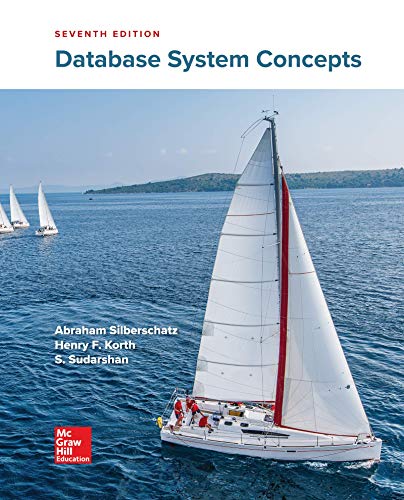
In this assignment, you will create a Java program to read a text file and count the frequency of its words.
1. The input file name is passed in as the arguments at command line. For example, assume your package
name is FuAssignment8 and your main class name is FuMain8, and your executable files are in
“C:\Users\2734848\eclipse-workspace\CIS 265 Assignments\bin”. The following command line will read
from a local file “cat.txt”:
C:\Users\2734848\eclipse-workspace\CIS 265 Assignments\bin > java FuAssignment8.FuMain8 cat.txt
2. If the program is run with incorrect number of arguments, your program must print an error message and
exit. The error message must show correct format to run your program, e.g., “Usage:
FuAssignment8.FuMain8 input_file” where FuAssignment8 is the package and FuMain8 is the main class.
3. The program will read from the file and count the occurrence of each word.
4. The program then prints the words and their frequencies in alphabetical order.
5. Given the following input file cat.txt:
you fit into me
like a hook into an eye
a fish hook
an open eye

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- When you compile and run packaged software from an IDE, the execution process can be as easy as clicking a run icon, as the IDE will maintain the classpath for you and will also let you know if anything is out of sorts. When you try to compile and interpret the code yourself from the command line, you will need to know exactly how to path your files. Let us start from c:\Code directory for this assignment. Consider a java file who's .class will result in the com.CITC1318.course package as follows: package com.CITC1318.course; public class GreetingsClass { public static void main(String[] args) { System.out.println("$ Greetings, CITC1318!"); } } This exercise will have you compiling and running the application with new classes created in a separate package: 1. Compile the program: c:\Code>javac -d . GreetingsClass.java 2. Run the program to ensure it is error-free: c:\Code>java -cp . com.CITC1318.course.GreetingsClass 3. Create three classes named Chapter1, Chapter2, and Chapter3…arrow_forwardWrite the code in java and understand what the question says and give me the code and don't copy or plagiarize pleasearrow_forwardCreate a file named Question.py and then create a class on it named Question. A Question object will contain information about a trivia question. It should have the following: A constructor, with the following parameters: 1 string to be the question itself (for example: "When was Cypress College Founded?"). A list of four strings, each a possible answer to the question (only one of them should be correct) An integer named 'answer': the index in the list of the correct answer. Example: if the third answer in the answer list is the correct one, the client should pass 2 to this parameter to signify the correct answer is in index 2. Overload the __str__ method. It should return a string made up of the question and the four possible answers. A ‘guess’ method, with an integer parameter. If the 'answer' attribute matches the int passed to 'guess', return True. Otherwise, False On Main.py, in your main function, create a list and add four Question objects to it. You may give them with…arrow_forward
- The files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. You also will use a file named DebugEmployeeIDException.java with the DebugTwelve4.java file. // An employee ID can't be more than 999 // Keep executing until user enters four valid employee IDs // This program throws a FixDebugEmployeeIDException import java.util.*; public class DebugTwelve4 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String inStr, outString = ""; final int MAX = 999; int[] emps = new int[4]; for(int x = 0; x < emps.length; ++x) { System.out.println("Enter employee ID number"); inStr = input.next(); try { emps[x] = Integer.parseInt(inStr); if(emps[x] > MAX) { throw(new…arrow_forwardWrite a function in python named "read_words" that declares a parameter for a filename and returns a collection of unique words in the file. Even though words.txt contains a single word per line, you should not assume that this is true of every file. For example, if the line is a sentence, you should split the line into individual words before storing each word in your collection. Handle any errors that occur by printing a detailed error message. Hint: the Python set works like Java's HashSet.arrow_forward11 11. You have imported a library with the birthMonth() function. Based on the API, how many strings are inputed to calculate the birth month? // calculate birth month based on the day of the month, day of the week, and the birth year // dayMonth {number} // dayWeek {string} - a day of a month from 1 to 31 - the name of the day of the week // year {number} -the birth year // return {string} - the month you were born BirthdayLibrary.birthMonth(dayMonth, dayWeek, year); А. 1 B. 4 C. O D. 3 ооarrow_forward
- Challenge 3: Encryption.java and Decryption.java Write an Encryption class that encrypts the contents of a binary file. Your encryption program should work like a filter, reading the contents of one file, modifying the data into a code, and then writing the coded content out to a second file. The second file will be a version of the first file, but written in a secret code. Use a simple encryption technique, such as reading one character at a time, and add 10 to the character code of each character before it is written to the second file. Come up with your own encryption method. Also write a Decryption class that reads the file produced by the Encryption program. The decryption file will use the method that you used and restore the data to its original state, and write it to a new file.arrow_forwardPlease help me code in java: Write a program that reads two files “Data1.txt”, “Data2.txt”; adding their corresponding elements produces an output file “output.txt”. If the number of elements are not equal, fill the elements of the smaller file up with “0” s. Sample output: Elements of the “Data1.txt”: 3 5 7 8 9 Elements of the “Data2.txt”: 45 11 Elements of the “Output.txt”: 48 16 7 8arrow_forwardWith no error Instructions The files provided in the code editor to the right contain syntax and/or logic errors. In each case, determine and fix the problem, remove all syntax and coding errors, and run the program to ensure it works properly. An example of the program is shown below: Enter a number >> 56 Enter a larger number >> 111 83.5 is halfway between 56 and 111 Task 1: The DebugSix4 class compiles without error. Task 2: The DebugSix4 program accepts user input and displays the correct output.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
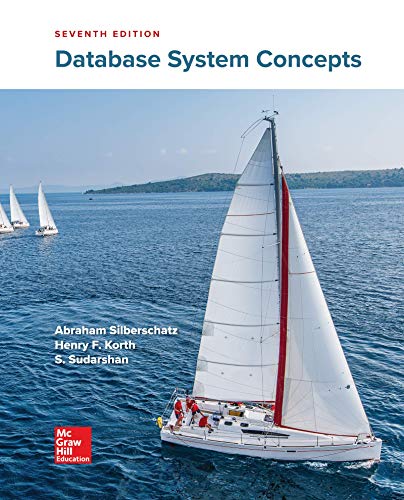
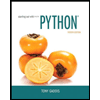
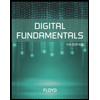
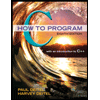
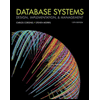
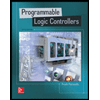