A set of integers 0..MAX may be implemented using an array of boolean values. This particular implementation is called a bit-vector implementation of a Set. Since C doesn’t have the Boolean data type, then substitute TRUE with 1 and FALSE with 0, or define 1 and 0 as TRUE and FALSE, respectively. For example, if the integer 3 is an element of the set, then the array element indexed by 3 is TRUE. On the other hand, if 3 is not an element, then the array element indexed by 3 is FALSE. For example, if the integer 3 is an element of the set, then the array element indexed by 3 is TRUE. On the other hand, if 3 is not an element, then the array element indexed by 3 is FALSE. For example: if s = {3,4,6,8}, the array looks like this: Implement a programmer-defined data type called BitSet to represent a set as follows: typedef int BitSet[MAX]; Implement the following functions: void initialize(BitSet s); - set all array elements to FALSE void add(int elem,BitSet s); - set the item indexed by elem to TRUE void display(BitSet s); - display the set on the screen using set notation, e.g. {3,4,5,6} - this means that you will print the index value if the content of that cell is TRUE void getUnion(BitSet result,BitSet s1,BitSet s2); - store in the array result the set resulting from the union of s1 and s2 - x is an element of s1 union s2 if x is an element of s1 or x is an element of s2 void intersection(BitSet result,BitSet s1,BitSet s2); - store in the array result the set resulting from the intersection of s1 and s2 - x is an element of s1 intersection s2 if x is an element of s1 and x is an element of s2 void difference(BitSet result,BitSet s1,BitSet s2); - store in the array result the set resulting from the difference of s1 and s2 - x is an element of s1 - s2 if x is an element of s1 and x is not an element of s2 int isEmpty(BitSet s); - the set is empty of all array elements are false int contains(BitSet s,int elem); - elem is an element of s if the array value indexed by elem is TRUE int disjoint(BitSet s1,BitSet s2); - two sets are disjoint if the intersection is empty int equal(BitSet s1,BitSet s2); - two sets are equal if they have exactly the same elements int cardinality(BitSet s); - the cardinality of the set is the number of TRUE elements int subset(BitSet s1,BitSet s2); - s1 is a subset of s2 if all elements of s1 are in s2
A set of integers 0..MAX may be implemented using an array of boolean values. This
particular implementation is called a bit-
the Boolean data type, then substitute TRUE with 1 and FALSE with 0, or define 1 and 0 as
TRUE and FALSE, respectively.
For example, if the integer 3 is an element of the set, then the array element indexed by 3 is
TRUE. On the other hand, if 3 is not an element, then the array element indexed by 3 is
FALSE.
For example, if the integer 3 is an element of the set, then the array element indexed by 3 is
TRUE. On the other hand, if 3 is not an element, then the array element indexed by 3 is
FALSE.
For example: if s = {3,4,6,8}, the array looks like this:
Implement a programmer-defined data type called BitSet to represent a set as follows:
typedef int BitSet[MAX];
Implement the following functions:
void initialize(BitSet s);
- set all array elements to FALSE
void add(int elem,BitSet s);
- set the item indexed by elem to TRUE
void display(BitSet s);
- display the set on the screen using set notation, e.g. {3,4,5,6}
- this means that you will print the index value if the content of that cell is TRUE
void getUnion(BitSet result,BitSet s1,BitSet s2);
- store in the array result the set resulting from the union of s1 and s2
- x is an element of s1 union s2 if x is an element of s1 or x is an element of s2
void intersection(BitSet result,BitSet s1,BitSet s2);
- store in the array result the set resulting from the intersection of s1 and s2
- x is an element of s1 intersection s2 if x is an element of s1 and x is an element of s2
void difference(BitSet result,BitSet s1,BitSet s2);
- store in the array result the set resulting from the difference of s1 and s2
- x is an element of s1 - s2 if x is an element of s1 and x is not an element of s2
int isEmpty(BitSet s);
- the set is empty of all array elements are false
int contains(BitSet s,int elem);
- elem is an element of s if the array value indexed by elem is TRUE
int disjoint(BitSet s1,BitSet s2);
- two sets are disjoint if the intersection is empty
int equal(BitSet s1,BitSet s2);
- two sets are equal if they have exactly the same elements
int cardinality(BitSet s);
- the cardinality of the set is the number of TRUE elements
int subset(BitSet s1,BitSet s2);
- s1 is a subset of s2 if all elements of s1 are in s2
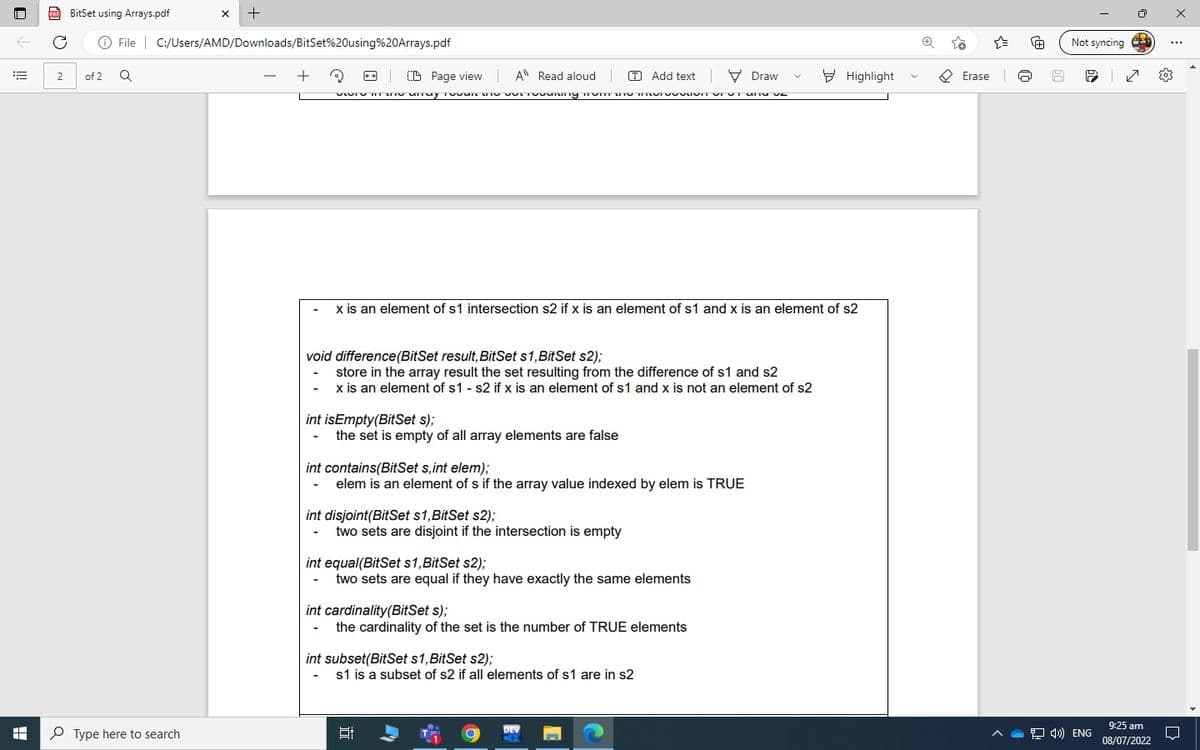
![11:3
H
PDF
C
1
BitSet using Arrays.pdf
File C:/Users/AMD/Downloads/BitSet%20using%20Arrays.pdf
+
of 2 Q
X +
Type here to search
CD Page view A Read aloud
A set of integers 0..MAX may be implemented using an array of boolean values. This
particular implementation is called a bit-vector implementation of a Set. Since C doesn't have
the Boolean data type, then substitute TRUE with 1 and FALSE with 0, or define 1 and 0 as
TRUE and FALSE, respectively.
-
For example, if the integer 3 is an element of the set, then the array element indexed by 3 is
TRUE. On the other hand, if 3 is not an element, then the array element indexed by 3 is
FALSE.
For example: if s = {3,4,6,8), the array looks like this:
0
1
2
3
4
5
6
7
8
9
FALSE FALSE FALSE TRUE TRUE FALSE TRUE FALSE TRUE FALSE
TAdd textDraw
Description
Implement a programmer-defined data type called BitSet to represent a set as follows:
typedef int BitSet[MAX];
Implement the following functions:
void initialize(BitSet s);
set all array elements to FALSE
void add(int elem, BitSet s);
set the item indexed by elem to TRUE
void display(BitSet s);
display the set on the screen using set notation, e.g. {3,4,5,6)
- this means that you will print the index value if the content of that cell is TRUE
void getUnion (BitSet result, BitSet s1, BitSet s2);
store in the array result the set resulting from the union of s1 and s2
- x is an element of s1 union s2 if x is an element of s1 or x is an element of s2
DEV
C+4
Highlight
void intersection (BitSet result, BitSet s1, BitSet s2);
store in the array result the set resulting from the intersection of s1 and s2
1²
[+ Not syncing 1
50
Erase A 8
№.9
4) ENG
9:24 am
08/07/2022
...
LI](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9490f4b0-0337-4e00-bf69-d25dd1e500d1%2F8861646d-b1c9-44c1-92bd-751d4a134c43%2Ftoe936k_processed.jpeg&w=3840&q=75)

Step by step
Solved in 4 steps with 7 images

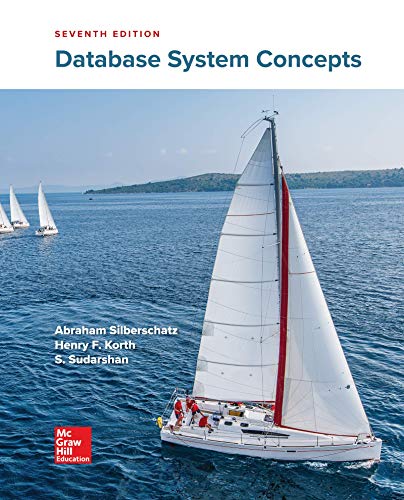
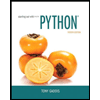
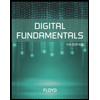
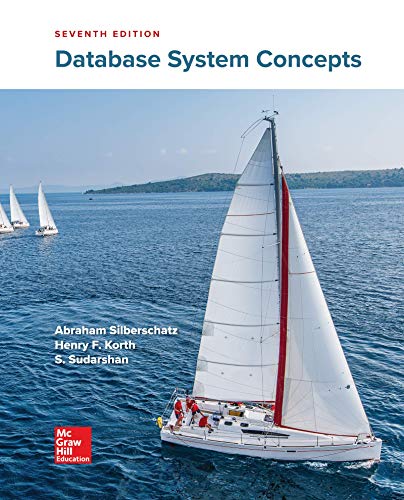
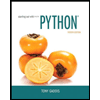
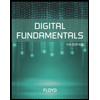
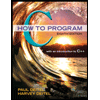
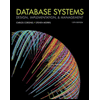
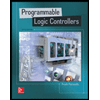