Assignment For this project, you get to design and write a stock class to keep track of the profit of stock purchases. You also get to design and write stockDriver class that requests input from the user and interacts with the stock class. Your stock class should store the following information: • Total number of shares purchased • Total cost basis (defined above) Provide the following methods in your stock class: • No-argument constructor that initializes both the total number of shares purchased and the total cost basis • addstockPurchase () that takes the number of shares purchased (as an int) and the price per share (as a double). This method should add the newly purchased shares to the total number of shares purchased and update the cost basis (with the number of shared purchased multiplied by the price per share). • getNumberofShares () returns the total number of shares purchased as an int • getCostBasis () returns the total cost basis as a double, rounded to 2 decimals because it is money • calculateProfit () that takes the price per share (at the time of the sale) (as a double) and returns the profit of selling all of the shares (as a double, rounded to 2 decimals because it is money) Your StockDriver class should create a stock object to keep track of the user's input. First, it requests the number of stock purchases from the user. For each purchase, it prompts the user for the number of shares purchased (a positive number greater than 0)., then the price per share (a positive number greater than 0.0). While either of these inputs are negative or equal to o (or 0.0), it prompts the user again before proceeding with the next input. Note, if the user provided an invalid number of shares purchased, the program repeatedly requests valid input before requesting the price per share. After all purchases are inputted, the user is prompted for the the price per share at the time of sale (a positive number greater than 0.0). Again, if an invalid number is entered, the program should repeatedly request valid input. Note, do not keep track of the cost basis in your stockDriver class. Instead, make the appropriate method calls using the stock object. Each of the methods in your stock class will be tested using unit tests. Additionally, the output of your entire program will be tested with various inputs. . Examples (user input in bold text) Example 1 (with invalid input) Welcome to the Stocks Profit Calculator! Please enter the number of stock puchases: 3 Please enter the number of shares purchased: -99 Please enter the number of shares purchased: 0 Please enter the number of shares purchased: 100 Please enter the price per share: 0.0 Please enter the price per share: -98.76 Please enter the price per share: 5.5 Please enter the number of shares purchased: -100 Please enter the number of shares purchased: 100 Please enter the price per share: 5.15 Please enter the number of shares purchased: 100 Please enter the price per share: 6.16 The cost basis is $1681.0. To calculate the profit, please enter the price per share of a sale: -6.25 To calculate the profit, please enter the price per share of a sale: 6.25 The profit from selling 300 shares at 6.25 each is $194.e. Notice that multiple invalid values are entered and that the program keeps asking for a valid values until it gets a valid one. Also notice that the cost basis and profit are each rounded. If you're trying to debug this example (and I hope that you would), you could temporarily display the cost basis after each valid price per purchased share is entered. That should give you the following values: The current cost basis is $550.0. The current cost basis is $1065.0. The current cost basis is $1681.e.
Assignment For this project, you get to design and write a stock class to keep track of the profit of stock purchases. You also get to design and write stockDriver class that requests input from the user and interacts with the stock class. Your stock class should store the following information: • Total number of shares purchased • Total cost basis (defined above) Provide the following methods in your stock class: • No-argument constructor that initializes both the total number of shares purchased and the total cost basis • addstockPurchase () that takes the number of shares purchased (as an int) and the price per share (as a double). This method should add the newly purchased shares to the total number of shares purchased and update the cost basis (with the number of shared purchased multiplied by the price per share). • getNumberofShares () returns the total number of shares purchased as an int • getCostBasis () returns the total cost basis as a double, rounded to 2 decimals because it is money • calculateProfit () that takes the price per share (at the time of the sale) (as a double) and returns the profit of selling all of the shares (as a double, rounded to 2 decimals because it is money) Your StockDriver class should create a stock object to keep track of the user's input. First, it requests the number of stock purchases from the user. For each purchase, it prompts the user for the number of shares purchased (a positive number greater than 0)., then the price per share (a positive number greater than 0.0). While either of these inputs are negative or equal to o (or 0.0), it prompts the user again before proceeding with the next input. Note, if the user provided an invalid number of shares purchased, the program repeatedly requests valid input before requesting the price per share. After all purchases are inputted, the user is prompted for the the price per share at the time of sale (a positive number greater than 0.0). Again, if an invalid number is entered, the program should repeatedly request valid input. Note, do not keep track of the cost basis in your stockDriver class. Instead, make the appropriate method calls using the stock object. Each of the methods in your stock class will be tested using unit tests. Additionally, the output of your entire program will be tested with various inputs. . Examples (user input in bold text) Example 1 (with invalid input) Welcome to the Stocks Profit Calculator! Please enter the number of stock puchases: 3 Please enter the number of shares purchased: -99 Please enter the number of shares purchased: 0 Please enter the number of shares purchased: 100 Please enter the price per share: 0.0 Please enter the price per share: -98.76 Please enter the price per share: 5.5 Please enter the number of shares purchased: -100 Please enter the number of shares purchased: 100 Please enter the price per share: 5.15 Please enter the number of shares purchased: 100 Please enter the price per share: 6.16 The cost basis is $1681.0. To calculate the profit, please enter the price per share of a sale: -6.25 To calculate the profit, please enter the price per share of a sale: 6.25 The profit from selling 300 shares at 6.25 each is $194.e. Notice that multiple invalid values are entered and that the program keeps asking for a valid values until it gets a valid one. Also notice that the cost basis and profit are each rounded. If you're trying to debug this example (and I hope that you would), you could temporarily display the cost basis after each valid price per purchased share is entered. That should give you the following values: The current cost basis is $550.0. The current cost basis is $1065.0. The current cost basis is $1681.e.
Chapter1: Creating Java Programs
Section: Chapter Questions
Problem 12PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
100%
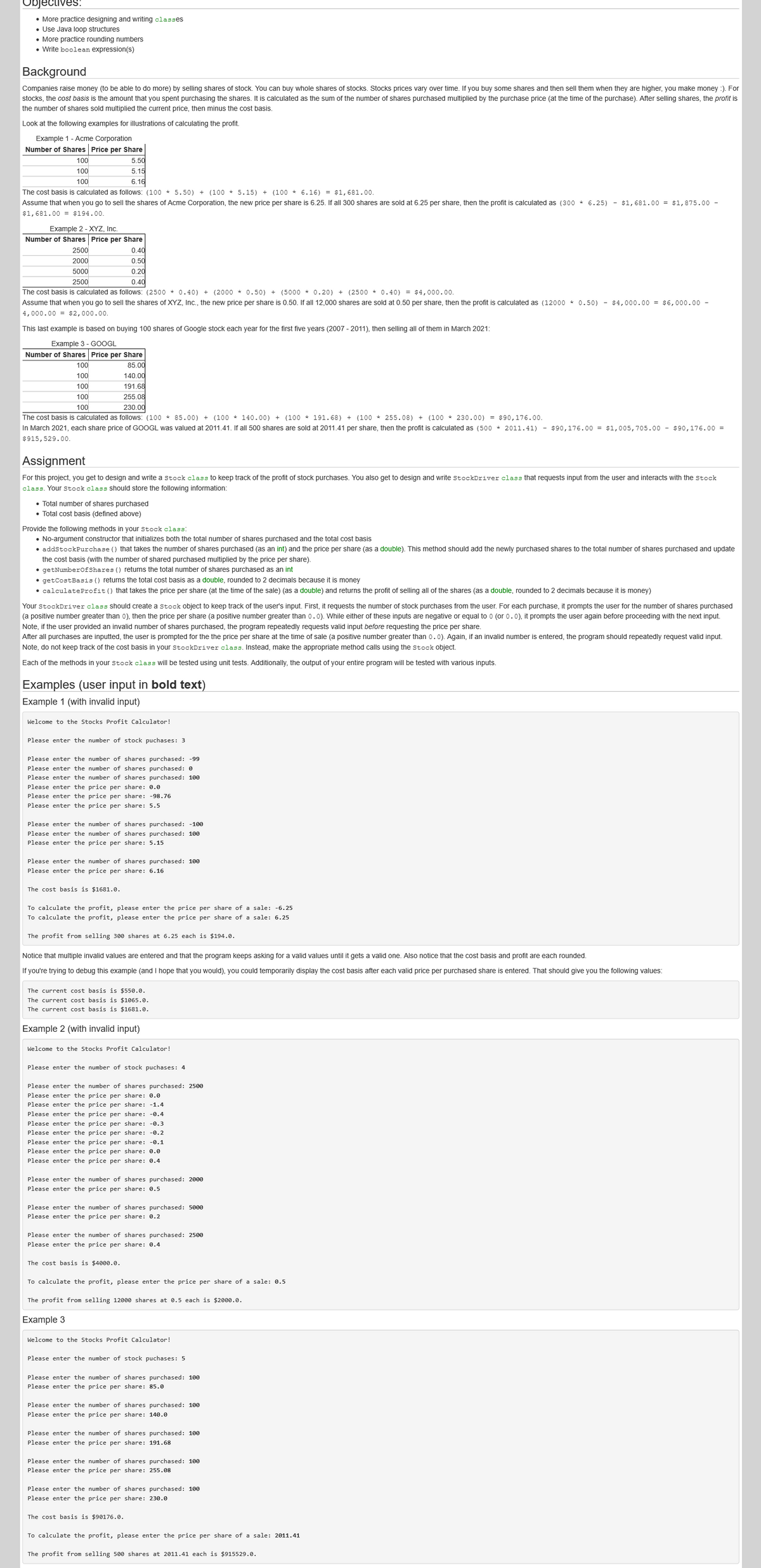
Transcribed Image Text:Objectives:
• More practice designing and writing classes
• Use Java loop structures
• More practice rounding numbers
• Write boolean expression(s)
Background
Companies raise money (to be able to do more) by selling shares of stock. You can buy whole shares of stocks. Stocks prices vary over time. If you buy some shares and then sell them when they are higher, you make money :). For
stocks, the cost basis is the amount that you spent purchasing the shares. It is calculated as the sum of the number of shares purchased multiplied by the purchase price (at the time of the purchase). After selling shares, the profit is
the number of shares sold multiplied the current price, then minus the cost basis.
Look at the following examples for illustrations of calculating the profit.
Example 1 - Acme Corporation
Number of Shares Price per Share
5.50
5.15
100
100
100
6.16
The cost basis is calculated as follows: (100 * 5.50) + (100 * 5.15) + (100 * 6.16) = $1,681.00.
Assume that when you go to sell the shares of Acme Corporation, the new price per share is 6.25. If all 300 shares are sold at 6.25 per share, then the profit is calculated as (300 * 6.25) - $1,681.00 = $1,875.00 -
$1,681.00 = $194.00.
Example 2 - XYZ, Inc.
Number of Shares Price per Share
2500
0.40
0.50
0.20
2000
5000
2500
0.40
The cost basis is calculated as follows: (2500 * 0.40) + (2000 * 0.50) + (5000 * 0.20) + (2500 * 0.40) = $4,000.00.
Assume that when you go to sell the shares of XYZ, Inc., the new price per share is 0.50. If all 12,000 shares are sold at 0.50 per share, then the profit is calculated as (12000 * 0.50) - $4,000.00 = ş6, 000.00 -
4,000.00 = $2,000.00.
This last example is based on buying 100 shares of Google stock each year for the first five years (2007 - 2011), then selling all of them in March 2021:
Example 3 - GOOGL
Number of Shares Price per Share
100
85.00
100
140.00
100
191.68
100
255.08
100
230.00
The cost basis is calculated as follows: (100 * 85.00) + (100 * 140.00) + (100 * 191.68)
+ (100 * 255.08) + (100 * 230.00) = $90,176.00.
In March 2021, each share price of GOOGL was valued at 2011.41. If all 500 shares are sold at 2011.41 per share, then the profit is calculated as (500 * 2011.41) - $90,176.00 = $1,005,705.00 - $90,176.00 =
$915,529.00.
Assignment
For this project, you get to design and write a Stock class to keep track of the profit of stock purchases. You also get to design and write StockDriver class that requests input from the user and interacts with the stock
class. Your stock class Should store the following information:
• Total number of shares purchased
• Total cost basis (defined above)
Provide the following methods in your stock class:
• No-argument constructor that initializes both the total number of shares purchased and the total cost basis
• addstockPurchase () that takes the number of shares purchased (as an int) and the price per share (as a double). This method should add the newly purchased shares to the total number of shares purchased and update
the cost basis (with the number of shared purchased multiplied by the price per share).
• getNumberofShares () returns the total number of shares purchased as an int
• getCostBasis () returns the total cost basis as a double, rounded to 2 decimals because it is money
• calculateProfit () that takes the price per share (at the time of the sale) (as a double) and returns the profit of selling all of the shares (as a double, rounded to 2 decimals because it is money)
Your StockDriver class Sshould create a Stock object to keep track of the user's input. First, it requests the number of stock purchases from the user. For each purchase, it prompts the user for the number of shares purchased
(a positive number greater than o0), then the price per share (a positive number greater than 0.0). While either of these inputs are negative or equal to o (or 0.0), it prompts the user again before proceeding with the next input.
Note, if the user provided an invalid number of shares purchased, the program repeatedly requests valid input before requesting the price per share.
After all purchases are inputted, the user is prompted for the the price per share at the time of sale (a positive number greater than 0.0). Again, if an invalid number is entered, the program should repeatedly request valid input.
Note, do not keep track of the cost basis in your stockDriver class. Instead, make the appropriate method calls using the stock object.
Each of the methods in your stock class will be tested using unit tests. Additionally, the output of your entire program will be tested with various inputs.
Examples (user input in bold text)
Example 1 (with invalid input)
Welcome to the Stocks Profit Calculator!
Please enter the number of stock puchases: 3
Please enter the number of shares purchased: -99
Please enter the number of shares purchased: 0
Please enter the number of shares purchased: 100
Please enter the price per share: 0.0
Please enter the price per share: -98.76
Please enter the price per share: 5.5
Please enter the number of shares purchased: -100
Please enter the number of shares purchased: 100o
Please enter the price per share: 5.15
Please enter the number of shares purchased: 100
Please enter the price per share: 6.16
The cost basis is $1681.0.
To calculate the profit, please enter the price per share of a sale: -6.25
To calculate the profit, please enter the price per share of a sale: 6.25
The profit from selling 300 shares at 6.25 each is $194.0.
Notice that multiple invalid values are entered and that the program keeps asking for a valid values until it gets a valid one. Also notice that the cost basis and profit are each rounded.
If you're trying to debug this example (and I hope that you would), you could temporarily display the cost basis after each valid price per purchased share is entered. That should give you the following values:
The current cost basis is $550.0.
The current cost basis is $1065.0.
The current cost basis is $1681.0.
Example 2 (with invalid input)
Welcome to the Stocks Profit Calculator!
Please enter the number of stock puchases: 4
Please enter the number of shares purchased: 25ø0
Please enter the price per share: 0.0
Please enter the price per share: -1.4
Please enter the price per share: -0.4
Please enter the price per share: -0.3
Please enter the price per share: -0.2
Please enter the price per share: -0.1
Please enter the price per share: 0.0
Please enter the price per share: 0.4
Please enter the number of shares purchased: 2000
Please enter the price per share: 0.5
Please enter the number of shares purchased: 5000
Please enter the price per share: 0.2
Please enter the number of shares purchased: 2500
Please enter the price per share: 0.4
The cost basis is $4000.0.
To calculate the profit, please enter the price per share of a sale: 0.5
The profit from selling 12000 shares at 0.5 each is $2000.0.
Example 3
Welcome to the Stocks Profit Calculator!
Please enter the number of stock puchases: 5
Please enter the number of shares purchased: 100
Please enter the price per share: 85.0
Please enter the number of shares purchased: 100o
Please enter the price per share: 140.0
Please enter the number of shares purchased: 100
Please enter the price per share: 191.68
Please enter the number of shares purchased: 100
Please enter the price per share: 255.08
Please enter the number of shares purchased: 100
Please enter the price per share: 230.0
The cost basis is $90176.0.
To calculate the profit, please enter the price per share of a sale: 2011.41
The profit from selling 500 shares at 2011.41 each is $915529.0.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
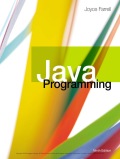
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
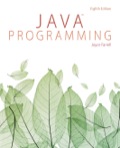
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
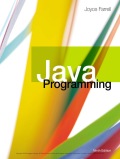
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
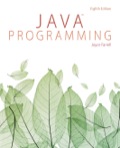
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781305480537
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT