c++ hw In this hw, you're going to be working with partially filled arrays that are parallel with each other. That means that the row index in multiple arrays identifies different pieces of data for the same person. This is a simple payroll system that just calculates gross pay given a set of employees, hours worked for the week and hourly rate. code format: // TODO: Add function prototypes for all the declared functions // TODO: Declare two functions to compute the highestPay and lowestPay// HINT: Each should return the index of the correct value in the grossPay array. /* TODO: Create a function calledgetEmployeeNames Arguments : Name array(first and last name) Maximum size Return the number of names filled.*/ /* TODO: Create a function calledgetHourlyPay Arguments : NameArray(filled in Step 1) HourlyPayArray(starts empty) NumberOfNamesFilled(from Step1)*/ int main(){ // TODO: Declare an array of strings for the employeeNames able to hold a maximum of SIZE employees // TODO: Declare arrays of double hourlyPay and grossPay, each able to hold a maximum of SIZE employees // TODO: Declare a 2-D array of doubles for the hours. It should hold a maximum of SIZE employees, each having 7 days. // TODO: Read in the employee names and return the numberOfEmployees. // TODO: Call your function to read in the hourly pay for each of the employees. // TODO: Read in the daily hours for each employee. There will be 7 entries per week.// NOTE: This prints the headers with a set width for each field. Follow this format for the entriescout << setw(20) << "Employee Name" << setw(12) << " Hours" << setw(10) << "Gross Pay" << endl;-// TODO: For each employee, print: name, total hours and gross pay // TODO: Call the function that finds the highest gross pay and print the corresponding employee name and gross pay. // HINT: consider finding the Index of the value rather than returning the actual highest gross pay. // TODO: Call the function to find the lowest gross pay and print the corresponding employee name and gross pay. return 0;}
c++ hw
In this hw, you're going to be working with partially filled arrays that are parallel with each other. That means that the row index in multiple arrays identifies different pieces of data for the same person. This is a simple payroll system that just calculates gross pay given a set of employees, hours worked for the week and hourly rate.
code format:
// TODO: Add function prototypes for all the declared functions
// TODO: Declare two functions to compute the highestPay and lowestPay
// HINT: Each should return the index of the correct value in the grossPay array.
/* TODO: Create a function called
getEmployeeNames
Arguments :
Name array(first and last name)
Maximum size
Return the number of names filled.
*/
/* TODO: Create a function called
getHourlyPay
Arguments :
NameArray(filled in Step 1)
HourlyPayArray(starts empty)
NumberOfNamesFilled(from Step1)
*/
int main()
{
// TODO: Declare an array of strings for the employeeNames able to hold a maximum of SIZE employees
// TODO: Declare arrays of double hourlyPay and grossPay, each able to hold a maximum of SIZE employees
// TODO: Declare a 2-D array of doubles for the hours. It should hold a maximum of SIZE employees, each having 7 days.
// TODO: Read in the employee names and return the numberOfEmployees.
// TODO: Call your function to read in the hourly pay for each of the employees.
// TODO: Read in the daily hours for each employee. There will be 7 entries per week.
// NOTE: This prints the headers with a set width for each field. Follow this format for the entries
cout << setw(20) << "Employee Name" << setw(12) << " Hours" << setw(10) << "Gross Pay" << endl;
-
// TODO: For each employee, print: name, total hours and gross pay
// TODO: Call the function that finds the highest gross pay and print the corresponding employee name and gross pay.
// HINT: consider finding the Index of the value rather than returning the actual highest gross pay.
// TODO: Call the function to find the lowest gross pay and print the corresponding employee name and gross pay.
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 6 images

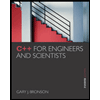
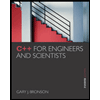