Bishop's Bridge There's construction on the bridge in front of Bishop's (the one that crosses into Lennoxville) and you've been charged with synchronizing the movement of vehicles across it from both directions. This means that you will only be able to let vehicles from one side cross when there are no vehicles from the other side crossing. You realize that you've just learned about semaphores and that they'd allow you to solve this problem effortlessly! Semaphores are simple counters so using them you can lock and control the number of cars on the bridge along with their direction. Start off by building a simple Car class like so: abstract class Car { private String name; Bridge Make a class called Bridge to represent the bridge that the cars will cross. It should have an array of Cars that are currently on it, and two semaphores: one for Bishops BoundCars, and another for LionsBoundCars. Create methods to add and remove cars from the array of cars on the Bridge. These methods should print the type of car being added or removed. Lastly, create a method to print all the cars on the bridge. Bishop's and Lion's Bound Cars Create two classes that represent the Cars moving in either direction that implement the Runnable interface and inherit from the Car class. These get initialized with the Bridge that they are crossing. In their run method they will both do the following in an infinite loop (while(true)): 1. Sleep for a random amount of time. a. The students driving to the Lion's drive 100 times faster than the ones travelling to In their run method they will both do the following in an infinite loop (while(true)): 1. Sleep for a random amount of time. a. The students driving to the Lion's drive 100 times faster than the ones travelling to Bishop's. 2. If there are no cars on the bridge, or the type of car on the bridge is the opposite type, then wait until this type can proceed with one of the semaphores. 3. Start driving over it. 4. Sleep for a bit. 5. 6. a. The students travelling to Bishop's take 4 times longer than the students going to the Lion's. Get off the bridge. If there are no cars left on the bridge, then let the other side go through. Test your program by creating a bridge with 3 Bishop's bound cars, and 2 Lion's bound cars associated to it. Start all of these cars in new threads and run them forever. Optionally, you could add a timeout to your while loops. Try modifying the number of permits you initialize the semaphores with to see what happens.
Bishop's Bridge There's construction on the bridge in front of Bishop's (the one that crosses into Lennoxville) and you've been charged with synchronizing the movement of vehicles across it from both directions. This means that you will only be able to let vehicles from one side cross when there are no vehicles from the other side crossing. You realize that you've just learned about semaphores and that they'd allow you to solve this problem effortlessly! Semaphores are simple counters so using them you can lock and control the number of cars on the bridge along with their direction. Start off by building a simple Car class like so: abstract class Car { private String name; Bridge Make a class called Bridge to represent the bridge that the cars will cross. It should have an array of Cars that are currently on it, and two semaphores: one for Bishops BoundCars, and another for LionsBoundCars. Create methods to add and remove cars from the array of cars on the Bridge. These methods should print the type of car being added or removed. Lastly, create a method to print all the cars on the bridge. Bishop's and Lion's Bound Cars Create two classes that represent the Cars moving in either direction that implement the Runnable interface and inherit from the Car class. These get initialized with the Bridge that they are crossing. In their run method they will both do the following in an infinite loop (while(true)): 1. Sleep for a random amount of time. a. The students driving to the Lion's drive 100 times faster than the ones travelling to In their run method they will both do the following in an infinite loop (while(true)): 1. Sleep for a random amount of time. a. The students driving to the Lion's drive 100 times faster than the ones travelling to Bishop's. 2. If there are no cars on the bridge, or the type of car on the bridge is the opposite type, then wait until this type can proceed with one of the semaphores. 3. Start driving over it. 4. Sleep for a bit. 5. 6. a. The students travelling to Bishop's take 4 times longer than the students going to the Lion's. Get off the bridge. If there are no cars left on the bridge, then let the other side go through. Test your program by creating a bridge with 3 Bishop's bound cars, and 2 Lion's bound cars associated to it. Start all of these cars in new threads and run them forever. Optionally, you could add a timeout to your while loops. Try modifying the number of permits you initialize the semaphores with to see what happens.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
I really need help on this, Just imagine that there’s construction on the bridge. In Java
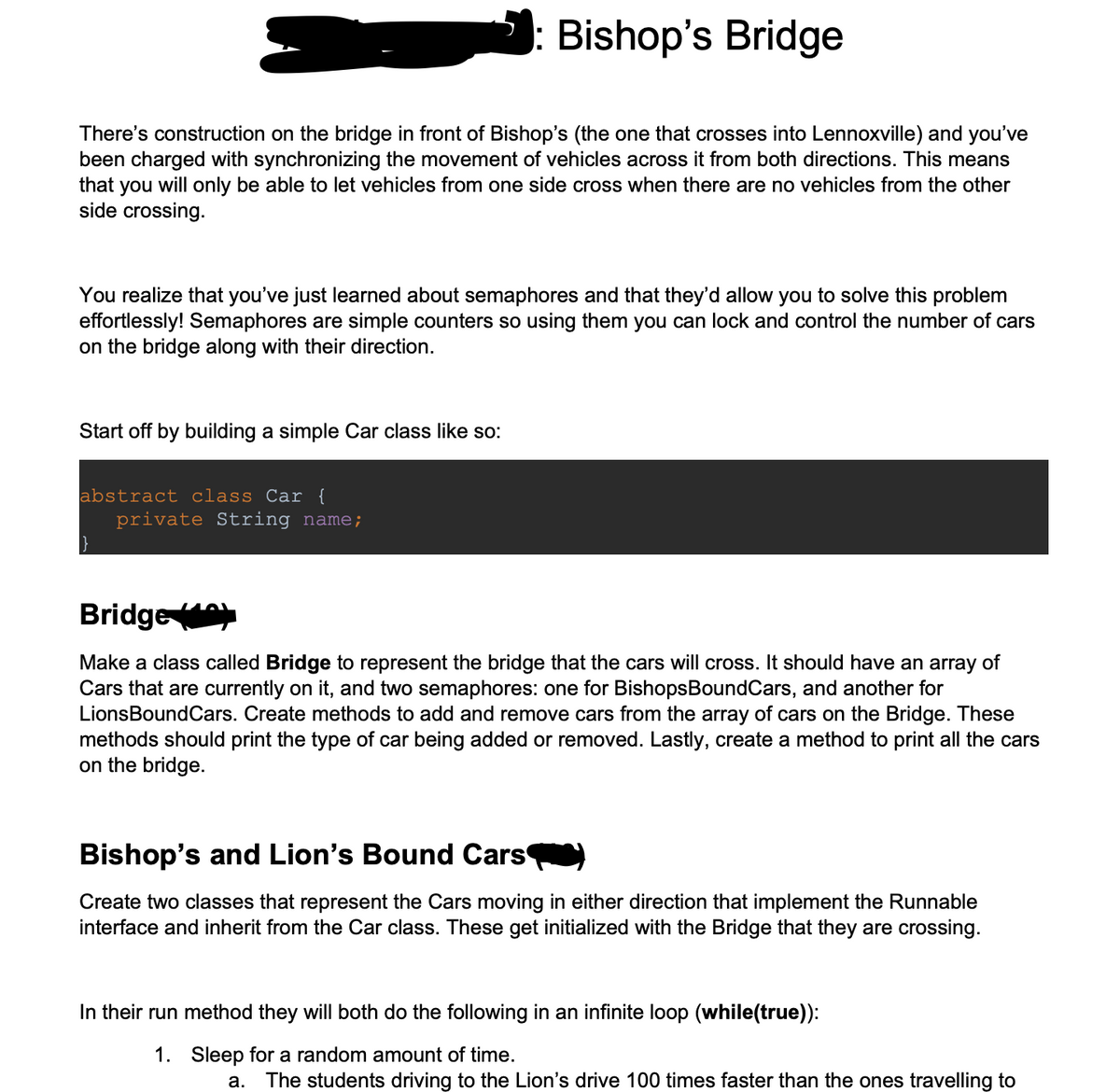
Transcribed Image Text:Bishop's Bridge
There's construction on the bridge in front of Bishop's (the one that crosses into Lennoxville) and you've
been charged with synchronizing the movement of vehicles across it from both directions. This means
that you will only be able to let vehicles from one side cross when there are no vehicles from the other
side crossing.
You realize that you've just learned about semaphores and that they'd allow you to solve this problem
effortlessly! Semaphores are simple counters so using them you can lock and control the number of cars
on the bridge along with their direction.
Start off by building a simple Car class like so:
abstract class Car {
private String name;
Bridge
Make a class called Bridge to represent the bridge that the cars will cross. It should have an array of
Cars that are currently on it, and two semaphores: one for Bishops BoundCars, and another for
LionsBoundCars. Create methods to add and remove cars from the array of cars on the Bridge. These
methods should print the type of car being added or removed. Lastly, create a method to print all the cars
on the bridge.
Bishop's and Lion's Bound Cars
Create two classes that represent the Cars moving in either direction that implement the Runnable
interface and inherit from the Car class. These get initialized with the Bridge that they are crossing.
In their run method they will both do the following in an infinite loop (while(true)):
1. Sleep for a random amount of time.
a.
The students driving to the Lion's drive 100 times faster than the ones travelling to
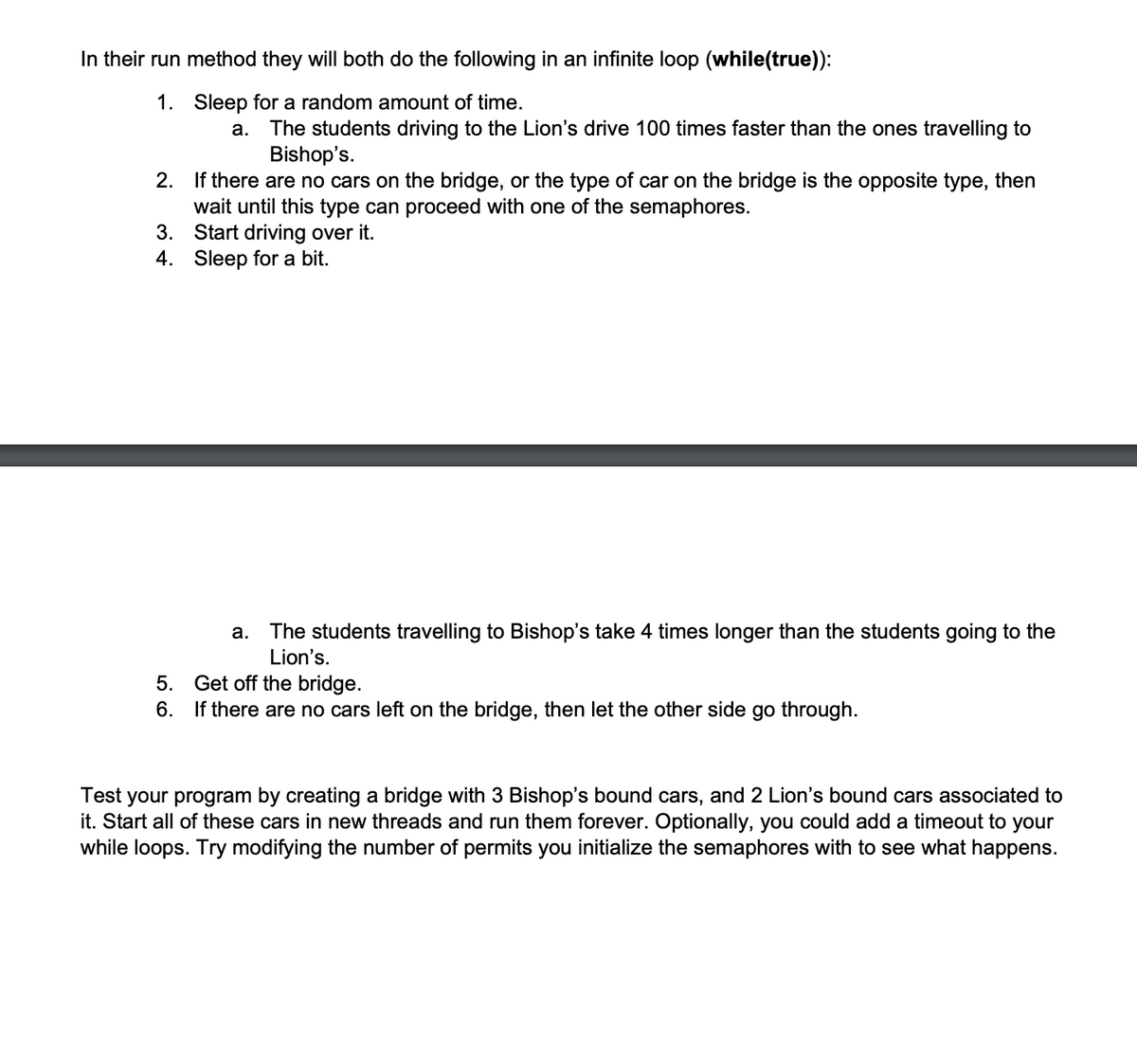
Transcribed Image Text:In their run method they will both do the following in an infinite loop (while(true)):
1. Sleep for a random amount of time.
a. The students driving to the Lion's drive 100 times faster than the ones travelling to
Bishop's.
2. If there are no cars on the bridge, or the type of car on the bridge is the opposite type, then
wait until this type can proceed with one of the semaphores.
3. Start driving over it.
4. Sleep for a bit.
5.
6.
a. The students travelling to Bishop's take 4 times longer than the students going to the
Lion's.
Get off the bridge.
If there are no cars left on the bridge, then let the other side go through.
Test your program by creating a bridge with 3 Bishop's bound cars, and 2 Lion's bound cars associated to
it. Start all of these cars in new threads and run them forever. Optionally, you could add a timeout to your
while loops. Try modifying the number of permits you initialize the semaphores with to see what happens.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
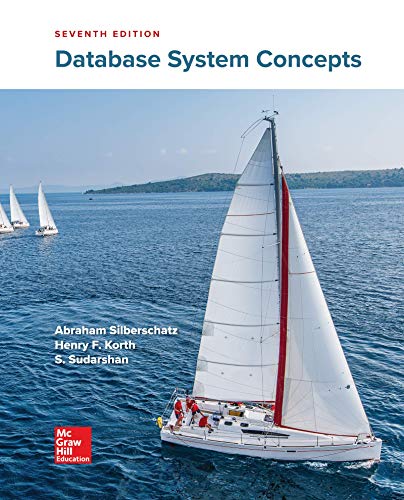
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
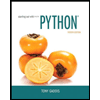
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
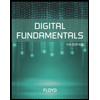
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
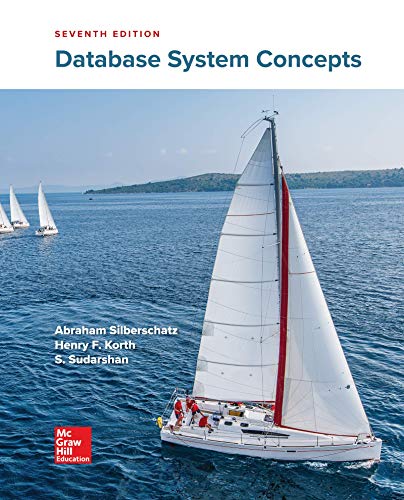
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
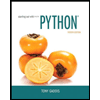
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
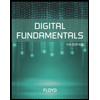
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
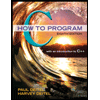
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
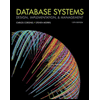
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
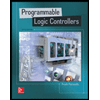
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education