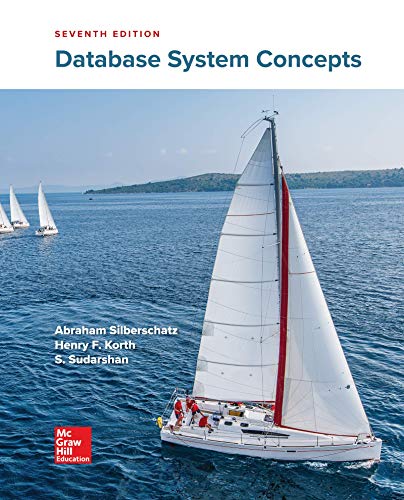
I have a problem with my code in java, replit. When I run my code in the console, the output for the csv file is always the random ID number, null, 0,0,0. I don't want null, 0,0,0. null,0,0,0 reflects name, totalgames, totalguesses, and bestgame. When I run my program it doesn't give the actual value of totalgames, totalguesses, and bestgame just 0,0,0 every time. Could you fix this? Also name is null because I haven't asked for the player's name, so I would like you to include that as well in this code.
Here is the code, each class in seperate files in replit:
import java.util.Scanner;
import java.util.Random;
public class Main {
public static void main(String[] args) {
Scanner console = new Scanner(System.in);
Random random = new Random();
Game game = new Game(console, random);
game.startGame();
console.close();
}
}
import java.util.Scanner;
import java.util.UUID;
public class Player {
private UUID id;
private String name;
private int totalGames;
private int totalGuesses;
private int bestGame;
public Player() {
this.id = UUID.randomUUID();
}
public UUID getId() {
return id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getTotalGames() {
return totalGames;
}
public void setTotalGames(int totalGames) {
this.totalGames = totalGames;
}
public int getTotalGuesses() {
return totalGuesses;
}
public void setTotalGuesses(int totalGuesses) {
this.totalGuesses = totalGuesses;
}
public int getBestGame() {
return bestGame;
}
public void setBestGame(int bestGame) {
this.bestGame = bestGame;
}
public int playGame(Scanner console, int answer) {
System.out.println("I'm thinking of a number between 1 and " + Game.MAX + "...");
int guesses = 0;
while (true) {
System.out.print("Your guess? ");
if (console.hasNextInt()) {
int guess = console.nextInt();
guesses++;
if (guess == answer) {
System.out.println("You got it right in " + guesses + " guess" + (guesses == 1 ? "!" : "es!"));
break;
} else if (guess < answer) {
System.out.println("It's higher.");
} else {
System.out.println("It's lower.");
}
} else {
System.out.println("Invalid input. Please enter a valid integer.");
console.next(); // consume invalid input
}
}
return guesses;
}
}
import java.io.*;
import java.util.Scanner;
import java.util.Random;
public class Game {
public static final int MAX = 100;
private final Scanner console;
private final Random random;
private static final String CSV_FILE_PATH = "player_results.csv";
public Game(Scanner console, Random random) {
this.console = console;
this.random = random;
}
public void startGame() {
int totalGames = 0;
int totalGuesses = 0;
int bestGame = Integer.MAX_VALUE;
while (true) {
Player player = new Player();
int answer = 1 + random.nextInt(MAX);
int guesses = player.playGame(console, answer);
totalGames++;
totalGuesses += guesses;
bestGame = Math.min(bestGame, guesses);
// Save player information to CSV
savePlayerToCSV(player);
System.out.println("Play again y/n?");
String playAgain = console.next().toLowerCase();
if (!playAgain.startsWith("y")) {
break;
}
}
printOverallStatistics(totalGames, totalGuesses, bestGame);
}
private void printOverallStatistics(int totalGames, int totalGuesses, int bestGame) {
System.out.println("Total games: " + totalGames);
System.out.println("Total guesses: " + totalGuesses);
System.out.println("Guesses/game: " + (totalGames == 0 ? 0 : totalGuesses / totalGames));
System.out.println("Best game: " + bestGame);
}
private void savePlayerToCSV(Player player) {
try (FileWriter writer = new FileWriter(CSV_FILE_PATH, true);
BufferedWriter bw = new BufferedWriter(writer);
PrintWriter out = new PrintWriter(bw)) {
out.println(player.getId() + "," +
player.getName() + "," +
player.getTotalGames() + "," +
player.getTotalGuesses() + "," +
player.getBestGame());
} catch (IOException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
Scanner console = new Scanner(System.in);
Random random = new Random();
Game game = new Game(console, random);
game.startGame();
console.close();
}
}
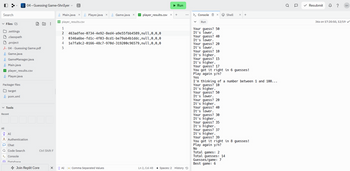

Step by stepSolved in 3 steps

- Please help me fix the errors in this java program. I can’t get the program to work Class: AnimatedBall Import java.swing.*; import java.awt.*; import java.awt.event.*; public class AnimatedBall extends JFrame implements ActionListener { private Button btnBounce; // declare a button to play private TextField txtSpeed; // declare a text field to enter number private Label lblDelay; private Panel controls; // generic panel for controls private BouncingBall display; // drawing panel for ball Container frame; public AnimatedBall () { // Set up the controls on the applet frame = getContentPane(); btnBounce = new Button ("Bounce Ball"); //create the objects txtSpeed = new TextField("10000", 10); lblDelay = new Label("Enter Delay"); display = new BouncingBall();//create the panel objects controls = new Panel(); setLayout(new BorderLayout()); // set the frame layout controls.add (btnBounce); // add controls to panel controls.add (lblDelay); controls.add (txtSpeed); frame.add(controls,…arrow_forwardTHIS IS MEANT TO BE IN JAVA. What we've learned so far is variables, loops, and we just started learning some array today. The assignment is to get an integer from input, and output that integer squared, ending with newline. But i've been given some instructions that are kind of confusing to me. Please explain why and please show what the end result is. Here are some extra things i've been told to do with the small amount of code i've already written... Type 2 in the input box, then run the program so I can note that the answer is 4 Type 3 in the input box instead, run, and note the output is 6. Change the output statement to output a newline: System.out.println(userNumSquared);. Type 2 in the input box Change the program to use * rather than +, and try running with input 2 (output is 4) and 3 (output is now 9, not 6 as before). This is what I have so far... import java.util.Scanner; public class NumSquared {public static void main(String[] args) {Scanner scnr = new…arrow_forwardPlease make a java gui ( the program make a tic tac toe grid with black and red X’s and O’s and then there should also be a score board. Make it similar to the one belowarrow_forward
- I'm having some trouble coding this certain prompt in Eclipse and I don't know what I'm doing wrong. This is the prompt below: Write a program that prompts a user for their first name (a String), last name (a String), and age (an integer). Then print the last name, followed by a comma, a space, the first name, followed by a space, a colon, a space, and the age (all on the same line). For example: Please enter your first and last name: Harry MorganPlease enter your age: 34Morgan, Harry : 34 This is the code I have now: package lab; public class Lab3 {public static void main(String[] args) {importjava.util.scanner;new.scanner(System.in);System.out.print("Please enter your first and last name: ");innextInt(); } } If you can get back to me as soon as possible, that would be great!!arrow_forwardHello! I am having a problem trying to code a addNode method implementation to my program. I am trying to only get it to sort the lines alphabetically and then the categories will be sorted from the way it is first read in the input text file.So an input file would look like this: Name Clovis Bagwell Interesting The quick brown fox jumped over the lazy dog. Interesting Shake rattle and roll. Name Archibald Beechcroft and the output file should look like this: Name Archibald Beechcroft Clovis Bagwell Interesting Shake rattle and roll. The quick brown fox jumped over the lazy dog. My addNode code keeps organizing all of the categories and the lines in alphabetical order when categories is supposed to be organized by the way they are first read in the text file, and the lines under it are alphabetical.arrow_forwardhow to write a method thar reads in any csv file and extracts the data from the csv file into header and the remaining data using the csv module, and stores them in instance variables. also how to write a code to handle potential errors with file content for example empty file and file not foundarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
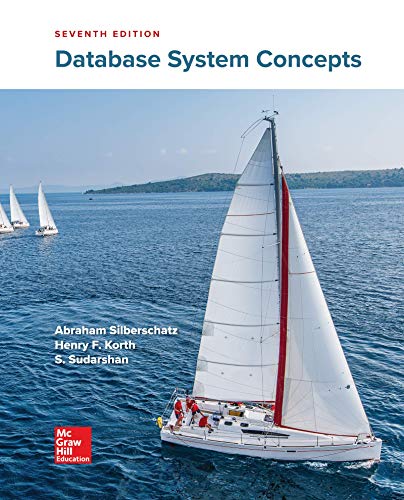
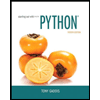
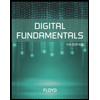
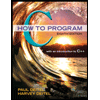
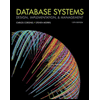
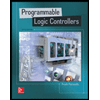