Assuming there are no deposits other than the original investment, the balance in a saving account after one year may be calculated as Rate Amount = Principal x (1+ T. %3D Principal is the balance in the savings account, Rate is the interest rate, and Tis the number of times the interest is compounded during a year (e.g. T is 4 if the interest is compounded quarterly). Write a program named saving.cpp that asks for the principal, the interest rate, and the number of times the interest is compounded. Compute the interest earned and the total amount in the saving account, and print the report shown in the task 2 sample output. Task 2 Sample Output: Please enter the principal amount: 1000.00 Please enter the interest rate: 4.25 Please enter the number of times compounded: 12 Interest Rate: Times Compounded: Principal: 4.254 12 1000.00 $ 43.34 1043.34 Interest: Amount in Savings: All the $ must be aligned Note: • Hint: Use library for pow () finction for equation auluulı
Assuming there are no deposits other than the original investment, the balance in a saving account after one year may be calculated as Rate Amount = Principal x (1+ T. %3D Principal is the balance in the savings account, Rate is the interest rate, and Tis the number of times the interest is compounded during a year (e.g. T is 4 if the interest is compounded quarterly). Write a program named saving.cpp that asks for the principal, the interest rate, and the number of times the interest is compounded. Compute the interest earned and the total amount in the saving account, and print the report shown in the task 2 sample output. Task 2 Sample Output: Please enter the principal amount: 1000.00 Please enter the interest rate: 4.25 Please enter the number of times compounded: 12 Interest Rate: Times Compounded: Principal: 4.254 12 1000.00 $ 43.34 1043.34 Interest: Amount in Savings: All the $ must be aligned Note: • Hint: Use library for pow () finction for equation auluulı
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter5: Repetition Statements
Section5.5: A Closer Look: Loop Programming Techniques
Problem 12E: (Program) Write a program that tests the effectiveness of the rand() library function. Start by...
Related questions
Question
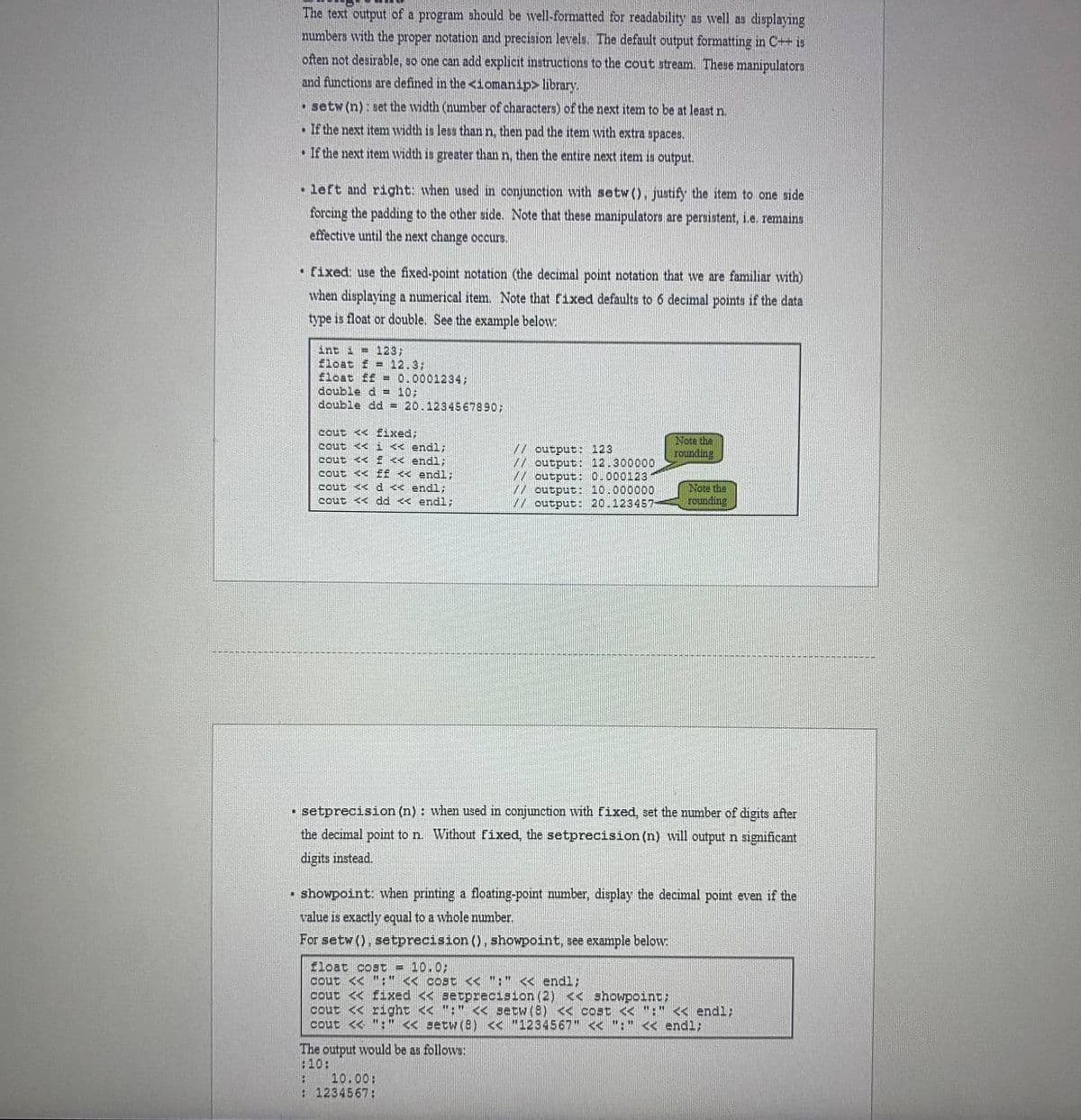
Transcribed Image Text:The text output of a program should be well-formatted for readability as well as displaying
numbers with the proper notation and precision levels. The default output formatting in C++ is
often not desirable, so one can add explicit instructions to the cout stream. These manipulators
and functions are defined in the <iomanip> library.
O setw (n): set the width (number of characters) of the next item to be at least n.
• If the next item width is less than n, then pad the item with extra spaces.
• If the next item width is greater than n, then the entire next item is output.
• left and right: when used in conjunction with setw (), justify the item to one side
forcing the padding to the other side. Note that these manipulators are persistent, i.e. remains
effective until the next change occurs.
• fixed: use the fixed-point notation (the decimal point notation that we are familiar with)
when displaying a numerical item. Note that fixed defaults to 6 decimal points if the data
type is float or double. See the example below:
int i = 123;
float f = 12.3;
float ff = 0.0001234;
double d = 10;
ble dd = 20.123456789o;
cout << £ixed;
cout << i < endl;
sout << E << endl;
Note the
roundine
// output: 123
// output: 12.300000
// output: 0.000123
// output: 10.000000
// output: 20.123457
cout << EE
cut << d << endl:
endl;
Note the
rounding
cout << dd << endl;
• setprecision (n) : when used in conjunction wvith fixed, set the number of digits after
the decimal point to n. Without fixed, the setprecision (n) will output n sigmificant
digits instead.
• showpoint: when printing a floating-point mumber, display the decimal point even if the
value is exactly equal to a whole number.
For setw (), setprecision (), showpoint, see example below:
float cost = 10.0;
cout << ":" < cost < ":" << endl;
cout << fixed << setprecision (2) < showpoint;
cout << right << ":" << setw (8) << cost << ":" << endl;
cout << ":" << setw(8) << "1234567" << ":" << endl;
The output would be as follows:
:10:
10.00:
: 1234567:
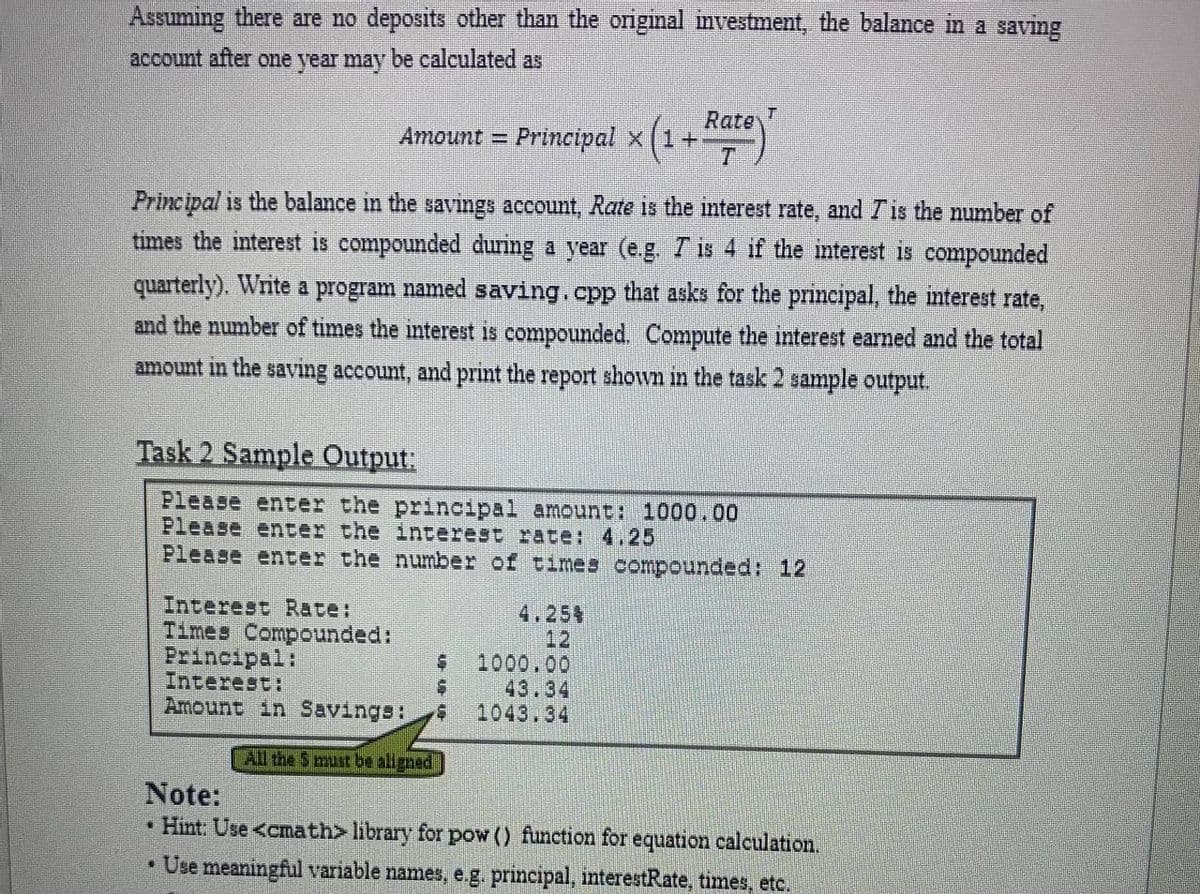
Transcribed Image Text:Assuming there are no deposits other than the original investment, the balance in a saving
account after one year may be calculated as
Rate
Amount = Principal x (1+
%3D
T.
Principal is the balance in the savings account, Rate is the nterest rate, and Tis the number of
times the interest is compounded during a year (e.g Tis 4 if the interest is compounded
quarterly). Write a program named saving.cpp that asks for the principal, the mterest rate,
and the number of times the interest is compounded. Compute the interest earned and the total
amount in the saving account, and print the report shown in the task 2 sample output.
Task 2 Sample Output:
Please enter the principal amount: 1000.00
Please enter the interest rate: 4.25
Please enter the number of times compounded: 12
Interest Rate:
Times Compounded:
Principal:
Interest:
Amount in Savings:
4.254
12
1000.00
43.34
1043.34
All the S must be aligned
Note:
• Hint: Use <emath> library for pow () function for equation calculation.
• Use meaningful variable names, e.g. principal, interestRate, times, etc.
Expert Solution

Step 1
I give the code in C++ along with code and output screenshot
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
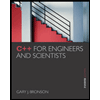
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
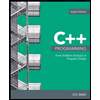
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
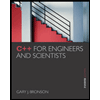
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
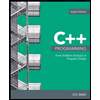
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning