= ax? + bx+ c. • Ask the user for the coefficients a, b and c of a quadratic function f (x) : Output the results for x values of (0, 1, 2, .., 9), then x values of (10, 100, ..., 10,000,000). • You must write two functions: 1. A function that returns the value of the quadratic function given the coefficients and the value x. 2. A function that prints the value of the quadratic function given the coefficients and the value x. This function will call the first function. • You must use a for loop for the x values 0 to 9, and a while loop for the powers of ten. Example output looks like this: Enter coefficient a: 2.0 Enter coefficient b: 4.0 Enter coefficient c: 2.0 Parabola y = 2.0 x^2 + 4.0 x + 2.0 At x = 0, y - 2.0 At x = 1, y = 8.0 At x = 2, y = 18.0 At x = 3 , y = 32.0 At x = 4 , y = 50.0 At x = 5 , y - 72.0 At x = 6 , y = 98.0 At x = 7 , y - 128.0 At x = 8 , y - 162.0 At x = 9 , y = 200.0 At x = 10 , y = 242.0 At x = 100 At x = 1000 At x = 10000 , y = 200040002.0 At x - 100000 At x = 1000000 , y = 2000004000002.0 At x = 10000000 20402.0 y = 2004002.0 y y - 20000400002.0 y = 200000040000002.0
= ax? + bx+ c. • Ask the user for the coefficients a, b and c of a quadratic function f (x) : Output the results for x values of (0, 1, 2, .., 9), then x values of (10, 100, ..., 10,000,000). • You must write two functions: 1. A function that returns the value of the quadratic function given the coefficients and the value x. 2. A function that prints the value of the quadratic function given the coefficients and the value x. This function will call the first function. • You must use a for loop for the x values 0 to 9, and a while loop for the powers of ten. Example output looks like this: Enter coefficient a: 2.0 Enter coefficient b: 4.0 Enter coefficient c: 2.0 Parabola y = 2.0 x^2 + 4.0 x + 2.0 At x = 0, y - 2.0 At x = 1, y = 8.0 At x = 2, y = 18.0 At x = 3 , y = 32.0 At x = 4 , y = 50.0 At x = 5 , y - 72.0 At x = 6 , y = 98.0 At x = 7 , y - 128.0 At x = 8 , y - 162.0 At x = 9 , y = 200.0 At x = 10 , y = 242.0 At x = 100 At x = 1000 At x = 10000 , y = 200040002.0 At x - 100000 At x = 1000000 , y = 2000004000002.0 At x = 10000000 20402.0 y = 2004002.0 y y - 20000400002.0 y = 200000040000002.0
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section: Chapter Questions
Problem 7PP: (Numerical) Heron’s formula for the area, A, of a triangle with sides of length a, b, and c is...
Related questions
Question
The code is not printing the correct result. Please explain to me step by step.

Transcribed Image Text:= ax? + bx+c.
• Ask the user for the coefficients a, b and c of a quadratic function f (x)
• Output the results for x values of (0, 1, 2, .., 9), then x values of (10, 100, .., 10,000,000).
• You must write two functions:
1. A function that returns the value of the quadratic function given the coefficients and the value x.
2. A function that prints the value of the quadratic function given the coefficients and the value x. This function will call the first
function.
• You must use a for loop for the x values 0 to 9, and a while loop for the powers of ten.
Example output looks like this:
Enter coefficient a: 2.0
Enter coefficient b: 4.0
Enter coefficient c: 2.0
Parabola y = 2.0 x^2 + 4.0 x + 2.0
At x = 0.
At x = 1 y = 8.0
At x = 2 , y = 18.0
At x = 3 , y = 32.0
At x = 4 , y = 50.0
At x = 5 , y = 72.0
At x = 6
At x = 7 , y = 128.0
At x = 8
At x = 9
At x = 10 , y = 242.0
At x = 100
At x = 1000
At x = 10000
At x = 100000 , y = 20000400002.0
At x = 1000000 , y = 2000004000002.0
At x = 10000000
, y = 2.0
= 98.0
у%3D 162.0
y = 200.0
y = 20402.0
y = 2004002.0
y = 200040002.0
y =
200000040000002.0

Transcribed Image Text:Code screenshot
1 # function that returns the value of the quadratic function given the coefficients and the value x
2 def quadratic(a, b, c, x):
return a * x ** 2 + b * x + c
4
5 # function to print the value of a quadratic function given the coefficients and the value x
6 def print_quad(a, b, c, x):
print(f"At x = {x} , y = {quadratic(a, b, c, x)}")
8.
9 # get the coefficients from the user
10 a = float(input("Enter coefficient a: "))
11 b = float(input("Enter coefficient b: "))
12 c = float(input("Enter coefficient c: "))
I
13
14 # print the quadratic equation
15 print(f"Parabola y = {a} x^2 + {b} x + {c}")
16
17 # print the value of x and quadratic function for x values of (0, 1, 2, ... , 9)
18 for x in range(10):
19
print_quad(a, b, c, x)
20
21 # print the value of x and quadratic function for x values of (10, 100, ... , 10_000_000)
22 X = 10
23 power = 1
24 while power < 8:
print_quad(a, b, c, x**power)
power += 1
25
26
27
RMAL plot_quad.py
Sample output
(base) ~/chegg/src/526 $ python plot_quad.py
Enter coefficient a: 2.0
Enter coefficient b: 4.0
Enter coefficient c: 2.0
Parabola y = 2.0 x^2 + 4.0 x + 2.0
At x = 0
At x = 1
At x = 2
, y = 2.0
y = 8.0
y = 18.0
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
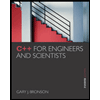
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
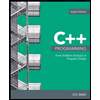
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
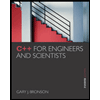
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
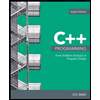
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning