Breathe Well (PTY) LTD have contacted you to build a console-based system to keep track of any ventilator motherboards they build. To this end, you have to create a class named Motherboard (MB). For each MB they plan to keep track of the following values: • A count (integer) of the number of MB objects which have been created - this must be a read-only property • Name of the MB (string)-a read-only property Description of the MB (string) - a read-only property An "auto-generated" serial number (string) - a read-only property o The serial number must consist of a combination of the letters "VENTMB" and the current count of the number of MB objects which have been created, with leading zeroes. The serial number may never be less than 11 characters long; e.g. MB number 101 should have a serial number of VENTMBO0101. For all three the following; the properties have to ensure that no negative values are saved. If a negative value is received by the property, the value should be set to 5 (by the property). o Voltage (double) o Current (double) o Resistance (double) A MB must have the following constructors, which have to, at least, initialize all the of the class fields: 1. A constructor which receives the name of the MB, a description, the Voltage, the Current and Resistance. 2. A constructor which receives the name of the MB and a description. The Voltage, Current and Resistance should default to 5. This constructor should call the constructor described in 1. 3. A constructor which receives no parameters. The name and description should default to "Unnamed" and "No description". This constructor should call the constructor described in 2. A MB must contain a method DisplayMotherboard(), which returns a string containing the MB's name, description, serial number, voltage, current and resistance. It must be possible to call Array.Sort() on an array of MB objects. When sorting MB objects, they need to be sorted according to their names. It must be possible to use the "+" symbol to combine the details of two MB objects. When combining two MB objects, a new MB should be created with the following details: • A name which is the combination of both MB's names. A description which is the combination of both MB's description. • A completely new serial number. • The first MB's voltage. The second MB's current and resistance. Demonstrate that your Motherboard class works by writing the following Main program - you may hard code all values to whatever you choose, i.e. you don't need to capture user input: 1. Create an array of 5 Motherboard objects. 2. For the first element in the array assign a MB using the first constructor. 3. For the second element in the array assign a MB using the second constructor. 4. For the third element in the array assign a MB using the third constructor. 5. For the fourth element in the array assign a MB using object initialization to set the MB's Voltage and Current. 6. For the fifth element in the array assign the MB which is created when the first and fourth MB elements are combined. 7. Sort the MB array. 8. Display the name, description, serial number, voltage, current and resistance of each MB, in the array, to the console window.
Breathe Well (PTY) LTD have contacted you to build a console-based system to keep track of any ventilator motherboards they build. To this end, you have to create a class named Motherboard (MB). For each MB they plan to keep track of the following values: • A count (integer) of the number of MB objects which have been created - this must be a read-only property • Name of the MB (string)-a read-only property Description of the MB (string) - a read-only property An "auto-generated" serial number (string) - a read-only property o The serial number must consist of a combination of the letters "VENTMB" and the current count of the number of MB objects which have been created, with leading zeroes. The serial number may never be less than 11 characters long; e.g. MB number 101 should have a serial number of VENTMBO0101. For all three the following; the properties have to ensure that no negative values are saved. If a negative value is received by the property, the value should be set to 5 (by the property). o Voltage (double) o Current (double) o Resistance (double) A MB must have the following constructors, which have to, at least, initialize all the of the class fields: 1. A constructor which receives the name of the MB, a description, the Voltage, the Current and Resistance. 2. A constructor which receives the name of the MB and a description. The Voltage, Current and Resistance should default to 5. This constructor should call the constructor described in 1. 3. A constructor which receives no parameters. The name and description should default to "Unnamed" and "No description". This constructor should call the constructor described in 2. A MB must contain a method DisplayMotherboard(), which returns a string containing the MB's name, description, serial number, voltage, current and resistance. It must be possible to call Array.Sort() on an array of MB objects. When sorting MB objects, they need to be sorted according to their names. It must be possible to use the "+" symbol to combine the details of two MB objects. When combining two MB objects, a new MB should be created with the following details: • A name which is the combination of both MB's names. A description which is the combination of both MB's description. • A completely new serial number. • The first MB's voltage. The second MB's current and resistance. Demonstrate that your Motherboard class works by writing the following Main program - you may hard code all values to whatever you choose, i.e. you don't need to capture user input: 1. Create an array of 5 Motherboard objects. 2. For the first element in the array assign a MB using the first constructor. 3. For the second element in the array assign a MB using the second constructor. 4. For the third element in the array assign a MB using the third constructor. 5. For the fourth element in the array assign a MB using object initialization to set the MB's Voltage and Current. 6. For the fifth element in the array assign the MB which is created when the first and fourth MB elements are combined. 7. Sort the MB array. 8. Display the name, description, serial number, voltage, current and resistance of each MB, in the array, to the console window.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
C# Programming
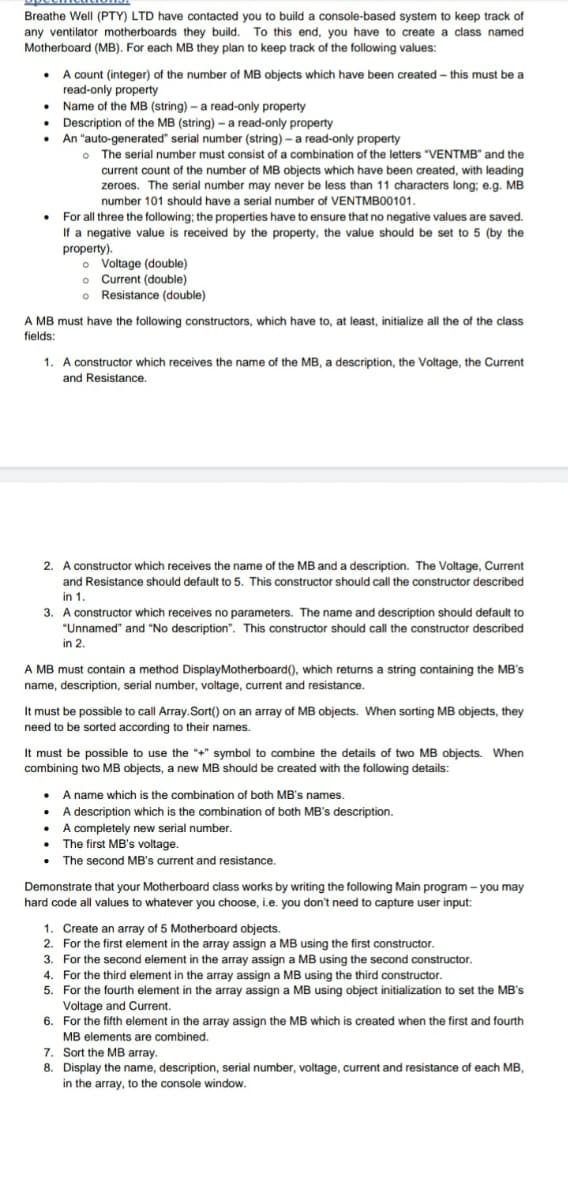
Transcribed Image Text:Breathe Well (PTY) LTD have contacted you to build a console-based system to keep track of
any ventilator motherboards they build. To this end, you have to create a class named
Motherboard (MB). For each MB they plan to keep track of the following values:
• A count (integer) of the number of MB objects which have been created – this must be a
read-only property
Name of the MB (string) – a read-only property
• Description of the MB (string) - a read-only property
An "auto-generated" serial number (string) – a read-only property
• The serial number must consist of a combination of the letters "VENTMB" and the
current count of the number of MB objects which have been created, with leading
zeroes. The serial number may never be less than 11 characters long; e.g. MB
number 101 should have a serial number of VENTMBO0101.
For all three the following; the properties have to ensure that no negative values are saved.
If a negative value is received by the property, the value should be set to 5 (by the
property).
o Voltage (double)
o Current (double)
o Resistance (double)
A MB must have the following constructors, which have to, at least, initialize all the of the class
fields:
1. A constructor which receives the name of the MB, a description, the Voltage, the Current
and Resistance.
2. A constructor which receives the name of the MB and a description. The Voltage, Current
and Resistance should default to 5. This constructor should call the constructor described
in 1.
3. A constructor which receives no parameters. The name and description should default to
"Unnamed" and "No description". This constructor should call the constructor described
in 2.
A MB must contain a method DisplayMotherboard(), which returns a string containing the MB's
name, description, serial number, voltage, current and resistance.
It must be possible to call Array.Sort() on an array of MB objects. When sorting MB objects, they
need to be sorted according to their names.
It must be possible to use the "+" symbol to combine the details of two MB objects. When
combining two MB objects, a new MB should be created with the following details:
• A name which is the combination of both MB's names.
• A description which is the combination of both MB's description.
• A completely new serial number.
• The first MB's voltage.
• The second MB's current and resistance.
Demonstrate that your Motherboard class works by writing the following Main program - you may
hard code all values to whatever you choose, i.e. you don't need to capture user input:
1. Create an array of 5 Motherboard objects.
2. For the first element in the array assign a MB using the first constructor.
3. For the second element in the array assign a MB using the second constructor.
4. For the third element in the array assign a MB using the third constructor.
5. For the fourth element in the array assign a MB using object initialization to set the MB's
Voltage and Current.
6. For the fifth element in the array assign the MB which is created when the first and fourth
MB elements are combined.
7. Sort the MB array.
8. Display the name, description, serial number, voltage, current and resistance of each MB,
in the array, to the console window.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
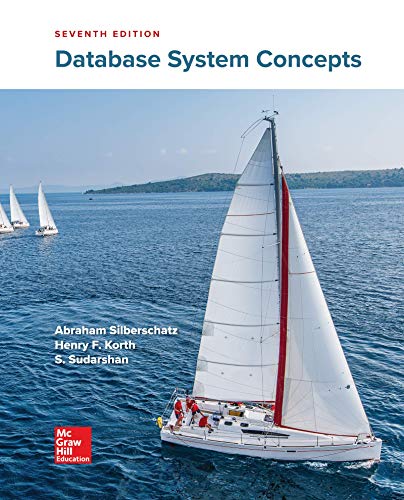
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
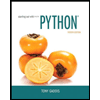
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
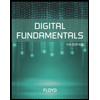
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
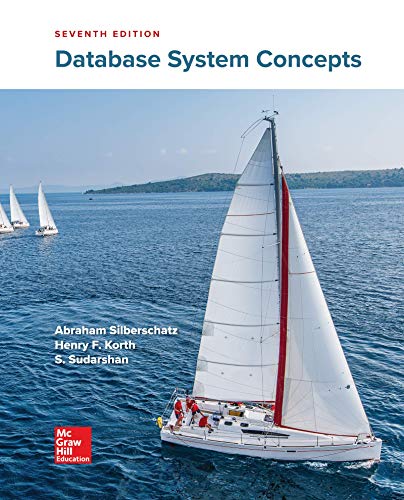
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
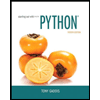
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
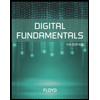
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
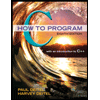
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
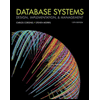
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
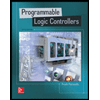
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education