binary search tree c++)i am having issues with my code i am able to insert names into into the tree but they do not save(it says tree is empty). i will show you a picture of my output(black background) and a picture of the desired output(white background), i will also include my source main and .h class. //bt.cpp - file contains member functions #include "bt.h"
(binary search tree c++)i am having issues with my code i am able to insert names into into the tree but they do not save(it says tree is empty). i will show you a picture of my output(black background) and a picture of the desired output(white background), i will also include my source main and .h class.
//bt.cpp - file contains member functions
#include "bt.h"
#include <iostream>
using namespace std;
//Constructor
BT::BT()
{
root = NULL;
}
//Insert new item in BST
void BT::insert(string d)
{
node* t = new node;
node* parent;
t->data = d;
t->left = NULL;
t->right = NULL;
parent = NULL;
if (isEmpty())
root = t;
else
{
//Note: ALL insertions are as leaf nodes
node* curr;
curr = root;
// Find the Node's parent
while (curr)
{
parent = curr;
if (t->data > curr->data)
curr = curr->right;
else
curr = curr->left;
}
if (t->data < parent->data)
parent->left = t;
else
parent->right = t;
}
}
//Function - Preorder traversing driver
void BT::print_preorder()
{
preorderTrav(root);
}
//Function - Preorder traversing
void BT::preorderTrav(node* p)
{
if (isEmpty())
{
cout << " Tree is empty." << endl;
return;
}
if (p != NULL)
{
cout << " " << p->data;
if (p->left != NULL)
cout << " " << p->left->data;
else
cout << " NIL";
if (p->right != NULL)
cout << " " << p->right->data << endl;
else
cout << " NIL" << endl;
preorderTrav(p->left);
preorderTrav(p->right);
}
else
return;
}
//Function - Inorder traversing driver
void BT::print_inorder()
{
inorderTrav(root);
}
//Function - Inorder traversing
void BT::inorderTrav(node* p)
{
if (isEmpty())
{
cout << " Tree is empty." << endl;
return;
}
if (p != NULL)
{
inorderTrav(p->left);
cout << " " << p->data;
if (p->left != NULL)
cout << " " << p->left->data;
else
cout << " NIL";
if (p->right != NULL)
cout << " " << p->right->data << endl;
else
cout << " NIL" << endl;
inorderTrav(p->right);
}
else
return;
}__________________________________________________________________________
#include "bt.h"
#include<string>
#include <iostream>
using namespace std;
int main()
{
BT b;
int ch, num;
string d;
cout << "-----------Menu-------------" << endl;
cout << endl;
cout << "1. Insert node(s)" << endl;
cout << "2. Traverse Preorder" << endl;
cout << "3. Traverse Inorder" << endl;
cout << "4. Traverse Inorde" << endl;
cout << "5. Quit" << endl << endl;
do
{
cout << endl << "Enter Your Choice <1 - 5> ";
cin >> ch;
switch (ch)
{
case 1:
cout << "Enter number of nodes to insert: ";
cin >> num;
for (int i = 0; i<num; i++)
{
cout << endl;
cout << "Enter node: ";
cin >> d;
//b.insert(toupper(d));
cout << "Inserted." << endl;
}
break;
case 2:
cout << endl;
cout << "Traversing Preorder" << endl;
cout << " Node Info Left Child Info Right Child Info" << endl;
cout << " --------- --------------- ----------------" << endl;
b.print_preorder();
cout << endl;
break;
case 3:
cout << endl;
cout << "Traversing Inorder" << endl;
cout << " Node Info Left Child Info Right Child Info" << endl;
cout << " --------- --------------- ----------------" << endl;
b.print_inorder();
cout << endl
__________________________________________________________________________
#include <iostream>
#ifndef BT_H
#define BT_H
using namespace std;
class BT
{
private:
struct node
{
string data; //whatever is the data type; use that
node* left;
node* right;
};
node* root;
public:
BT(); //Constructor
bool isEmpty() const { return root == NULL; } //Check for empty
void insert(string); //Insert item in BST
void print_preorder(); //Preorder traversing driver
void preorderTrav(node*); //Preorder traversing

Step by step
Solved in 5 steps with 10 images

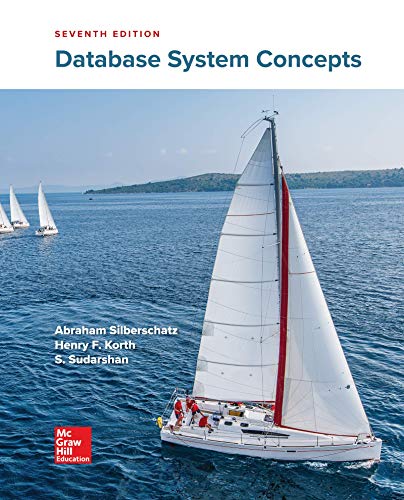
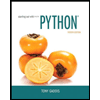
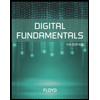
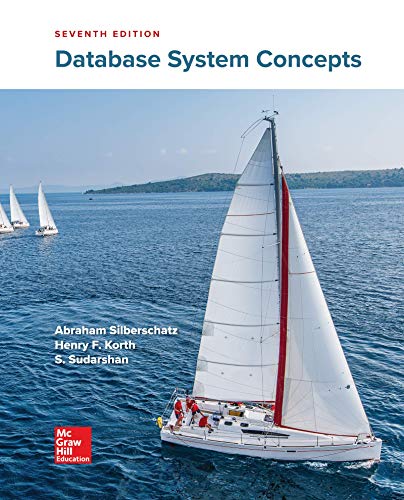
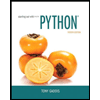
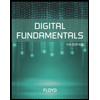
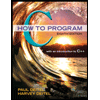
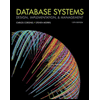
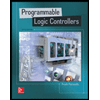