Language: JAVA Leap-Year Write a program to find if a year is a leap year or not. We generally assume that if a year number is divisible by 4 it is a leap year. But it is not the only case. A year is a leap year if − It is evenly divisible by 100 If it is divisible by 100, then it should also be divisible by 400 Except this, all other years evenly divisible by 4 are leap years. So the leap year algorithm is, given the year number Y, Check if Y is divisible by 4 but not 100, DISPLAY "leap year" Check if Y is divisible by 400, DISPLAY "leap year" Otherwise, DISPLAY "not leap year" For this program you have to take the input, that is the year number from an input file input.txt that is provided to you. The input file contains multiple input year numbers. Use a while loop to input the year numbers from the input file one at a time and check if that year is a leap year or not. Create a class named LeapYear which will contain the main method and write all your code in the main method. Copy and paste the given input.txt file outside of the src (in which you have your LeapYear.java file) folder in the project folder. Sample input and output: Sample input file (input.txt) content: 2010 2011 2012 2013 2014 2015 2016 2017 2018 2019 2020 Sample output: 2010 is not a leap year 2011 is not a leap year 2012 is a leap year 2013 is not a leap year 2014 is not a leap year 2015 is not a leap year 2016 is a leap year 2017 is not a leap year 2018 is not a leap year 2019 is not a leap year 2020 is a leap year
Language: JAVA
- Leap-Year
Write a program to find if a year is a leap year or not. We generally assume that if a year number is divisible by 4 it is a leap year. But it is not the only case. A year is a leap year if −
- It is evenly divisible by 100
- If it is divisible by 100, then it should also be divisible by 400
- Except this, all other years evenly divisible by 4 are leap years.
So the leap year
- Check if Y is divisible by 4 but not 100, DISPLAY "leap year"
- Check if Y is divisible by 400, DISPLAY "leap year"
- Otherwise, DISPLAY "not leap year"
For this program you have to take the input, that is the year number from an input file input.txt that is provided to you. The input file contains multiple input year numbers. Use a while loop to input the year numbers from the input file one at a time and check if that year is a leap year or not.
Create a class named LeapYear which will contain the main method and write all your code in the main method. Copy and paste the given input.txt file outside of the src (in which you have your LeapYear.java file) folder in the project folder.
Sample input and output:
Sample input file (input.txt) content:
2010
2011
2012
2013
2014
2015
2016
2017
2018
2019
2020
Sample output:
2010 is not a leap year
2011 is not a leap year
2012 is a leap year
2013 is not a leap year
2014 is not a leap year
2015 is not a leap year
2016 is a leap year
2017 is not a leap year
2018 is not a leap year
2019 is not a leap year
2020 is a leap year

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

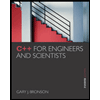
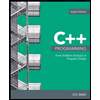
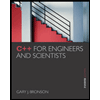
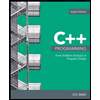