Answer in java language Problem Description: The Example University wanted to reduce wrong student number entries on their systems. For that reason, they designed the student numbering system to have an embedded safety mechanism. The purpose is to check whether student number entered on the website or any other information system is correct or wrong by looking at the digits. If one or more digits are entered incorrectly, the number will not validate and systems will show error message to user. Student numbers are always 8 digits long. The validation mechanism is described as follows: Take digits at odd positions from left to right and add them up. Take digits at even positions from left to right, multiply each one of those digits by two, if result have two digits (in other words result is greater than 9) sum digits of the result to have a single digit result, and add all of them up. If sum of result from step 1 and step 2 is multiple of 10, the student number is valid, otherwise it is invalid. Example 1: Let us check whether student number 15743602 is valid or not. Step 1. Take digits at odd positions from left to right and add them up. 1+7+3+0=11 Step 2. Take digits at even positions from left to right, 5462 multiply each one of those digits by two, 5*2=10 4*2=8 6*2=12 2*2=4 if result have two digits (in other words result is greater than 9) sum digits of the result to have a single digit result, 10 (1+0=1) 8 12 (1+2=3) 4 and add all of them up. 1+8+3+4=16 Step 3. If sum of result from step 1 and step 2 is multiple of 10, the student number is valid, otherwise it is invalid. 11+16=27 27 is not divisible by ten. Student number 15743602 is invalid. Example 2: Another example where the student number 88110464 is valid: Step 1. Take digits at odd positions from left to right and add them up. 8+1+0+6=15 Step 2. Take digits at even positions from left to right, 8144 multiply each one of those digits by two, 8*2=161*2=24*2=84*2=8 if result have two digits (in other words result is greater than 9) sum digits of the result to have a single digit result, 16 (1+6=7) 288 and add all of them up. 7+2+8+8=25 Step 3. If sum of result from step 1 and step 2 is multiple of 10, the student number is valid, otherwise it is invalid. 15 + 25 = 40. Student number 88110464 is valid. Requirement: Write a program that reads student numbers from user input and classifies "valid" and "invalid" numbers. Program should have two arrays of integers for valid and invalid student numbers. Arrays should be empty initially. Array sizes should be set as 20, with the assumption that the user will enter at most 20 student numbers. Program should start asking for an integer from user input. It should be assumed that user always enters numbers, error handling is not necessary if user enters letters or characters other than numbers. If the student number is valid according to above mentioned validation steps, it should be added to "valid numbers" array. If the number is invalid, it should be added to "invalid numbers" array. If the user enters number 0, the program should display valid and invalid numbers arrays and stop.
Answer in java language Problem Description: The Example University wanted to reduce wrong student number entries on their systems. For that reason, they designed the student numbering system to have an embedded safety mechanism. The purpose is to check whether student number entered on the website or any other information system is correct or wrong by looking at the digits. If one or more digits are entered incorrectly, the number will not validate and systems will show error message to user. Student numbers are always 8 digits long. The validation mechanism is described as follows: Take digits at odd positions from left to right and add them up. Take digits at even positions from left to right, multiply each one of those digits by two, if result have two digits (in other words result is greater than 9) sum digits of the result to have a single digit result, and add all of them up. If sum of result from step 1 and step 2 is multiple of 10, the student number is valid, otherwise it is invalid. Example 1: Let us check whether student number 15743602 is valid or not. Step 1. Take digits at odd positions from left to right and add them up. 1+7+3+0=11 Step 2. Take digits at even positions from left to right, 5462 multiply each one of those digits by two, 5*2=10 4*2=8 6*2=12 2*2=4 if result have two digits (in other words result is greater than 9) sum digits of the result to have a single digit result, 10 (1+0=1) 8 12 (1+2=3) 4 and add all of them up. 1+8+3+4=16 Step 3. If sum of result from step 1 and step 2 is multiple of 10, the student number is valid, otherwise it is invalid. 11+16=27 27 is not divisible by ten. Student number 15743602 is invalid. Example 2: Another example where the student number 88110464 is valid: Step 1. Take digits at odd positions from left to right and add them up. 8+1+0+6=15 Step 2. Take digits at even positions from left to right, 8144 multiply each one of those digits by two, 8*2=161*2=24*2=84*2=8 if result have two digits (in other words result is greater than 9) sum digits of the result to have a single digit result, 16 (1+6=7) 288 and add all of them up. 7+2+8+8=25 Step 3. If sum of result from step 1 and step 2 is multiple of 10, the student number is valid, otherwise it is invalid. 15 + 25 = 40. Student number 88110464 is valid. Requirement: Write a program that reads student numbers from user input and classifies "valid" and "invalid" numbers. Program should have two arrays of integers for valid and invalid student numbers. Arrays should be empty initially. Array sizes should be set as 20, with the assumption that the user will enter at most 20 student numbers. Program should start asking for an integer from user input. It should be assumed that user always enters numbers, error handling is not necessary if user enters letters or characters other than numbers. If the student number is valid according to above mentioned validation steps, it should be added to "valid numbers" array. If the number is invalid, it should be added to "invalid numbers" array. If the user enters number 0, the program should display valid and invalid numbers arrays and stop.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Warning ⚠️ Don't post AI generated answer or plagiarised answer. If I see these things I'll give you multiple downvotes and will report immediately.

Transcribed Image Text:Answer in java language
Problem Description:
The Example University wanted to reduce wrong student number entries on their
systems. For that reason, they designed the student numbering system to have an
embedded safety mechanism. The purpose is to check whether student number
entered on the website or any other information system is correct or wrong by looking
at the digits. If one or more digits are entered incorrectly, the number will not validate
and systems will show error message to user.
Student numbers are always 8 digits long.
The validation mechanism is described as follows:
Take digits at odd positions from left to right and add them up.
Take digits at even positions from left to right, multiply each one of those digits by
two, if result have two digits (in other words result is greater than 9) sum digits of the
result to have a single digit result, and add all of them up.
If sum of result from step 1 and step 2 is multiple of 10, the student number is valid,
otherwise it is invalid.
Example 1:
Let us check whether student number 15743602 is valid or not.
Step 1. Take digits at odd positions from left to right and add them up.
1+7+3+0=11
Step 2. Take digits at even positions from left to right,
5462
multiply each one of those digits by two,
5*2=10
4*2=8
6*2=12
2*2=4
if result have two digits (in other words result is greater than 9) sum digits of the result
to have a single digit result,
10 (1+0=1)
8
12 (1+2=3)
4
and add all of them up.
1+8+3+4=16
Step 3. If sum of result from step 1 and step 2 is multiple of 10, the student number is
valid, otherwise it is invalid.
11 +16=27
27 is not divisible by ten. Student number 15743602 is invalid.
Example 2:
Another example where the student number 88110464 is valid:
Step 1. Take digits at odd positions from left to right and add them up.
8+1+0+6=15
Step 2. Take digits at even positions from left to right,
8144
multiply each one of those digits by two,
8*2=161*2=24*2=84*2=8
if result have two digits (in other words result is greater than 9) sum digits of the result
to have a single digit result,
16 (1+6=7) 288
and add all of them up.
7+2+8+8= 25
Step 3. If sum of result from step 1 and step 2 is multiple of 10, the student number is
valid, otherwise it is invalid.
15 + 25 = 40.
Student number 88110464 is valid.
Requirement:
Write a program that reads student numbers from user input and classifies "valid" and
"invalid" numbers. Program should have two arrays of integers for valid and invalid
student numbers. Arrays should be empty initially. Array sizes should be set as 20,
with the assumption that the user will enter at most 20 student numbers. Program
should start asking for an integer from user input. It should be assumed that user
always enters numbers, error handling is not necessary if user enters letters or
characters other than numbers. If the student number is valid according to above
mentioned validation steps, it should be added to "valid numbers" array. If the number
is invalid, it should be added to "invalid numbers" array. If the user enters number 0,
the program should display valid and invalid numbers arrays and stop.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
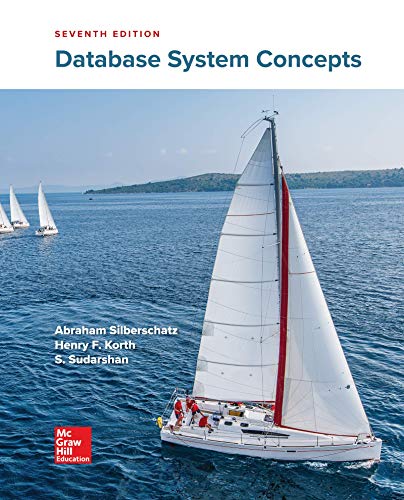
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
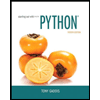
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
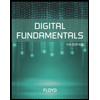
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
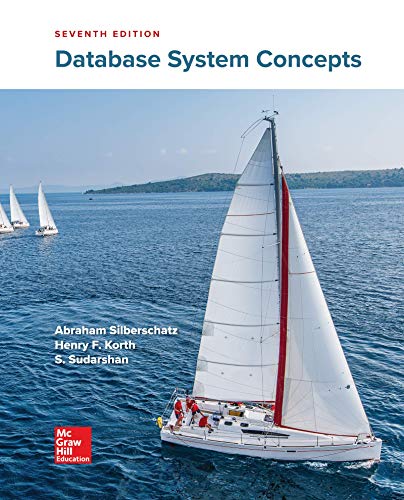
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
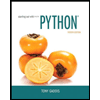
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
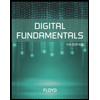
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
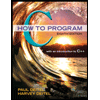
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
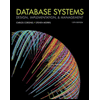
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
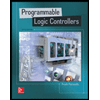
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education