Assignment You work at the Computer Science 201L Library, and your boss just bought a bunch of new books for the library! All of the new books need to be cataloged and sorted back on the shelf. You don’t need to keep track of what customer has the book or not, just whether they are checked in or out. Objects You need to create a class called LibraryBook. It needs to have the following member variables, stored privately: string title, string author, and string ISBN. You also need to have a Boolean to store its status whether it’s checked out or not. Call this variable checkedOut. Create a header file and a .cpp file for the LibraryBook class. Call it LibraryBook.cpp and LibraryBook.h For functions, this library book class needs to have: A default constructor setting all strings blank and the checked out status to false A constructor setting all strings through the parameters (title, author, ISBN), and setting the checked out status to false. “Getters” to get the values from the private variables. (So getTitle(), getAuthor(), getISBN()) A checkOut() function to change the status so it is checked out A checkIn() function to change the status so it is checked in (or NOT checked out) A isCheckedOut() function to return the status of the book More Information and File Structure The books.txt file has a list of all of the books at the library. The books are listed with the title on one line, the author on the next, then the ISBN on the next. For simplicity, all three are stored as strings in the class, including the number. Using the file streams, you’ve learned how to read in one word or number at a time. To read in an entire line, use the getline() function. For example: string str; // string variable ifstream fin(“file.txt”); // input file stream getline(fin, str); // read one line, store in variable This will read in the while line (not the first word) from the file and store it in the variable str, which is a string. Use this example to read in the title, author, and ISBN from the file. From there, you can pass in your data in as parameters into the LibraryBook object. For example: string title = “title”; string author = “author”; string ISBN = “123”; LibraryBook myBook(title, author, ISBN); STOP HERE! Make sure your program works correctly at this point before continuing. Next, in the isbns.txt file, you have a list of ISBN numbers, one per line. If the ISBN number appears in this file, it has passed through the barcode scanner on the computer to be checked in or out. You can read these in with the normal style you are used to (for example: string theISBN; fin >> theISBN; ). You can then either use the LibraryBook’s checkIn() or checkOut() function to tag it as either there or not there. To determine which one to use, call the isCheckedOut() function to find out if it’s at the library or not, then use that Boolean to determine which function you call. STOP HERE! Make sure your program works correctly (again) at this point before continuing. Finally, you need to print the report your boss needs. The report should be named checkedout.txt and should print out a header consisting of the words “Title”, “Author”, and “ISBN”, separated by tabs, and on each line after that, the title, author, and ISBN of the books that are checked out, separated by tabs. You will need to do another loop to iterate through the array, and print out books that are checked out. For the files provided, your checkedout.txt file should look like: (Screenshot) =================== Books.txt =================== Nine Algorithms That Changed the Future John MacCormick 1691147140 Code: The Hidden Language of Computer Hardware and Software Charles Petzold 1735611319 Introduction to Algorithms Thomas H. Cormen 1262033844 The Science of Programming David Gries 1387964800 Interconnection Networks Jose Duato 1558608524 Computer System Architecture M. Morris Mano 1131755633 Understanding Computers: Today & Tomorrow, Comprehensive Deborah Morley 1423925211 Logic and Computer Design Fundamentals M. Morris Mano 1131678493 Methods in Algorithmic Analysis Vladimir A. Dobrushkin 1420068296 Software Engineering: An Engineering Approach James F. Peters 1471189642 ========================================= isbns.txt ========================================= 1558608524 1131678493 1735611319 1471189642 1387964800 1691147140 1558608524 1131678493 1420068296 1735611319 1131755633 1387964800 1735611319 1420068296 1387964800 1691147140 1420068296 1471189642 1420068296 1131678493 1735611319 1387964800 1423925211 1131755633 1131755633 1131755633 1735611319 1558608524
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Assignment
You work at the Computer Science 201L Library, and your boss just bought a bunch of new books for the library! All of the new books need to be cataloged and sorted back on the shelf.
You don’t need to keep track of what customer has the book or not, just whether they are checked in or out.
Objects
You need to create a class called LibraryBook. It needs to have the following member variables, stored privately: string title, string author, and string ISBN. You also need to have a Boolean to store its status whether it’s checked out or not. Call this variable checkedOut.
Create a header file and a .cpp file for the LibraryBook class. Call it LibraryBook.cpp and LibraryBook.h
For functions, this library book class needs to have:
- A default constructor setting all strings blank and the checked out status to false
- A constructor setting all strings through the parameters (title, author, ISBN), and setting the checked out status to false.
- “Getters” to get the values from the private variables. (So getTitle(), getAuthor(), getISBN())
- A checkOut() function to change the status so it is checked out
- A checkIn() function to change the status so it is checked in (or NOT checked out)
- A isCheckedOut() function to return the status of the book
More Information and File Structure
The books.txt file has a list of all of the books at the library. The books are listed with the title on one line, the author on the next, then the ISBN on the next. For simplicity, all three are stored as strings in the class, including the number. Using the file streams, you’ve learned how to read in one word or number at a time. To read in an entire line, use the getline() function. For example:
string str; // string variable
ifstream fin(“file.txt”); // input file stream
getline(fin, str); // read one line, store in variable
This will read in the while line (not the first word) from the file and store it in the variable str, which is a string. Use this example to read in the title, author, and ISBN from the file.
From there, you can pass in your data in as parameters into the LibraryBook object. For example:
string title = “title”;
string author = “author”;
string ISBN = “123”;
LibraryBook myBook(title, author, ISBN);
STOP HERE! Make sure your
Next, in the isbns.txt file, you have a list of ISBN numbers, one per line. If the ISBN number appears in this file, it has passed through the barcode scanner on the computer to be checked in or out. You can read these in with the normal style you are used to (for example: string theISBN; fin >> theISBN; ). You can then either use the LibraryBook’s checkIn() or checkOut() function to tag it as either there or not there. To determine which one to use, call the isCheckedOut() function to find out if it’s at the library or not, then use that Boolean to determine which function you call.
STOP HERE! Make sure your program works correctly (again) at this point before continuing.
Finally, you need to print the report your boss needs. The report should be named checkedout.txt and should print out a header consisting of the words “Title”, “Author”, and “ISBN”, separated by tabs, and on each line after that, the title, author, and ISBN of the books that are checked out, separated by tabs. You will need to do another loop to iterate through the array, and print out books that are checked out. For the files provided, your checkedout.txt file should look like: (Screenshot)
===================
Books.txt
===================
Nine
John MacCormick
1691147140
Code: The Hidden Language of Computer Hardware and Software
Charles Petzold
1735611319
Introduction to Algorithms
Thomas H. Cormen
1262033844
The Science of Programming
David Gries
1387964800
Interconnection Networks
Jose Duato
1558608524
Computer System Architecture
M. Morris Mano
1131755633
Understanding Computers: Today & Tomorrow, Comprehensive
Deborah Morley
1423925211
Logic and Computer Design Fundamentals
M. Morris Mano
1131678493
Methods in Algorithmic Analysis
Vladimir A. Dobrushkin
1420068296
Software Engineering: An Engineering Approach
James F. Peters
1471189642
=========================================
isbns.txt
=========================================
1558608524
1131678493
1735611319
1471189642
1387964800
1691147140
1558608524
1131678493
1420068296
1735611319
1131755633
1387964800
1735611319
1420068296
1387964800
1691147140
1420068296
1471189642
1420068296
1131678493
1735611319
1387964800
1423925211
1131755633
1131755633
1131755633
1735611319
1558608524
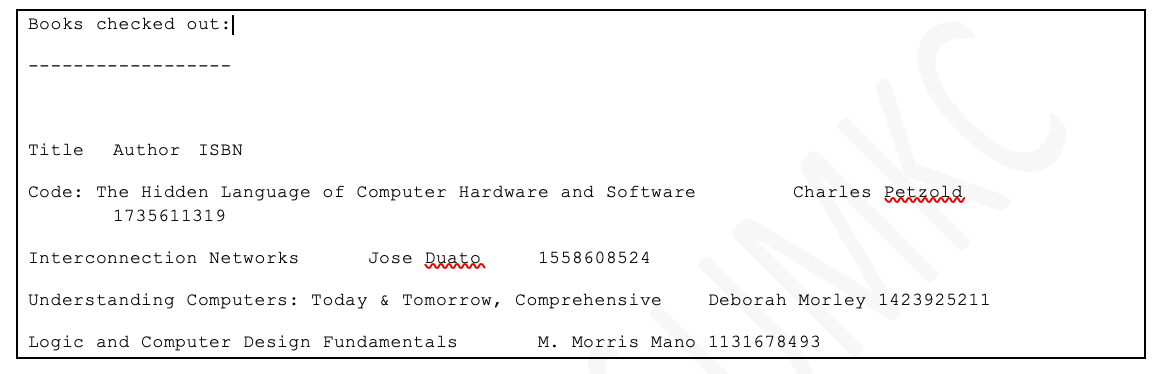

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

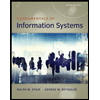
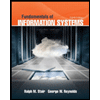
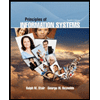
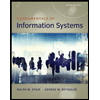
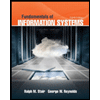
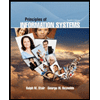
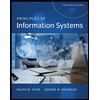