Assignment Write a program that calculates the revenue of a trip of a train composed of several train-cars. For the train, the user will give the total allowed train- car count (int), total allowed weight (double), and base speed (double). Then, the user will give train-car information for ALL train-cars that will TRY TO BE ADDED to the train. First, the number of train-cars will be given (int). For each train-car, three information will be given: type of the cargo (std::string), weight of the train-car (double), and how full the train-car is (int). After getting all this information, the program will TRY TO ADD each train-car to the train according to the train-car count and total allowed weight values. You CANNOT ADD a train-car to a train if it exceeds its train-car count. You CANNOT ADD a train-car to a train if by adding this train-car, the total weight of the train exceeds the train's total allowed weight. Each added train-car reduces the speed of the train by half its weight. Finally, the program will get a distance (int) and a deadline (double) value, calculate the revenue of this trip and prints it out to the screen. The revenue of a trip is the summation of the revenues of each train-car ADDED to the train affected by the deadline. If the duration of the trip is longer than the deadline there will be a 40% penalty. Requirements:
Assignment Write a program that calculates the revenue of a trip of a train composed of several train-cars. For the train, the user will give the total allowed train- car count (int), total allowed weight (double), and base speed (double). Then, the user will give train-car information for ALL train-cars that will TRY TO BE ADDED to the train. First, the number of train-cars will be given (int). For each train-car, three information will be given: type of the cargo (std::string), weight of the train-car (double), and how full the train-car is (int). After getting all this information, the program will TRY TO ADD each train-car to the train according to the train-car count and total allowed weight values. You CANNOT ADD a train-car to a train if it exceeds its train-car count. You CANNOT ADD a train-car to a train if by adding this train-car, the total weight of the train exceeds the train's total allowed weight. Each added train-car reduces the speed of the train by half its weight. Finally, the program will get a distance (int) and a deadline (double) value, calculate the revenue of this trip and prints it out to the screen. The revenue of a trip is the summation of the revenues of each train-car ADDED to the train affected by the deadline. If the duration of the trip is longer than the deadline there will be a 40% penalty. Requirements:
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
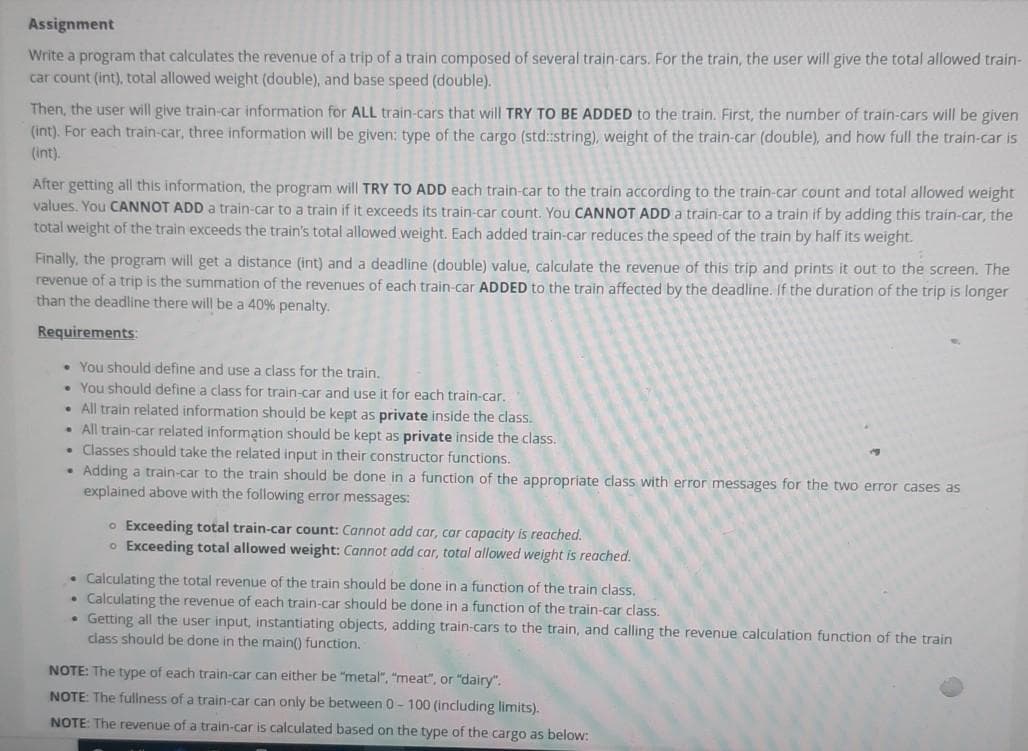
Transcribed Image Text:Assignment
Write a program that calculates the revenue of a trip of a train composed of several train-cars. For the train, the user will give the total allowed train-
car count (int), total allowed weight (double), and base speed (double).
Then, the user will give train-car information for ALL train-cars that will TRY TO BE ADDED to the train. First, the number of train-cars will be given
(int). For each train-car, three information will be given: type of the cargo (std:string), weight of the train-car (double), and how full the train-car is
(int).
After getting all this information, the program will TRY TO ADD each train-car to the train according to the train-car count and total allowed weight
values. You CANNOT ADD a train-car to a train if it exceeds its train-car count. You CANNOT ADD a train-car to a train if by adding this train-car, the
total weight of the train exceeds the train's total allowed weight. Each added train-car reduces the speed of the train by half its weight.
Finally, the program will get a distance (int) and a deadline (double) value, calculate the revenue of this trip and prints it out to the screen. The
revenue of a trip is the summation of the revenues of each train-car ADDED to the train affected by the deadline. If the duration of the trip is longer
than the deadline there will be a 40% penalty.
Requirements:
• You should define and use a class for the train.
• You should define a class for train-car and use it for each train-car.
• All train related information should be kept as private inside the class.
• All train-car related information should be kept as private inside the class.
• Classes should take the related input in their constructor functions.
• Adding a train-car to the train should be done in a function of the appropriate class with error messages for the two error cases as
explained above with the following error messages:
o Exceeding total train-car count: Cannot add car, car capacity is reached.
o Exceeding total allowed weight: Cannot add car, total allowed weight is reached.
Calculating the total revenue of the train should be done in a function of the train class.
• Calculating the revenue of each train-car should be done in a function of the train-car class.
Getting all the user input, instantiating objects, adding train-cars to the train, and calling the revenue calculation function of the train
class should be done in the main() function.
NOTE: The type of each train-car can either be "metal", "meat", or "dairy".
NOTE: The fullness of a train-car can only be between 0- 100 (including limits).
NOTE: The revenue of a train-car is calculated based on the type of the cargo as below:
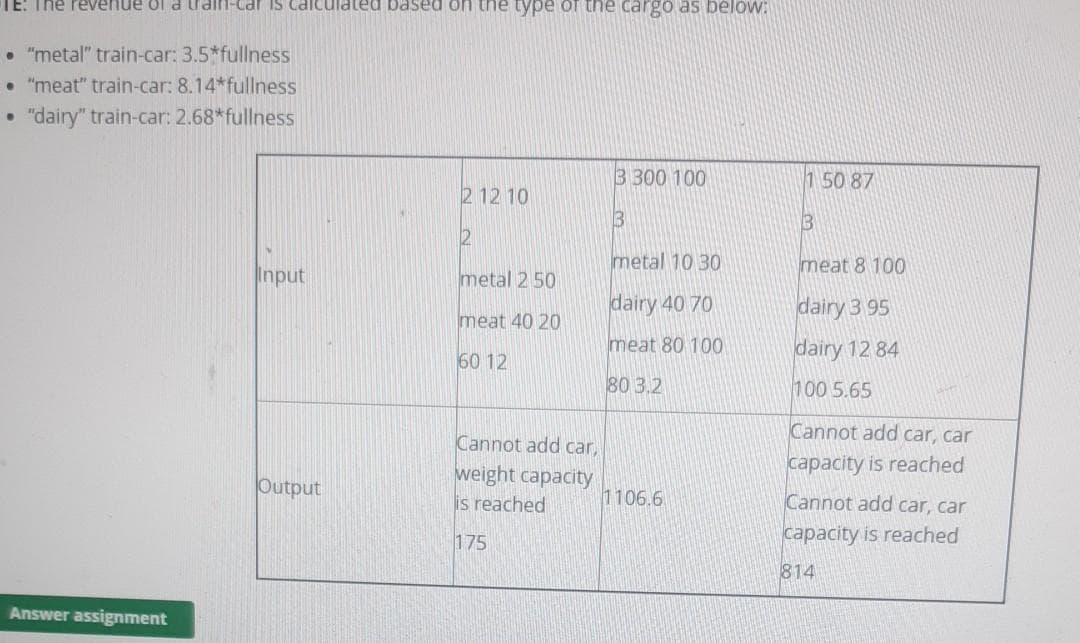
Transcribed Image Text:TE: The revenue ol a trdin-car is caIculated based on the type of the cargo as below:
• "metal" train-car: 3.5*fullness
• "meat" train-car: 8.14*fullness
"dairy" train-car: 2.68*fullness
3 300 100
1 50 87
2 12 10
13
metal 10 30
meat 8 100
Input
metal 2 50
dairy 40 70
dairy 3 95
meat 40 20
meat 80 100
dairy 12 84
60 12
80 3.2
100 5.65
Cannot add car, car
Cannot add car,
weight capacity
capacity is reached
Output
reached
1106.6
Cannot add car, car
175
capacity is reached
814
Answer assignment
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 6 steps with 4 images

Recommended textbooks for you
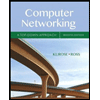
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
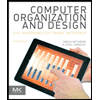
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
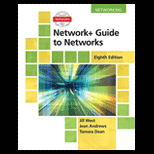
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
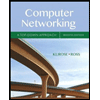
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
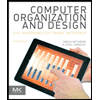
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
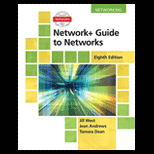
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
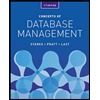
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
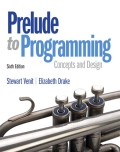
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
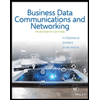
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY