in c programing Write a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the algorithm is: As long as x is greater than 0 Output x % 2 (remainder is either 0 or 1) x = x / 2 Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string. Ex: If the input is: 6 the output is: 110 The program must define and call the following two functions. Define a function named IntToReverseBinary that takes an int named integerValue as the first parameter and assigns the second parameter, binaryValue, with a string of 1's and 0's representing the integerValue in binary (in reverse order). Define a function named StringReverse that takes an input string as the first parameter and assigns the second parameter, reversedString, with the reverse of inputString. void IntToReverseBinary(int integerValue, char binaryValue[]) void StringReverse(char inputString[], char reversedString[])
in c programing
Write a program that takes in a positive integer as input, and outputs a string of 1's and 0's representing the integer in binary. For an integer x, the
Note: The above algorithm outputs the 0's and 1's in reverse order. You will need to write a second function to reverse the string.
Ex: If the input is:
6the output is:
110The program must define and call the following two functions. Define a function named IntToReverseBinary that takes an int named integerValue as the first parameter and assigns the second parameter, binaryValue, with a string of 1's and 0's representing the integerValue in binary (in reverse order). Define a function named StringReverse that takes an input string as the first parameter and assigns the second parameter, reversedString, with the reverse of inputString.
void IntToReverseBinary(int integerValue, char binaryValue[])
void StringReverse(char inputString[], char reversedString[])

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

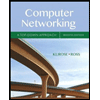
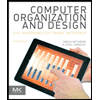
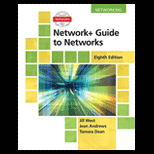
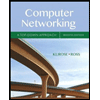
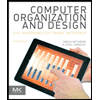
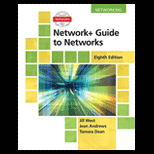
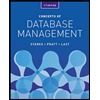
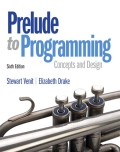
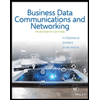