Below is my C++ code. What must be the pseudocode for this? #include using namespace std; int main () { int first_opt, area_opt, perimeter_opt, length, width, radius, side, height, base, a, b, c, A_Rect, A_Square, P_Rect, P_Square, P_Triangle; float A_Circle, A_Triangle, P_Circle; cout<<"Welcome!. Choose what you want to solve"<<"\n"; cout<<"1. AREA"<<"\n"; cout<<"2. PERIMETER"<<"\n"; cin>>first_opt; cout<<"Choose from the following"<<"\n"; switch(first_opt) { case 1: cout<<"1. Area of the Rectangle"<<"\n"; cout<<"2. Area of the Circle"<<"\n"; cout<<"3. Area of the Square"<<"\n"; cout<<"4. Area of the Triangle"<<"\n"; cin>>area_opt; switch(area_opt) { case 1: cout<<"Enter length: "<<"\n"; cin>>length; cout<<"Enter width: "<<"\n"; cin>>width; A_Rect = length*width; cout<<"The area of the rectangle is: "<>radius; A_Circle = 3.1416*radius*radius; cout<<"The area of the circle is: "<>side; A_Square = side*side; cout<<"The area of the square is: "<>height; cout<<"Enter base: "<<"\n"; cin>>base; A_Triangle = (height*base)/2; cout<<"The area of the triangle is: "<>perimeter_opt; switch(perimeter_opt) { case 1: cout<<"Enter length: "<<"\n"; cin>>length; cout<<"Enter width: "<<"\n"; cin>>width; P_Rect = 2*(length+width); cout<<"The perimeter of the rectangle is: "<>radius; P_Circle = 3.1416*radius*2; cout<<"The perimeter of the circle is: "<>side; P_Square = 4*side; cout<<"The perimeter of the square is: "<>a; cout<<"Enter the length of second side: "<<"\n"; cin>>b; cout<<"Enter the length of third side: "<<"\n"; cin>>c; P_Triangle = a+b+c; cout<<"The area of the triangle is: "<
Below is my C++ code. What must be the pseudocode for this?
#include <iostream>
using namespace std;
int main ()
{
int first_opt, area_opt, perimeter_opt, length, width, radius, side, height, base, a, b, c, A_Rect, A_Square, P_Rect, P_Square, P_Triangle;
float A_Circle, A_Triangle, P_Circle;
cout<<"Welcome!. Choose what you want to solve"<<"\n";
cout<<"1. AREA"<<"\n";
cout<<"2. PERIMETER"<<"\n";
cin>>first_opt;
cout<<"Choose from the following"<<"\n";
switch(first_opt)
{
case 1:
cout<<"1. Area of the Rectangle"<<"\n";
cout<<"2. Area of the Circle"<<"\n";
cout<<"3. Area of the Square"<<"\n";
cout<<"4. Area of the Triangle"<<"\n";
cin>>area_opt;
switch(area_opt)
{
case 1:
cout<<"Enter length: "<<"\n";
cin>>length;
cout<<"Enter width: "<<"\n";
cin>>width;
A_Rect = length*width;
cout<<"The area of the rectangle is: "<<A_Rect<<"\n";
case 2:
cout<<"Enter radius: "<<"\n";
cin>>radius;
A_Circle = 3.1416*radius*radius;
cout<<"The area of the circle is: "<<A_Circle<<"\n";
case 3:
cout<<"Enter side: "<<"\n";
cin>>side;
A_Square = side*side;
cout<<"The area of the square is: "<<A_Square<<"\n";
case 4:
cout<<"Enter height: "<<"\n";
cin>>height;
cout<<"Enter base: "<<"\n";
cin>>base;
A_Triangle = (height*base)/2;
cout<<"The area of the triangle is: "<<A_Triangle<<"\n";
default:
cout<<"out of options";
break;
}
break;
case 2:
cout<<"1. Perimeter of the Rectangle"<<"\n";
cout<<"2. Perimeter of the Circle"<<"\n";
cout<<"3. Perimeter of the Square"<<"\n";
cout<<"4. Perimeter of the Triangle"<<"\n";
cin>>perimeter_opt;
switch(perimeter_opt)
{
case 1:
cout<<"Enter length: "<<"\n";
cin>>length;
cout<<"Enter width: "<<"\n";
cin>>width;
P_Rect = 2*(length+width);
cout<<"The perimeter of the rectangle is: "<<P_Rect<<"\n";
case 2:
cout<<"Enter radius: "<<"\n";
cin>>radius;
P_Circle = 3.1416*radius*2;
cout<<"The perimeter of the circle is: "<<P_Circle<<"\n";
case 3:
cout<<"Enter side: "<<"\n";
cin>>side;
P_Square = 4*side;
cout<<"The perimeter of the square is: "<<P_Square<<"\n";
case 4:
cout<<"Enter the length of first side: "<<"\n";
cin>>a;
cout<<"Enter the length of second side: "<<"\n";
cin>>b;
cout<<"Enter the length of third side: "<<"\n";
cin>>c;
P_Triangle = a+b+c;
cout<<"The area of the triangle is: "<<P_Triangle<<"\n";
default:
cout<<"out of options";
break;
}
break;
default:
cout<<"out of options";
break;
}
return 0;
}

Step by step
Solved in 2 steps

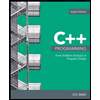
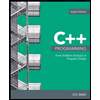