Assume you're writing this menu-driven program for a swimming pool service company. There are three levels of customers: occasional, monthly, and yearly. There are two types of service: normal and deluxe a. As shown in Program 4-18, please declare constants for the levels of customers. Most C++ programmers write the names of constants in ALL CAPS; please do likewise in this program. b. Show the menu in a clear form, and prompt the user for the customer level; the rest of the program depends on the customer level chosen. c. 1. For occasional customers, ask for the type of service and the company cost (double) involved. If the service type is normal, then the price for the customer is 1.8 times the company cost. For deluxe service, the price for the customer is 2.5 times the company cost. 2. For monthly customers, ask for the type of service, and the company cost (double) involved. If the service type is normal, then the price for the customer is 1.5 times the company cost. For deluxe service, the price for the customer is 2 times the company cost. 3. For yearly customers, ask for the type of service and the company cost involved. If the service type is normal, then the price for the customer is 1.25 times the company cost. For deluxe service, the price for the customer is 1.5 times the company cost. For yearly customers, there is a 10% discount. 4. Please verify the user input each time the user enters a value. If the input is incorrect, set a flag to indicate that condition. You'll need the flag when you display output. d. Output. Here are several scenarios. The customer is an occasional one, service type is normal, and company cost is $137.68. The output should look like: Ex1: : Occasional : Normal Price to Customer : $247.82 Customer level Service type The customer is a yearly one, service type is deluxe, and company cost is $298.16. The output should look like: Ex2: : Yearly : Deluxe Customer level Service type Discount : 10% Price to Customer : $402.52 Ex3: The input is invalid for at least one of the prompts. The only output should be: There was invalid input. Please try again.
Addition of Two Numbers
Adding two numbers in programming is essentially the same as adding two numbers in general arithmetic. A significant difference is that in programming, you need to pay attention to the data type of the variable that will hold the sum of two numbers.
C++
C++ is a general-purpose hybrid language, which supports both OOPs and procedural language designed and developed by Bjarne Stroustrup. It began in 1979 as “C with Classes” at Bell Labs and first appeared in the year 1985 as C++. It is the superset of C programming language, because it uses most of the C code syntax. Due to its hybrid functionality, it used to develop embedded systems, operating systems, web browser, GUI and video games.
Please, I need to answer this question using the C++
Program 4-18
// This program displays a menu and asks the user to make a
// selection. An if/else if statement determines which item
// the user has chosen.
#include <iostream>
#include <iomanip>
using namespace std;
int main()
{
int choice; // To hold a menu choice
int months; // To hold the number of months
double charges; // To hold the monthly charges
// Constants for membership rates
const double ADULT = 40.0,
SENIOR = 30.0,
CHILD = 20.0;
// Constants for menu choices
const int ADULT_CHOICE = 1,
CHILD_CHOICE = 2,
SENIOR_CHOICE = 3,
QUIT_CHOICE = 4;
// Display the menu and get a choice.
cout << "\t\tHealth Club Membership Menu\n\n"
<< "1. Standard Adult Membership\n"
<< "2. Child Membership\n"
<< "3. Senior Citizen Membership\n"
<< "4. Quit the Program\n\n"
<< "Enter your choice: ";
cin >> choice;
// Set the numeric output formatting.
cout << fixed << showpoint << setprecision(2);
// Respond to the user's menu selection.
if (choice == ADULT_CHOICE)
{
cout << "For how many months? ";
cin >> months;
charges = months * ADULT;
cout << "The total charges are $" << charges << endl;
}
else if (choice == CHILD_CHOICE)
{
cout << "For how many months? ";
cin >> months;
charges = months * CHILD;
cout << "The total charges are $" << charges << endl;
}
else if (choice == SENIOR_CHOICE)
{
cout << "For how many months? ";
cin >> months;
charges = months * SENIOR;
cout << "The total charges are $" << charges << endl;
}
else if (choice == QUIT_CHOICE)
{
cout << "Program ending.\n";
}
else
{
cout << "The valid choices are 1 through 4. Run the\n"
<< "program again and select one of those.\n";
}
return 0;
}
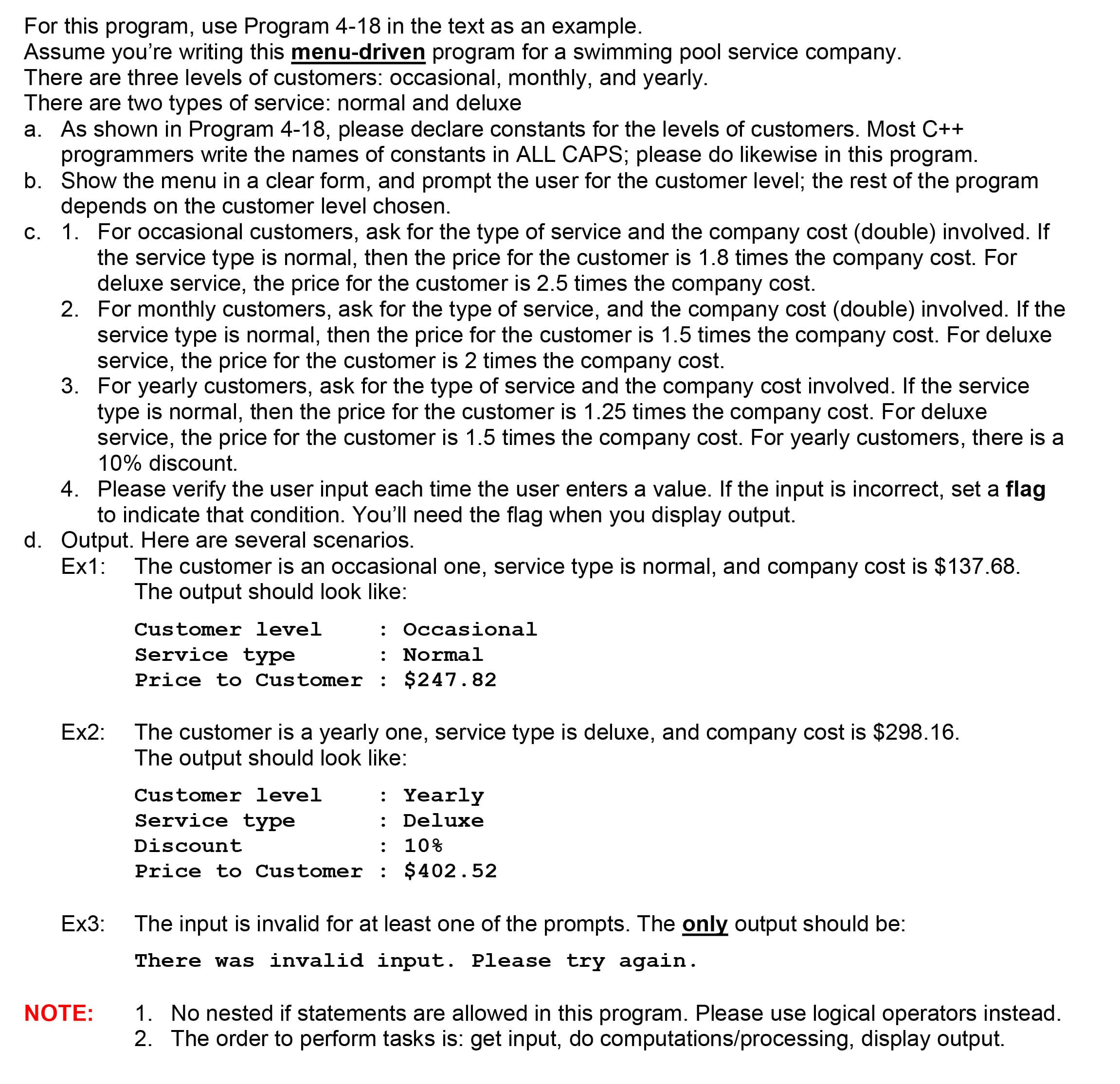

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

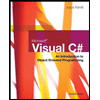
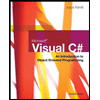