- Arithmetic Dictionaries At the core of our sock drawer implementation is a fundamental data structure which, for some reason, does not come standard in programming languages: a dictionary that has numerical values (and arbitrary keys). A number is a number. An array, or vector, is a list of numbers that can be indexed by their position in the vector. An arithmetic dictionary is a set of numbers that can be addressed by their associated key. Just as numbers, and arrays, can be combined via +, -, *, 7, and compared with >, <, etc, so can arithmetic dictionaries. Let us start defining them. For brevity, we call them simply AD rather than ArithmeticDictionary. We note that Python includes, in the collections module, the counter class, which supports addition. ADs are a more general version of the counter class, as they support (or you can make them to support) all math operations you wish. In Python, to be able to have two ADs d1 and d2 and compute their sum via the syntax d1 + d2, we need to define the _add_ operation, and similarly for the other arithmetic operations. There is a fine point to be discussed. Arrays can be combined with arithmetical operations only when they are of the same dimensions, or when one can be "broadcast" to fit the dimension of the other. For dictionaries, however, the restriction to be able to combine only arithmetic dictionaries with the same keys would be too restrictive. If we have an AD d representing a sock drawer, and we want to add to it one yellow and two blue socks, we want to be able to write something like: d += AD({'yellow': 1, 'blue': 2}) rather than worry that d might have different keys from {yellow', 'blue'}. Thus, in the definition of an operation, we will need to define suitable defaults for the missing values. In general, the suitable defaults are the neutral elements with respect to the operation: 0 for+ and –, and 1 for x and /. Let us begin from addition and subtraction. [] class AD(dict): def _init_(self, *args, **kwargs): "*"This initializer simply passes all arguments to dict, so that we can create an AD with the same ease with which we can create a dict. There is no need, indeed, to repeat the initializer, but we leave it here in case we like to create attributes specific of an AD later.""" super ()._init_(*args, **kwargs) def _add_(self, other): return AD._binary_op(self, other, lambda x, y: x + y, e) def _sub_(self, other): return AD._binary_op(self, other, lambda x, y: x - y, e) @staticmethod def _binary_op(left, right, op, neutral): r- AD() 1_keys = set(left.keys()) if isinstance(left, dict) else set() r_keys - set(right.keys()) if isinstance(right, dict) else set() for k in 1_keys | r_keys: # If the right (or left) element is a dictionary (or an AD), # we get the elements from the dictionary; else we use the right # or left value itself. This implements a sort of dictionary # broadcasting. 1_val - left.get(k, neutral) if isinstance(left, dict) else left r_val = right.get (k, neutral) if isinstance(right, dict) else right r[k] = op(1_val, r_val) return r
- Arithmetic Dictionaries At the core of our sock drawer implementation is a fundamental data structure which, for some reason, does not come standard in programming languages: a dictionary that has numerical values (and arbitrary keys). A number is a number. An array, or vector, is a list of numbers that can be indexed by their position in the vector. An arithmetic dictionary is a set of numbers that can be addressed by their associated key. Just as numbers, and arrays, can be combined via +, -, *, 7, and compared with >, <, etc, so can arithmetic dictionaries. Let us start defining them. For brevity, we call them simply AD rather than ArithmeticDictionary. We note that Python includes, in the collections module, the counter class, which supports addition. ADs are a more general version of the counter class, as they support (or you can make them to support) all math operations you wish. In Python, to be able to have two ADs d1 and d2 and compute their sum via the syntax d1 + d2, we need to define the _add_ operation, and similarly for the other arithmetic operations. There is a fine point to be discussed. Arrays can be combined with arithmetical operations only when they are of the same dimensions, or when one can be "broadcast" to fit the dimension of the other. For dictionaries, however, the restriction to be able to combine only arithmetic dictionaries with the same keys would be too restrictive. If we have an AD d representing a sock drawer, and we want to add to it one yellow and two blue socks, we want to be able to write something like: d += AD({'yellow': 1, 'blue': 2}) rather than worry that d might have different keys from {yellow', 'blue'}. Thus, in the definition of an operation, we will need to define suitable defaults for the missing values. In general, the suitable defaults are the neutral elements with respect to the operation: 0 for+ and –, and 1 for x and /. Let us begin from addition and subtraction. [] class AD(dict): def _init_(self, *args, **kwargs): "*"This initializer simply passes all arguments to dict, so that we can create an AD with the same ease with which we can create a dict. There is no need, indeed, to repeat the initializer, but we leave it here in case we like to create attributes specific of an AD later.""" super ()._init_(*args, **kwargs) def _add_(self, other): return AD._binary_op(self, other, lambda x, y: x + y, e) def _sub_(self, other): return AD._binary_op(self, other, lambda x, y: x - y, e) @staticmethod def _binary_op(left, right, op, neutral): r- AD() 1_keys = set(left.keys()) if isinstance(left, dict) else set() r_keys - set(right.keys()) if isinstance(right, dict) else set() for k in 1_keys | r_keys: # If the right (or left) element is a dictionary (or an AD), # we get the elements from the dictionary; else we use the right # or left value itself. This implements a sort of dictionary # broadcasting. 1_val - left.get(k, neutral) if isinstance(left, dict) else left r_val = right.get (k, neutral) if isinstance(right, dict) else right r[k] = op(1_val, r_val) return r
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter13: Overloading And Templates
Section: Chapter Questions
Problem 15PE
Related questions
Question
'''Returns a pair consisting of the max value in the AD, and of the set of keys that attain that value. This can be done in 7 lines of code. ""
Question 2, please. Also please code in python. Fucus on the
def ad_max_items(self):]
""" Returns a pair consisting of the max value in the AD, and of the
set of keys that attain that value. This can be done in 7 lines of code."""
# YOUR CODE HERE
**important** You might have to right-click and open image in a new tab for a clearer image
![- Arithmetic Dictionaries
At the core of our sock drawer implementation is a fundamental data structure which, for some reason, does not come standard in
programming languages: a dictionary that has numerical values (and arbitrary keys).
A number is a number. An array, or vector, is a list of numbers that can be indexed by their position in the vector. An arithmetic dictionary is a set
of numbers that can be addressed by their associated key. Just as numbers, and arrays, can be combined via +, -, *, 7, and compared with >, <,
etc, so can arithmetic dictionaries. Let us start defining them. For brevity, we call them simply AD rather than ArithmeticDictionary. We note that
Python includes, in the collections module, the counter class, which supports addition. ADs are a more general version of the counter class,
as they support (or you can make them to support) all math operations you wish.
In Python, to be able to have two ADs d1 and d2 and compute their sum via the syntax d1 + d2, we need to define the _add_ operation, and
similarly for the other arithmetic operations.
There is a fine point to be discussed. Arrays can be combined with arithmetical operations only when they are of the same dimensions, or when
one can be "broadcast" to fit the dimension of the other. For dictionaries, however, the restriction to be able to combine only arithmetic
dictionaries with the same keys would be too restrictive. If we have an AD d representing a sock drawer, and we want to add to it one yellow
and two blue socks, we want to be able to write something like:
d += AD({'yellow': 1, 'blue': 2})
rather than worry that d might have different keys from {yellow', 'blue'}. Thus, in the definition of an operation, we will need to define suitable
defaults for the missing values. In general, the suitable defaults are the neutral elements with respect to the operation: 0 for+ and –, and 1 for
x and /.
Let us begin from addition and subtraction.
[] class AD(dict):
def _init_(self, *args, **kwargs):
"*"This initializer simply passes all arguments to dict, so that
we can create an AD with the same ease with which we can create
a dict.
There is no need, indeed, to repeat the initializer,
but we leave it here in case we like to create attributes specific
of an AD later."""
super ()._init_(*args, **kwargs)
def _add_(self, other):
return AD._binary_op(self, other, lambda x, y: x + y, e)
def _sub_(self, other):
return AD._binary_op(self, other, lambda x, y: x - y, e)
@staticmethod
def _binary_op(left, right, op, neutral):
r- AD()
1_keys = set(left.keys()) if isinstance(left, dict) else set()
r_keys - set(right.keys()) if isinstance(right, dict) else set()
for k in 1_keys | r_keys:
# If the right (or left) element is a dictionary (or an AD),
# we get the elements from the dictionary; else we use the right
# or left value itself. This implements a sort of dictionary
# broadcasting.
1_val - left.get(k, neutral) if isinstance(left, dict) else left
r_val = right.get (k, neutral) if isinstance(right, dict) else right
r[k] = op(1_val, r_val)
return r](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F293032ce-89a7-4d5a-8a29-aae96cb1d151%2F63ec4d9f-1188-4c63-b9b9-3c7a4ac39c76%2Fzg2nmax_processed.png&w=3840&q=75)
Transcribed Image Text:- Arithmetic Dictionaries
At the core of our sock drawer implementation is a fundamental data structure which, for some reason, does not come standard in
programming languages: a dictionary that has numerical values (and arbitrary keys).
A number is a number. An array, or vector, is a list of numbers that can be indexed by their position in the vector. An arithmetic dictionary is a set
of numbers that can be addressed by their associated key. Just as numbers, and arrays, can be combined via +, -, *, 7, and compared with >, <,
etc, so can arithmetic dictionaries. Let us start defining them. For brevity, we call them simply AD rather than ArithmeticDictionary. We note that
Python includes, in the collections module, the counter class, which supports addition. ADs are a more general version of the counter class,
as they support (or you can make them to support) all math operations you wish.
In Python, to be able to have two ADs d1 and d2 and compute their sum via the syntax d1 + d2, we need to define the _add_ operation, and
similarly for the other arithmetic operations.
There is a fine point to be discussed. Arrays can be combined with arithmetical operations only when they are of the same dimensions, or when
one can be "broadcast" to fit the dimension of the other. For dictionaries, however, the restriction to be able to combine only arithmetic
dictionaries with the same keys would be too restrictive. If we have an AD d representing a sock drawer, and we want to add to it one yellow
and two blue socks, we want to be able to write something like:
d += AD({'yellow': 1, 'blue': 2})
rather than worry that d might have different keys from {yellow', 'blue'}. Thus, in the definition of an operation, we will need to define suitable
defaults for the missing values. In general, the suitable defaults are the neutral elements with respect to the operation: 0 for+ and –, and 1 for
x and /.
Let us begin from addition and subtraction.
[] class AD(dict):
def _init_(self, *args, **kwargs):
"*"This initializer simply passes all arguments to dict, so that
we can create an AD with the same ease with which we can create
a dict.
There is no need, indeed, to repeat the initializer,
but we leave it here in case we like to create attributes specific
of an AD later."""
super ()._init_(*args, **kwargs)
def _add_(self, other):
return AD._binary_op(self, other, lambda x, y: x + y, e)
def _sub_(self, other):
return AD._binary_op(self, other, lambda x, y: x - y, e)
@staticmethod
def _binary_op(left, right, op, neutral):
r- AD()
1_keys = set(left.keys()) if isinstance(left, dict) else set()
r_keys - set(right.keys()) if isinstance(right, dict) else set()
for k in 1_keys | r_keys:
# If the right (or left) element is a dictionary (or an AD),
# we get the elements from the dictionary; else we use the right
# or left value itself. This implements a sort of dictionary
# broadcasting.
1_val - left.get(k, neutral) if isinstance(left, dict) else left
r_val = right.get (k, neutral) if isinstance(right, dict) else right
r[k] = op(1_val, r_val)
return r
![We let multiplication and division to you to implement. You need to implement the operators:
_mul_
_truediv_
_floordiv_
[] ### Question 1: Implement multiplication and division
# Define _mul
def ad_mul(self, other):
# YOUR CODE HERE
AD._mul_
ad_mul
# Define below _truediv_, similarly.
# YOUR CODE HERE
# And finally, define below _floordiv_, for INTEGER division.
# YOUR CODE HERE
We will let you also implement max_items and min_items properties. The value of these properties should be a pair, consisting of the
maximum/minimum value, and of the set of keys that led to that value. The latter needs to be a set, because there are multiple key, value pairs
where the value is maximal or minimal. To avoid wasting your time, we will have you implement only the max_items property. If the AD is empty,
the property's value should be the pair (None, set()).
) *** Question 2: Implement "max_items
def ad_max_items (self):
"*"Returns a pair consisting of the max value in the AD, and of the
set of keys that attain that value. This can be done in 7 lines of code."""
# YOUR CODE HERE
* Remember the use of the property decorator.
AD. max_items - property(ad_max_items)
[] *** Tests for `max_items"
* For empty ADs, it has to return None as value, and the empty set as key set.
check_equal (AD(). max_items, (None, set()))
# Case of one maximum.
check_equal (AD(red-2, green-3, blue-1).max_items, (3, {'green'}))
* Case of multiple maxima.
check_equal(AD(red-2, yellow-3, blue-3, violet-3, pink-1).max_items,
(3, {'yellow", "blue", "violet'}))](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F293032ce-89a7-4d5a-8a29-aae96cb1d151%2F63ec4d9f-1188-4c63-b9b9-3c7a4ac39c76%2F099hzu_processed.png&w=3840&q=75)
Transcribed Image Text:We let multiplication and division to you to implement. You need to implement the operators:
_mul_
_truediv_
_floordiv_
[] ### Question 1: Implement multiplication and division
# Define _mul
def ad_mul(self, other):
# YOUR CODE HERE
AD._mul_
ad_mul
# Define below _truediv_, similarly.
# YOUR CODE HERE
# And finally, define below _floordiv_, for INTEGER division.
# YOUR CODE HERE
We will let you also implement max_items and min_items properties. The value of these properties should be a pair, consisting of the
maximum/minimum value, and of the set of keys that led to that value. The latter needs to be a set, because there are multiple key, value pairs
where the value is maximal or minimal. To avoid wasting your time, we will have you implement only the max_items property. If the AD is empty,
the property's value should be the pair (None, set()).
) *** Question 2: Implement "max_items
def ad_max_items (self):
"*"Returns a pair consisting of the max value in the AD, and of the
set of keys that attain that value. This can be done in 7 lines of code."""
# YOUR CODE HERE
* Remember the use of the property decorator.
AD. max_items - property(ad_max_items)
[] *** Tests for `max_items"
* For empty ADs, it has to return None as value, and the empty set as key set.
check_equal (AD(). max_items, (None, set()))
# Case of one maximum.
check_equal (AD(red-2, green-3, blue-1).max_items, (3, {'green'}))
* Case of multiple maxima.
check_equal(AD(red-2, yellow-3, blue-3, violet-3, pink-1).max_items,
(3, {'yellow", "blue", "violet'}))
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
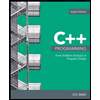
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
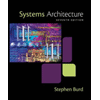
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
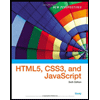
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning
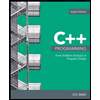
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
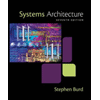
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
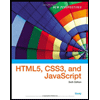
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning