ABC Bank needs to store the information of all the users. For a user, the information that the bank holds is the user's name, username, password, contact number. Read this data from the console and store it in a file named "example.txt". Write a C++ program to read inputs from the console and write the user details into a file as the comma-separated values. Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. File names used should be the same as mentioned in the problem description. The class named User has the following private member variables Data type Variable name string name string username string password string contactnumber Define the following member function in the class UserBO. Method name Description void writeUserdetails(ofstream &file,User obj) This function accepts a user object and an ofstream file object. The function is used to write the data into the file. In the main method, read the input from the user through the console and open a file named "example.txt", into which the data are needed to be written. Pass the file object to the writeUserdetails() function and write the inputs as comma-separated-values into the file. Input and Output format: Refer sample input and output for formatting specifications. Sample input and output 1: [All text in bold corresponds to input and rest corresponds to output] Enter the name of user: ramesh Enter the contact number: 900900901 Enter the username: ramesh123 Enter the password: qwertyramesh Datas written in file successfully sample output in file: (example.txt) ramesh,900900901,ramesh123,qwertyramesh --------STRICTLY USE BELOW TEMPLATES ONLY WHILE MAKING SOLUTION-------- UserBo.cpp #include #include #include #include #include #include #include #include"User.cpp" using namespace std; class UserBO { public: void writeUserdetails(ofstream &file,User obj){ //fill your code here. } }; User.cpp #include #include #include #include #include #include #include using namespace std; class User { private: string name; string username; string password; string contactnumber; public: void setName(string name) { this->name = name; } string getName() { return this->name; } void setUsername(string username) { this->username = username; } string getUsername() { return this->username; } void setPassword(string password) { this->password = password; } string getPassword() { return this->password; } void setContactnumber(string contactnumber) { this->contactnumber = contactnumber; } string getContactnumber() { return this->contactnumber; } };
ABC Bank needs to store the information of all the users. For a user, the information that the bank holds is the user's name, username, password, contact number. Read this data from the console and store it in a file named "example.txt".
Write a C++ program to read inputs from the console and write the user details into a file as the comma-separated values.
Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement. File names used should be the same as mentioned in the problem description.
The class named User has the following private member variables
Data type | Variable name |
string | name |
string | username |
string | password |
string | contactnumber |
Define the following member function in the class UserBO.
Method name | Description |
void writeUserdetails(ofstream &file,User obj) |
This function accepts a user object and an ofstream file object. The function is used to write the data into the file. |
In the main method, read the input from the user through the console and open a file named "example.txt", into which the data are needed to be written. Pass the file object to the writeUserdetails() function and write the inputs as comma-separated-values into the file.
Input and Output format:
Refer sample input and output for formatting specifications.
Sample input and output 1:
[All text in bold corresponds to input and rest corresponds to output]
Enter the name of user:
ramesh
Enter the contact number:
900900901
Enter the username:
ramesh123
Enter the password:
qwertyramesh
Datas written in file successfully
sample output in file: (example.txt)
ramesh,900900901,ramesh123,qwertyramesh
--------STRICTLY USE BELOW TEMPLATES ONLY WHILE MAKING SOLUTION--------
UserBo.cpp
#include<iostream> #include<string> #include<stdio.h> #include<fstream> #include<list> #include<iterator> #include<sstream> #include"User.cpp" using namespace std; class UserBO { public: void writeUserdetails(ofstream &file,User obj){ //fill your code here. } }; |
User.cpp
#include<iostream> }; |
main.cpp
#include<iostream> #include<string> #include<stdio.h> #include<fstream> #include<list> #include<iterator> #include<sstream> #include"UserBO.cpp" using namespace std; int main() { //fill your code here. return 0; } |
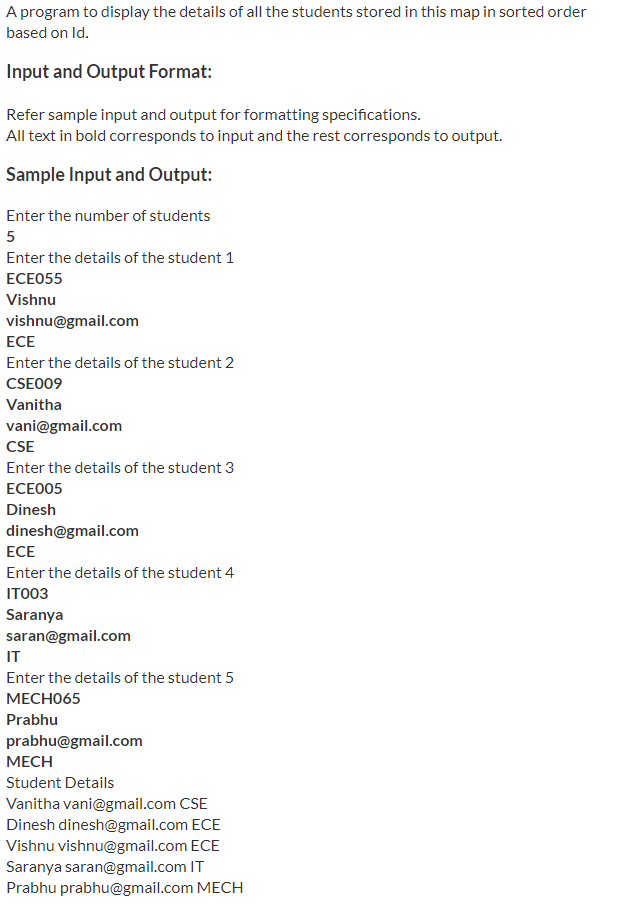

Step by step
Solved in 9 steps with 5 images
