am attempting to remake a diagram that shows the process of my color mixer program in C++. I have learned a new method of calling functions in a certain order to make the code nicer. I am still having some trouble learning how to make a flow-chart that uses proper shapes and symbols to diagram the program in a flow chart. What are the best practices for the most professional flow-chart? My program is: #include #include #include #include using namespace std; string mixColors(int color1, int color2) { string mixedColor; if ((color1 == 0 && color2 == 1) || (color1 == 1 && color2 == 0)) { mixedColor = "purple"; } else if ((color1 == 0 && color2 == 2) || (color1 == 2 && color2 == 0)) { mixedColor = "orange"; } else if ((color1 == 1 && color2 == 2) || (color1 == 2 && color2 == 1)) { mixedColor = "green"; } else { mixedColor = "No Mix"; } return mixedColor; } int getUserInput() { int color; cout << "Enter a prime color: " << endl; cout << "'0' for red, '1' for blue', and '2' for yellow: "; while (!(cin >> color) || color < 0 || color > 2) { cout << "Invalid input! Please enter a valid color ('0', '1', or '2'): "; cin.clear(); cin.ignore(numeric_limits::max(), '\n'); } return color; } int main() { string arr[] = {"red", "blue", "yellow"}; int color1, color2; string mixedColor; char choice; ofstream outputFile("color_mixer_results.txt"); // opens file that truncates do { color1 = getUserInput(); color2 = getUserInput(); mixedColor = mixColors(color1, color2); // below is where input inside do while loop is writ to .txt file outputFile << "Mixed color for your selection (" << color1 << " and " << color2 << ") is " << mixedColor << endl; cout << "Mixed color for your selection (" << color1 << " and " << color2 << ") is " << mixedColor << endl; cout << "Would you like to mix again? (Y/N): "; cin >> choice; } while (toupper(choice) == 'Y'); outputFile.close(); // closing output file cout << "Results have been saved in 'color_mix_results.txt'. Goodbye!" << endl; return
am attempting to remake a diagram that shows the process of my color mixer program in C++. I have learned a new method of calling functions in a certain order to make the code nicer. I am still having some trouble learning how to make a flow-chart that uses proper shapes and symbols to diagram the program in a flow chart. What are the best practices for the most professional flow-chart? My program is: #include #include #include #include using namespace std; string mixColors(int color1, int color2) { string mixedColor; if ((color1 == 0 && color2 == 1) || (color1 == 1 && color2 == 0)) { mixedColor = "purple"; } else if ((color1 == 0 && color2 == 2) || (color1 == 2 && color2 == 0)) { mixedColor = "orange"; } else if ((color1 == 1 && color2 == 2) || (color1 == 2 && color2 == 1)) { mixedColor = "green"; } else { mixedColor = "No Mix"; } return mixedColor; } int getUserInput() { int color; cout << "Enter a prime color: " << endl; cout << "'0' for red, '1' for blue', and '2' for yellow: "; while (!(cin >> color) || color < 0 || color > 2) { cout << "Invalid input! Please enter a valid color ('0', '1', or '2'): "; cin.clear(); cin.ignore(numeric_limits::max(), '\n'); } return color; } int main() { string arr[] = {"red", "blue", "yellow"}; int color1, color2; string mixedColor; char choice; ofstream outputFile("color_mixer_results.txt"); // opens file that truncates do { color1 = getUserInput(); color2 = getUserInput(); mixedColor = mixColors(color1, color2); // below is where input inside do while loop is writ to .txt file outputFile << "Mixed color for your selection (" << color1 << " and " << color2 << ") is " << mixedColor << endl; cout << "Mixed color for your selection (" << color1 << " and " << color2 << ") is " << mixedColor << endl; cout << "Would you like to mix again? (Y/N): "; cin >> choice; } while (toupper(choice) == 'Y'); outputFile.close(); // closing output file cout << "Results have been saved in 'color_mix_results.txt'. Goodbye!" << endl; return
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter7: User-defined Simple Data Types, Namespaces, And The String Type
Section: Chapter Questions
Problem 5SA
Related questions
Question
I am attempting to remake a diagram that shows the process of my color mixer
I have learned a new method of calling functions in a certain order to make the code nicer. I am still having some trouble learning how to make a flow-chart that uses proper shapes and symbols to diagram the program in a flow chart.
What are the best practices for the most professional flow-chart?
My program is:
#include <iostream>
#include <fstream>
#include <string>
#include <limits>
using namespace std;
string mixColors(int color1, int color2) {
string mixedColor;
if ((color1 == 0 && color2 == 1) || (color1 == 1 && color2 == 0)) {
mixedColor = "purple";
} else if ((color1 == 0 && color2 == 2) || (color1 == 2 && color2 == 0)) {
mixedColor = "orange";
} else if ((color1 == 1 && color2 == 2) || (color1 == 2 && color2 == 1)) {
mixedColor = "green";
} else {
mixedColor = "No Mix";
}
return mixedColor;
}
int getUserInput() {
int color;
cout << "Enter a prime color: " << endl;
cout << "'0' for red, '1' for blue', and '2' for yellow: ";
while (!(cin >> color) || color < 0 || color > 2) {
cout << "Invalid input! Please enter a valid color ('0', '1', or '2'): ";
cin.clear();
cin.ignore(numeric_limits<streamsize>::max(), '\n');
}
return color;
}
int main() {
string arr[] = {"red", "blue", "yellow"};
int color1, color2;
string mixedColor;
char choice;
ofstream outputFile("color_mixer_results.txt"); // opens file that truncates
do {
color1 = getUserInput();
color2 = getUserInput();
mixedColor = mixColors(color1, color2);
// below is where input inside do while loop is writ to .txt file
outputFile << "Mixed color for your selection (" << color1 << " and " << color2 << ") is " << mixedColor << endl;
cout << "Mixed color for your selection (" << color1 << " and " << color2 << ") is " << mixedColor << endl;
cout << "Would you like to mix again? (Y/N): ";
cin >> choice;
} while (toupper(choice) == 'Y');
outputFile.close(); // closing output file
cout << "Results have been saved in 'color_mix_results.txt'. Goodbye!" << endl;
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
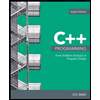
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
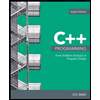
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning