. Find Relatively Prime Write a function to return a number that is relatively prime with the given number. /* return an integer that is relatively prime with n, and greater than 1 i.e., the gcd of the returned int and n is 1 Note: Although gcd(n,n-1)=1, we don't want to return n-1*/ int RelativelyPrime (int n) 4. Find Inverse Then implement the following function, which returns the inverse modulo, and test it. Note that you need to check whether a and n are relative prime before calling this function. Recall that a and n are relatively prime means that gcd(a,n)=1, i.e., their greatest common divisor is 1. Also recall that the extended Euclidean Algorithm can find the integer s and t for a and n such that as+nt=gcd(a,n), where s is the inverse of a modulo n. /* n>1, a is nonnegative */ /* a<=n */ /* a and n are relative prime to each other */ /* return s such that a*s mod n is 1 */ int inverse (int a, int n) { int s, t; int d = ExtednedEuclidAlgGCD (n, a, s, t); if (d==1) { return (mod (t, n)); // t might be negative, use mod() to reduce to // an integer between 0 and n-1 } else { cout <<"a and n are not relatively prime!\n"; } } -Please write in
3. Find Relatively Prime
Write a function to return a number that is relatively prime with the given number.
/* return an integer that is relatively prime with n, and greater than 1
i.e., the gcd of the returned int and n is 1
Note: Although gcd(n,n-1)=1, we don't want to return n-1*/
int RelativelyPrime (int n)
4. Find Inverse
Then implement the following function, which returns the inverse modulo, and test it.
Note that you need to check whether a and n are relative prime before calling this
function. Recall that a and n are relatively prime means that gcd(a,n)=1, i.e., their
greatest common divisor is 1. Also recall that the extended Euclidean
find the integer s and t for a and n such that as+nt=gcd(a,n), where s is the inverse
of a modulo n.
/* n>1, a is nonnegative */
/* a<=n */
/* a and n are relative prime to each other */
/* return s such that a*s mod n is 1 */
int inverse (int a, int n)
{
int s, t;
int d = ExtednedEuclidAlgGCD (n, a, s, t);
if (d==1)
{
return (mod (t, n)); // t might be negative, use mod() to reduce to
// an integer between 0 and n-1
}
else
{
cout <<"a and n are not relatively prime!\n";
}
}
-Please write in c++

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

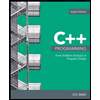
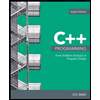