#Below is my Pizza class ,how would I write the function described in the attached image,based on this class? # The Pizza class should have two attributes(data items): class Pizza: # The Pizza class should have the following methods/operators): # __init__ - # constructs a Pizza of a given size (defaults to ‘M’) # and with a given set of toppings (defaults to empty set). def __init__(self, size='M', toppings=set()): self.size = size self.toppings = toppings # setSize – set pizza size to one of ‘S’,’M’or ‘L’ def setSize(self, size): self.size = size # getSize – returns size
#Python IDLE:
#Below is my Pizza class ,how would I write the function described in the attached image,based on this class?
# The Pizza class should have two attributes(data items):
class Pizza:
# The Pizza class should have the following methods/operators):
# __init__ -
# constructs a Pizza of a given size (defaults to ‘M’)
# and with a given set of toppings (defaults to empty set).
def __init__(self, size='M', toppings=set()):
self.size = size
self.toppings = toppings
# setSize – set pizza size to one of ‘S’,’M’or ‘L’
def setSize(self, size):
self.size = size
# getSize – returns size
def getSize(self):
return self.size
# addTopping – adds a topping to the pizza, no duplicates, i.e., adding ‘pepperoni’ twice only adds it once
def addTopping(self, topping):
self.toppings.add(topping)
# removeTopping – removes a topping from the pizza
def removeTopping(self, topping):
self.toppings.remove(topping)
# price – returns the price of the pizza according to the following scheme:
def price(self):
# ‘S’: $6.25 plus 70 cents per topping
if self.size == 'S':
return 6.25+0.7*len(self.toppings)
# ‘M’: $9.95 plus $1.45 per topping
elif self.size == 'M':
return 9.95+1.45*len(self.toppings)
# ‘L’: $12.95 plus $1.85 per topping
elif self.size == 'L':
return 12.95+1.85*len(self.toppings)
# __repr__ - returns representation as a string –
def __repr__(self):
# Pizza('L',{'anchovies', 'mushrooms', 'pepperoni'})
return f"Pizza('{self.size}',{self.toppings})"
# __eq__ - two pizzas are equal if they have the same size and same toppings
# (toppings don’t need to be in the same order)
def __eq__(self, value):
if self.size != value.size:
return False
if self.toppings != value.toppings:
return False
return True
# testing
pie = Pizza()
print(pie)
# Pizza('M', set())
pie.setSize('L')
print(pie.getSize())
# 'L'
pie.addTopping('pepperoni')
pie.addTopping('anchovies')
pie.addTopping('mushrooms')
print(pie)
# Pizza('L',{'anchovies', 'mushrooms', 'pepperoni'})
pie.addTopping('pepperoni')
print(pie)
# Pizza('L',{'anchovies', 'mushrooms', 'pepperoni'})
pie.removeTopping('anchovies')
print(pie)
# Pizza('L',{'mushrooms', 'pepperoni'})
pie.price()
# 16.65
pie2 = Pizza('L', {'mushrooms', 'pepperoni'})
print(pie2)
# Pizza('L',{'mushrooms', 'pepperoni'})
print(pie == pie2)
# True
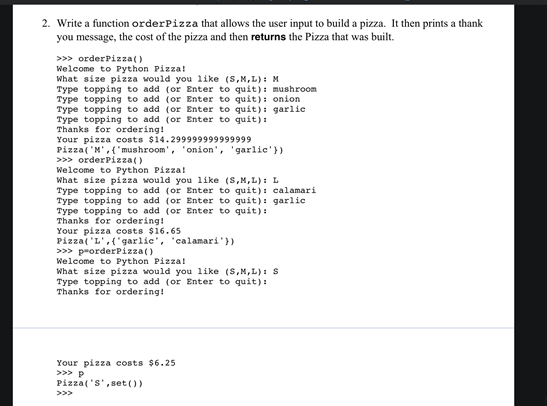

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

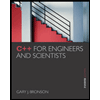
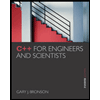