Write a void function that prints the list of nonnegative integers in reverse. Write a void function that prints all the numbers stored in the list that are greater than 10. It will also print the number of such numbers found. Write a function that determines the largest value stored in the array and returns that value to the calling function main. Write a function that determines the number of even integers stored in the array and returns that value to the calling function main. Write a function that calculates the average of the values stored in the array and returns that value. to the calling function main. Write the function calls with the appropriate arguments in the function main. Any values returned will be output. Write appropriate block comments for each function header similar to the ones provided. Show your understanding of the program and thus provide useful information to the reader. Test all the functions using appropriate test data. Use the following code and edit it where needed. #include //for cin, cout using namespace std; const int MAX_SIZE = 20; // maximum size of array //function prototypes void ReadList(int[], int&);
Write a void function that prints the list of nonnegative integers in reverse.
Write a void function that prints all the numbers stored in the list that are greater than 10. It will also print the number of such numbers found.
Write a function that determines the largest value stored in the array and returns that value to the calling function main.
Write a function that determines the number of even integers stored in the array and returns that value to the calling function main.
Write a function that calculates the average of the values stored in the array and returns that value. to the calling function main.
Write the function calls with the appropriate arguments in the function main. Any values returned will be output.
Write appropriate block comments for each function header similar to the ones provided. Show your understanding of the program and thus provide useful information to the reader.
Test all the functions using appropriate test data.
Use the following code and edit it where needed.
#include <iostream> //for cin, cout
using namespace std;
const int MAX_SIZE = 20; // maximum size of array
//function prototypes
void ReadList(int[], int&);
void PrintList(const int[], int);
int main()
{
int list[MAX_SIZE]; // array of nonnegative integers
int numberOfIntegers = 0; // number of nonnegative integers in array
ReadList(list, numberOfIntegers);
PrintList(list, numberOfIntegers);
//output your name etc.
cout << "123\n\n";
system("pause");
return 0;
}
//***************************************************************************
// Definition of function ReadList.
// This function reads nonnegative integers from the keyboard into an array.
// The parameter list is an array to hold nonnegative integers read.
//The parameter length is a reference parameter to an int. It holds the
// number of nonnegative integers read.
//***************************************************************************
void ReadList(int list[], int& length)
{
int number;
int index = 0;
cout << "\n\nEnter nonnegative integers each separated by a blank space,\n"
<< " and mark the end of the list with a negative number: ";
cin >> number; //read the first integer entered
//check that the number is nonnegative and
// the size of the array is not exceeded
while (number >= 0 && index < MAX_SIZE)
{
list[index] = number; //store the integer in the array
index++; // increment the index
cin >> number; // read the next integer
}
length = index; // length is the number of nonnegative integers
// in the list
}
//***************************************************************************
// Definition of function PrintList.
// The function prints the nonnegative integers in the array list and the
// number of integers in the array.
// The parameter list holds the nonnegative integers
// The parameter length holds thenumber of nonnegative integers.
//***************************************************************************
void PrintList(const int list[], int length)
{
int index;
cout << "\nThe list contains " << length
<< " nonnegative integer(s) as follows: \n";
for (index = 0; index < length; index++)
cout << list[index] << " ";
cout << endl;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

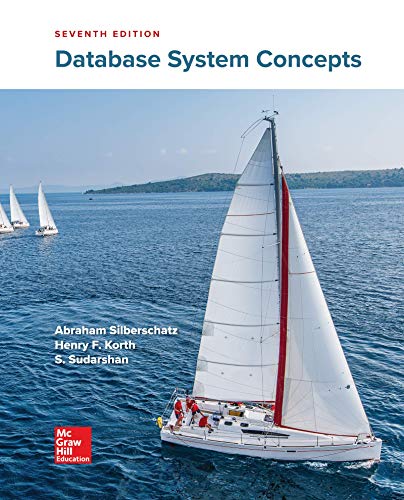
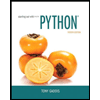
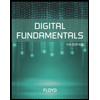
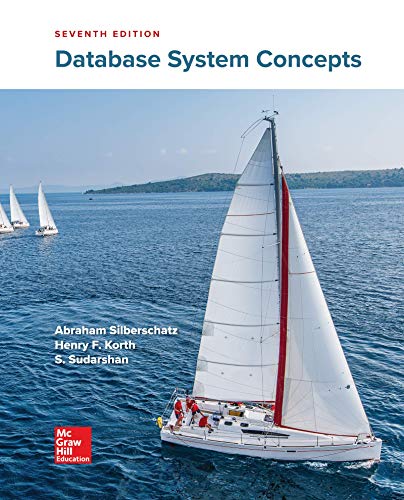
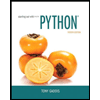
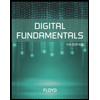
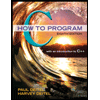
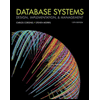
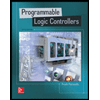