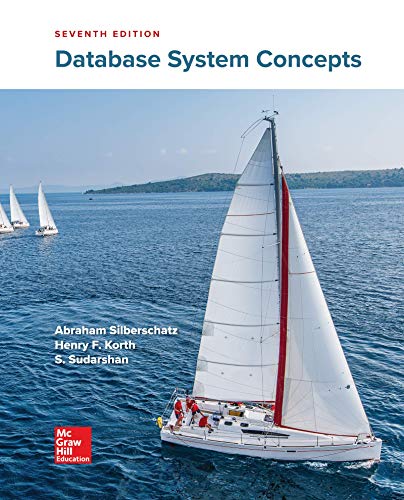
C Sharp How do I format my code to 2 decimal places whem I am calling my code in Main
using System.Threading.Tasks;
namespace ssullivan_A2_1.cs
{
class Program
{
//The output should display the Object Type (Circle, Square or Triangle)
//the values used to initialize the shape, the area, and the perimeter
static void Main(string[] args)
{ ////Store the objects in a Collection object (List, Array, HashSet, etc...) objects in the collection will be of type Shape
Shapes[] arrayOfShapes = new Shapes[3];
arrayOfShapes[0] = new Circle(5);//
arrayOfShapes[1] = new Rectangle(5, 5);//
arrayOfShapes[2] = new Triangle(3, 5, 5);//
//the values used to initialize the shape, the area, and the perimeter
foreach (Shapes objects in arrayOfShapes)
{// use arrayOfShapes to call methods
//The output should display the Object Type (Circle, Square or Triangle)
//the values used to initialize the shape, the area, and the perimeter
// use objects to call functions (
//GetType().Name} returns the name of the object
// use $ and { } when calling the object with the fubction
Console.WriteLine($"The area of {objects.GetType().Name} is: {objects.CalculateArea() }");
Console.WriteLine($"The perimeter of {objects.GetType().Name} is: {objects.CalculatePerimeter()}");
}
}
The area of Circle is: 19.6349540849362 // I want this to be 2 decimal places
The perimeter of Circle is: 15.707963267949 //I want this to be 2 decimal places
The area of Rectangle is: 25
The perimeter of Rectangle is: 20
The area of Triangle is: 0
The perimeter of Triangle is: 11
Press any key to continue . . .

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- 1 ) N integer array: long[] LongArr2) Method for entering integer values as follows: void input()- Number of integers: 3- Number one integer: 10 ...3) Method of ascending (order : 1) or descending (order : 2): void sort (int order)4) Code for creating and inputting class objects please c# codingarrow_forwardFix all the errors and send the code please // Application looks up home price // for different floor plans // allows upper or lowercase data entry import java.util.*; public class DebugEight3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String entry; char[] floorPlans = {'A','B','C','a','b','c'} int[] pricesInThousands = {145, 190, 235}; char plan; int x, fp = 99; String prompt = "Please select a floor plan\n" + "Our floorPlanss are:\n" + "A - Augusta, a ranch\n" + "B - Brittany, a split level\n" + "C - Colonial, a two-story\n" + "Enter floorPlans letter"; System.out.println(prompt); entry = input.next(); plan = entry.charAt(1); for(x = 0; x < floorPlans.length; ++x) if(plan == floorPlans[x]) x = fp; if(fp = 99) System.out.println("Invalid floor plan code entered")); else { if(fp…arrow_forwardTask- Median elements (C Language) Example #4 expected output is 5, but from the program below its coming out to 4. Please help make it come out to 5 as expected Given an array of integer elements, the median is the value that separates the higher half from the lower half of the values. In other words, the median is the central element of a sorted array. Since multiple elements of an input array can be equal to the median, in this task you are asked to compute the number of elements equal to the median in an input array of size N, with N being an odd number. Requirements Name your program project4_median.c. Follow the format of the examples below. The program will read the value of N, then read in the values that compose the array. These values are not necessarily sorted. The program should include the following function. Do not modify the function prototype. int compute_median(int *a, int n); a represents the input array, n is the length of the array. The function returns the…arrow_forward
- OOP (Object-Oriented Programming) in Java Applying the composite pattern, you may create a replica of any environment you choose. Any number of media may be used, from photographs to simulators to movies to video games, and so on.arrow_forwardThe provided file has syntax and/or logical errors. Determine the problem and fix the program.arrow_forwardOpenGL programming help using c++ The program should generate the square. The square should move in response to the left mouse button being held down and the mouse moved. The figure should stop at the defined window boundaries (N,S,E,W) making sure that the entire figure is always present in your window.arrow_forward
- Java Programming Assignment 6 – The Web Browser Web browsers are deceptively complex programs. The simple task of opening a web page carries a lot of steps: networking calls, protocol exchanges, rendering graphics, download and storage, JavaScript functionality, etc. It’s a lot to handle. The myriad number of tasks at hand provides a great example of different situations for collecting and managing information. Perfect for trying out the Java Collections Classes. Program requirements: • Create a new class, called “WebBrowser.” Inside it, place your main program. When run, your program will ask the user for commands. The following commands should be provided: o GOTO o DOWNLOAD o BACK o FORWARD o SHOW_DOWNLOADS o CLEAR_DOWNLOADS o SHOW_HISTORY o CLEAR_HISTORY o END • For the command above, should be any user-input String in the format of a typical web address. • Inside your WebBrowser main program, create the following: o A Stack of Strings called “backwards” o A Stack…arrow_forwardMorse Code (python ) Morse code maps each letter of the alphabet to a series of dashes and dots (a = .-). The list of the Morse code for all English letters (from a to z) is provided below: [".-","-...","-.-.","-..",".","..-.","--.","....","..",".---","-.-",".-..","- -","-.","---",".--.","--.-",".-.","...","-","..-","...-",".--","-..-","-.-- ","--.."] Your goal here is to implement a function that takes a list of strings as its only parameter (called “words”). Each of the words can be transformed to Morse code by concatenation of a number of the above strings (without spaces). For example, the transformation of “ab” will be “.--...” (“.-” + “-...”). The word “ems” will also have the same transformation “.--...” (“.” + “--” + “...”). In this function, the goal is to return the number of the unique transformations in “words” list. Here is an example (none of this is needed to be printed, the function will only return one integer): Words = [“ab”, “a”, “ems”]Returned value: 2Explanation:…arrow_forward@Build ER diagramarrow_forward
- First snippet public static void main(String[] args) { Dog aDog = new Dog("Max"); Dog oldDog = aDog; // we pass the object to foo foo(aDog); // aDog variable is still pointing to the "Max" dog when foo(...) returns aDog.getName().equals("Max"); // true aDog.getName().equals("Fifi"); // false aDog == oldDog; // true } public static void foo(Dog d) { d.getName().equals("Max"); // true // change d inside of foo() to point to a new Dog instance "Fifi" d = new Dog("Fifi"); d.getName().equals("Fifi"); // true } Second snippt public static void main(String[] args) { Dog aDog = new Dog("Max"); Dog oldDog = aDog; foo(aDog); // when foo(...) returns, the name of the dog has been changed to "Fifi" aDog.getName().equals("Fifi"); // true // but it is still the same dog: aDog == oldDog; // true } public static void foo(Dog d) { d.getName().equals("Max"); // true // this changes the name of d to be "Fifi" d.setName("Fifi"); } What is the difference between the first code snippet and the second?…arrow_forwardPointer variable stores the address where another object resides and system will automatically do garbage collection. Group of answer choices True Falsearrow_forwardComputer Science Part C: Interactive Driver Program Write an interactive driver program that creates a Course object (you can decide the name and roster/waitlist sizes). Then, use a loop to interactively allow the user to add students, drop students, or view the course. Display the result (success/failure) of each add/drop.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
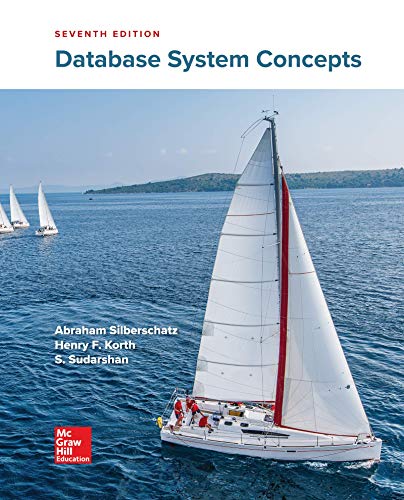
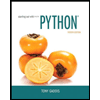
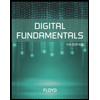
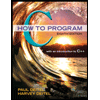
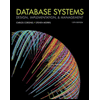
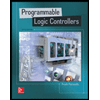