2. Partition modulo n Background Refer to the problem "Partition modulo three" for a discussion on partitioning a set into equivalence classes defined on a relation. Challenge Write a function called partition_modulo_n that accepts an integer n and a list of integers t as its arguments, and returns a dictionary that partitions the list. Each possible remainder, from 0 to n - 1, is a key in the dictionary, with its associated value being a list containing elements from the argument list t that leave that remainder. The function must leave the argument list t unmodified, i.e., partition_modulo_n has no side-effects. Note The dictionary must contain a list value for each possible remainder, even if no elements from the argument list t have that remainder, i.e., if no elements have a given remainder then the list value associated with that remainder key will be an empty list. Elements in the dictionary values must appear in the same order as in the argument list t. n may be negative, in which case the remainders are non-positive by convention.
2. Partition modulo n Background Refer to the problem "Partition modulo three" for a discussion on partitioning a set into equivalence classes defined on a relation. Challenge Write a function called partition_modulo_n that accepts an integer n and a list of integers t as its arguments, and returns a dictionary that partitions the list. Each possible remainder, from 0 to n - 1, is a key in the dictionary, with its associated value being a list containing elements from the argument list t that leave that remainder. The function must leave the argument list t unmodified, i.e., partition_modulo_n has no side-effects. Note The dictionary must contain a list value for each possible remainder, even if no elements from the argument list t have that remainder, i.e., if no elements have a given remainder then the list value associated with that remainder key will be an empty list. Elements in the dictionary values must appear in the same order as in the argument list t. n may be negative, in which case the remainders are non-positive by convention.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 2PE
Related questions
Question
Language is python 3
![Sample
>>> partition_modulo_n(3, [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11])
{0: [0, 3, 6, 9], 1: [1, 4, 7, 10], 2: [2, 5, 8, 11]}
>>> partition_modulo_n(3, [-6, -5, -4, -3, -2, -1, 0, l, 2, 3, 4,
5])
{0: [-6, -3, 0, 3], 1: [-5, -2, 1, 4], 2: [-4, -1, 2, 5]}
>> partition_modulo_n(3, [17, 20, -16, 34, 28, 47, -49, 37, -43,
-19, -8, 19]
{0: [], 1: [34, 28, 37, -8, 19], 2: [17, 20, -16, 47, -49, -43,
-19]}
>>> partition_modulo_n(4, [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11])
{0: [0, 4, 8], 1: [1, 5, 9], 2: [2, 6, 10], 3: [3, 7, 11]}
>>> partition_modulo_n(4, [-6, -5, -4, -3, -2, -1, 0, l, 2, 3, 4,
5]))
{0: [-4, 0, 4], 1: [-3, 1, 5], 2: [-6, -2, 2], 3: [-5, -1, 3]}
>> partition_modulo_n(4, [17, 20, -16, 34, 28, 47, -49, 37, -43,
-19, -8, 19])
{0: [20, -16, 28, -8], 1: [17, 37, -43, -19], 2: [34], 3: [47, -49,
19]}
>>> partition_modulo_n(-4, [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11])
{-3: [1, 5, 9], -2: [2, 6, 10], -1: [3, 7, 11], 0: [0, 4, 8]}
>>> partition_modulo_n(-4, [-6, -5, -4, -3, -2, -1, 0, 1, 2, 3, 4,
5])
{-3: [-3, 1, 5], -2: [-6, -2, 2], -1: [-5, -1, 3], 0: [-4, 0, 4]}
>>> partition_modulo_n(-4, [17, 20, -16, 34, 28, 47, -49, 37, -43,
-19, -8, 19])
{-3: [17, 37, -43, -19], -2: [34], -1: [47, -49, 19], 0: [20, -16,
28, -8]}
Input/Output
Input consists of a single integer by itself on the first line to represent n, and zero or
more space-separated integers on the second line to represent integers in list t.
HackerRank will read in the integers and pass the arguments to your function, and
then output the dictionary, with the keys sorted in a natural order. Dictionary values,
however, will not be sorted.
Constraints
isinstance (n, int) and all(isinstance(i, int) for i in t) and n !=
o is True.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F84d04b2c-23fb-40d7-9a76-0652257fb987%2F1c12327d-8da1-43ea-aed1-5ac71849030e%2Fphw0jb_processed.png&w=3840&q=75)
Transcribed Image Text:Sample
>>> partition_modulo_n(3, [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11])
{0: [0, 3, 6, 9], 1: [1, 4, 7, 10], 2: [2, 5, 8, 11]}
>>> partition_modulo_n(3, [-6, -5, -4, -3, -2, -1, 0, l, 2, 3, 4,
5])
{0: [-6, -3, 0, 3], 1: [-5, -2, 1, 4], 2: [-4, -1, 2, 5]}
>> partition_modulo_n(3, [17, 20, -16, 34, 28, 47, -49, 37, -43,
-19, -8, 19]
{0: [], 1: [34, 28, 37, -8, 19], 2: [17, 20, -16, 47, -49, -43,
-19]}
>>> partition_modulo_n(4, [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11])
{0: [0, 4, 8], 1: [1, 5, 9], 2: [2, 6, 10], 3: [3, 7, 11]}
>>> partition_modulo_n(4, [-6, -5, -4, -3, -2, -1, 0, l, 2, 3, 4,
5]))
{0: [-4, 0, 4], 1: [-3, 1, 5], 2: [-6, -2, 2], 3: [-5, -1, 3]}
>> partition_modulo_n(4, [17, 20, -16, 34, 28, 47, -49, 37, -43,
-19, -8, 19])
{0: [20, -16, 28, -8], 1: [17, 37, -43, -19], 2: [34], 3: [47, -49,
19]}
>>> partition_modulo_n(-4, [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11])
{-3: [1, 5, 9], -2: [2, 6, 10], -1: [3, 7, 11], 0: [0, 4, 8]}
>>> partition_modulo_n(-4, [-6, -5, -4, -3, -2, -1, 0, 1, 2, 3, 4,
5])
{-3: [-3, 1, 5], -2: [-6, -2, 2], -1: [-5, -1, 3], 0: [-4, 0, 4]}
>>> partition_modulo_n(-4, [17, 20, -16, 34, 28, 47, -49, 37, -43,
-19, -8, 19])
{-3: [17, 37, -43, -19], -2: [34], -1: [47, -49, 19], 0: [20, -16,
28, -8]}
Input/Output
Input consists of a single integer by itself on the first line to represent n, and zero or
more space-separated integers on the second line to represent integers in list t.
HackerRank will read in the integers and pass the arguments to your function, and
then output the dictionary, with the keys sorted in a natural order. Dictionary values,
however, will not be sorted.
Constraints
isinstance (n, int) and all(isinstance(i, int) for i in t) and n !=
o is True.
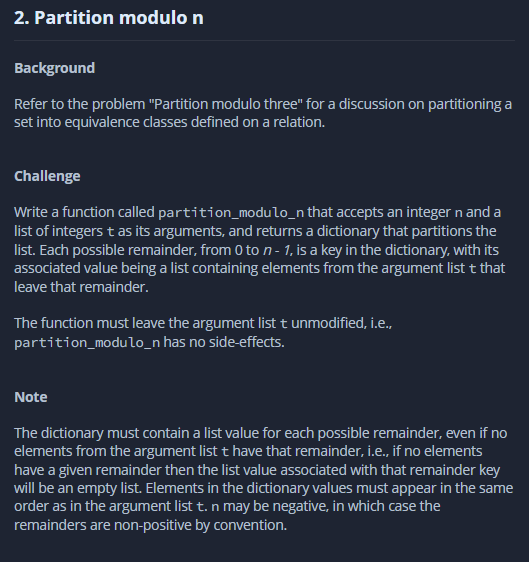
Transcribed Image Text:2. Partition modulo n
Background
Refer to the problem "Partition modulo three" for a discussion on partitioning a
set into equivalence classes defined on a relation.
Challenge
Write a function called partition_modulo_n that accepts an integer n and a
list of integers t as its arguments, and returns a dictionary that partitions the
list. Each possible remainder, from o to n - 1, is a key in the dictionary, with its
associated value being a list containing elements from the argument list t that
leave that remainder.
The function must leave the argument list t unmodified, i.e.,
partition_modulo_n has no side-effects.
Note
The dictionary must contain a list value for each possible remainder, even if no
elements from the argument list t have that remainder, i.e., if no elements
have a given remainder then the list value associated with that remainder key
will be an empty list. Elements in the dictionary values must appear in the same
order as in the argument list t. n may be negative, in which case the
remainders are non-positive by convention.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
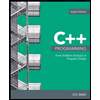
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
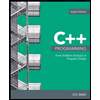
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning