 are High ."); System.out.println("3. Numbers 7 is neither high or Low."); //rules(); //Showo the rules do { //displaying points Method displayPoints(point); int pointToRisk = enterPointsToRisk(point); String option = predictHiLo("Predict <1.High, 0. Low>: "); int min = 1; int max = 13; int computerRandomNum = randomNumgen(min, max); if (resultPoints(option, computerRandomNum)) { point += pointToRisk; System.out.println("You Won this game."); System.out.println("Now you have " + point+" to go"); } else { point -= pointToRisk; System.out.println("You Lost this game."); System.out.println("Now you have " + point+" to go"); } System.out.println("Do you want to play agin? y/n"); Scanner in = new Scanner(System.in); String select =in.nextLine(); if (select.equals("y")) { continue; } else {System.out.println("Bye!!"); break; } } while (point > 0); } //rules Method /* public static void rules() { System.out.println("High Low Game."); System.out.println("RULES."); System.out.println("1. Numbers 1 through 6 are low ."); System.out.println("2. Numbers 8 through 13 are High ."); System.out.println("3. Numbers 7 is neither high or Low."); }*/ //Displaying Points Method public static void displayPoints(int point) { System.out.println("You have " +point +"number of points to risk"); } //Random Number Genertor Method public static int randomNumgen(int min, int max) { int result = 1; Random rnd = new Random(); result = rnd.nextInt(13 - 1 + 1) + 1; System.out.println("Random " +result); return result; } //enterPointsToRisk Method public static int enterPointsToRisk(int point) { System.out.println("Enter Points to risk"); Scanner in = new Scanner(System.in); int inputPoints = in.nextInt(); if (inputPoints > point) { inputPoints = point; } return inputPoints; } //predictHiLow Method public static String predictHiLo(String i) { String result = "1"; do { System.out.println(i); Scanner in = new Scanner(System.in); String option = in.next(); } while (!true); return result; } //result points Method public static boolean resultPoints(String option, int guessNumber) { boolean result = false; if (option.equalsIgnoreCase("1") && (guessNumber >= 8)&& (guessNumber <= 13)) { result = true; } else if (option.equalsIgnoreCase("0") && (guessNumber <= 6)) { result = true; } return result; } }
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
a) In the Hi-Lo game, the player begins with a score of 1000. The player is prompted for the number of points to risk and a second prompt asks the player to choose either High or Low. The player’s choice of either High or Low is compared to random number between 1 and 13, inclusive. If the number is between 1 and 6 inclusive, then it is considered “low”. A number between 8 and 13 inclusive is “high”. The number 7 is neither high nor low, and the player loses the points at risk. If the player had guessed correctly, the points at risk are doubled and added to the total points. For a wrong choice, the player loses the points at risk. Create a HiLo application based on this specification. Application output should look similar to:
b) Modify the application to allow the player to continue until there are 0 points left. At the end of the game, display the number of guesses the user took before running out of points.
This is my code I just need part b
import java.util.Random;
import java.util.Scanner;
public class HiLoGame {
public static void main(String[] args) {
final int MAX_POINT = 1000;
int point = MAX_POINT;
//Main() method contains rules
System.out.println("High Low Game.");
System.out.println("RULES.");
System.out.println("1. Numbers 1 through 6 are low .");
System.out.println("2. Numbers 8 through 13 are High .");
System.out.println("3. Numbers 7 is neither high or Low.");
//rules(); //Showo the rules
do {
//displaying points Method
displayPoints(point);
int pointToRisk = enterPointsToRisk(point);
String option = predictHiLo("Predict <1.High, 0. Low>: ");
int min = 1;
int max = 13;
int computerRandomNum = randomNumgen(min, max);
if (resultPoints(option, computerRandomNum)) {
point += pointToRisk;
System.out.println("You Won this game.");
System.out.println("Now you have " + point+" to go");
} else {
point -= pointToRisk;
System.out.println("You Lost this game.");
System.out.println("Now you have " + point+" to go");
}
System.out.println("Do you want to play agin? y/n");
Scanner in = new Scanner(System.in);
String select =in.nextLine();
if (select.equals("y")) {
continue;
}
else
{System.out.println("Bye!!");
break;
}
} while (point > 0);
}
//rules Method
/* public static void rules()
{
System.out.println("High Low Game.");
System.out.println("RULES.");
System.out.println("1. Numbers 1 through 6 are low .");
System.out.println("2. Numbers 8 through 13 are High .");
System.out.println("3. Numbers 7 is neither high or Low.");
}*/
//Displaying Points Method
public static void displayPoints(int point) {
System.out.println("You have " +point +"number of points to risk");
}
//Random Number Genertor Method
public static int randomNumgen(int min, int max) {
int result = 1;
Random rnd = new Random();
result = rnd.nextInt(13 - 1 + 1) + 1;
System.out.println("Random " +result);
return result;
}
//enterPointsToRisk Method
public static int enterPointsToRisk(int point) {
System.out.println("Enter Points to risk");
Scanner in = new Scanner(System.in);
int inputPoints = in.nextInt();
if (inputPoints > point) {
inputPoints = point;
}
return inputPoints;
}
//predictHiLow Method
public static String predictHiLo(String i) {
String result = "1";
do {
System.out.println(i);
Scanner in = new Scanner(System.in);
String option = in.next();
} while (!true);
return result;
}
//result points Method
public static boolean resultPoints(String option, int guessNumber) {
boolean result = false;
if (option.equalsIgnoreCase("1") && (guessNumber >= 8)&& (guessNumber <= 13)) {
result = true;
} else if (option.equalsIgnoreCase("0") && (guessNumber <= 6)) {
result = true;
}
return result;
}
}
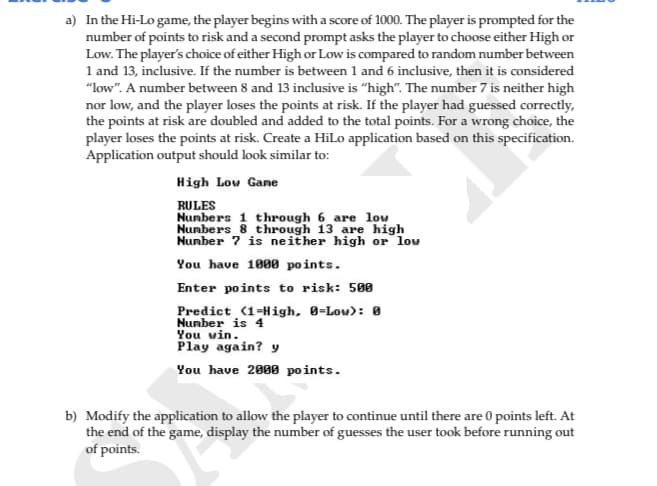

Code in the step 2
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

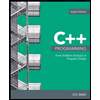
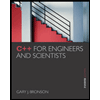
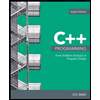
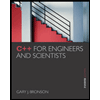