C lanaguge Managing Student Information! C LANGUAGE We're going to build a student information management system. The user should be shown a menu with four options: display student initials, display student GPAs, add students, or exit the program. If the user chooses to exit, the program should end. Otherwise, if the user chooses to display the student initials, the contents of the students.txt file should be read, and just the student number and initials should be displayed to the user in a tabular format. If the user chooses to display the student GPAs, the contents of the students.txt file should be read, and just the student number and GPAs should be displayed to the user in a tabular format. If the user chooses to add students, they should be prompted for the number of studnets they’d like to add. They should then be prompted that many times for a studnet number, a GPA, a first initial, and a last initial. Each of the new items should be saved to the students.txt file. Requirements main() Functionality: This main function should prompt the user for a menu option until they enter 4. It should decide, based on that menu option which actions to perform. Option 4 ends the program. Option 1 opens the students.txt file for reading and checks for a successful connection to the file stream. If it can, it will display the student initials, then close the file. Option 2 opens the students.txt file for reading and checks for a successful connection to the file stream. If it can, it will display the student GPAs, then close the file. Option 3 opens the studnets.txt for writing and checks for a successful connection to the file stream. If it can, it gets new students, then closes the file. Any other options should result in the error message. In addition to the main functions, your program should have 4 more functions: getMenuChoice() Input Parameters: none Returned Output: int Functionality: This function should display to the screen the options available to the user and return the users inputted menu choice. displayStudentInitials() Input Parameters: FILE pointer Returned Output: none Functionality: This function should accept a file pointer which has already been connected to a file stream. It should display a table of student numbers and student initials (with no spaces in between). Each of the values in the table should be read in from the students.txt file, but only the values specified should be in the table. displayStudentGpas() Input Parameters: FILE pointer Returned Output: none Functionality: This function should accept a file pointer which has already been connected to a file stream. It should display a table of student numbers and student GPAs (with a two decimal precision). Each of the values in the table should be read in from the students.txt file, but only the values specified should be in the table. addStudents() Input Parameters: FILE pointer Returned Output: none Functionality: This function should accept a file pointer which has already been connected to a file stream. It should prompt the user for the number of students they’d like to add. For each of those students, the user should be prompted for the five digit student number, gpa, first initial, and last initial, which should all be saved to the file.
C lanaguge
Managing Student Information! C LANGUAGE
We're going to build a student
The user should be shown a menu with four options: display student initials, display student GPAs, add students, or exit the
Requirements
main()
Functionality: This main function should prompt the user for a menu option until they enter 4. It should decide, based on that menu option which actions to perform. Option 4 ends the program. Option 1 opens the students.txt file for reading and checks for a successful connection to the file stream. If it can, it will display the student initials, then close the file. Option 2 opens the students.txt file for reading and checks for a successful connection to the file stream. If it can, it will display the student GPAs, then close the file. Option 3 opens the studnets.txt for writing and checks for a successful connection to the file stream. If it can, it gets new students, then closes the file. Any other options should result in the error message. In addition to the main functions, your program should have 4 more functions:
getMenuChoice()
Input Parameters: none
Returned Output: int
Functionality: This function should display to the screen the options available to the user and return the users inputted menu choice.
displayStudentInitials()
Input Parameters: FILE pointer
Returned Output: none
Functionality: This function should accept a file pointer which has already been connected to a file stream. It should display a table of student numbers and student initials (with no spaces in between). Each of the values in the table should be read in from the students.txt file, but only the values specified should be in the table.
displayStudentGpas()
Input Parameters: FILE pointer
Returned Output: none
Functionality: This function should accept a file pointer which has already been connected to a file stream. It should display a table of student numbers and student GPAs (with a two decimal precision). Each of the values in the table should be read in from the students.txt file, but only the values specified should be in the table.
addStudents()
Input Parameters: FILE pointer
Returned Output: none
Functionality: This function should accept a file pointer which has already been connected to a file stream. It should prompt the user for the number of students they’d like to add. For each of those students, the user should be prompted for the five digit student number, gpa, first initial, and last initial, which should all be saved to the file.


Purpose of the program: This program will add student information to a file and print the desired information on the console. The program prompts the user to enter choice and thus it must contain a menu for several operations.
Programming language: C
Source Code:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
//function to return choice
int getMenuChoice(){
int input;
printf("\n1 - Display students names");
printf("\n2 - Display students GPAs");
printf("\n3 - Add Students");
printf("\n4 - Exit\n");
scanf("%d",&input);
return input;
}
//display Student Initials from the file
void displayStudentInitials(FILE* ptr){
char stud_num[100],gpa[100],f_name[100],l_name[100];
printf("------------------------------\n");
printf("------------------------------\n");
printf("|| Student Num | Student Initial ||\n");
printf("------------------------------\n");
while (fscanf(ptr,"%s %s %s %s",stud_num,gpa,f_name,l_name) == 4 ) {
printf("|| %s | %s ||\n",stud_num,strcat(f_name,l_name));
printf("------------------------------\n");
}
printf("------------------------------\n");
}
//display Student GPA from the file
void displayStudentGpas(FILE* ptr){
char stud_num[100],gpa[100],f_name[100],l_name[100];
printf("------------------------------\n");
printf("------------------------------\n");
printf("|| Student Num | Student GPAs ||\n");
printf("------------------------------\n");
while (fscanf(ptr,"%s %s %s %s",stud_num,gpa,f_name,l_name) == 4 ) {
printf("|| %s | %s ||\n",stud_num,gpa);
printf("------------------------------\n");
}
printf("------------------------------\n");
}
//add new student to the file
void addStudents(FILE* ptr){
int num_st,st_no,i;
float gpa;
char f_init[100],l_init[100];
printf("How many student you want to add");
scanf("%d",&num_st);
for(i=0;i<num_st;i++){
printf("Please enter student Number\n");
scanf("%d",&st_no);
printf("Please enter student GPA\n");
scanf("%f",&gpa);
printf("Please enter student First initial\n");
scanf("%s",f_init);
printf("Please enter student Last initial\n");
scanf("%s",l_init);
fprintf(ptr,"\n%d\t%.2f\t%s\t%s",st_no,gpa,f_init,l_init);
}
}
int main()
{
int input;
FILE* ptr;
do{
input = getMenuChoice();
switch(input){
case 1:
ptr = fopen("studnets.txt","r");
if (ptr==NULL)
{
printf("no such file.");
return 0;
}
displayStudentInitials(ptr);
fclose(ptr);
break;
case 2:
ptr = fopen("studnets.txt","r");
if (ptr==NULL)
{
printf("no such file.");
return 0;
}
displayStudentGpas(ptr);
fclose(ptr);
break;
case 3:
ptr = fopen("studnets.txt","a");
if (ptr==NULL)
{
printf("no such file.");
return 0;
}
addStudents(ptr);
fclose(ptr);
break;
case 4:
exit(0);
}
}while(input != 4); //end do-while
return 0;
}
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

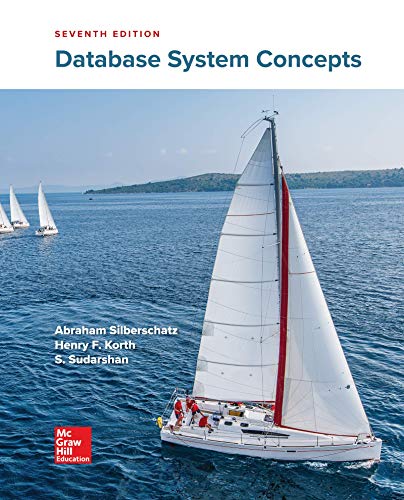
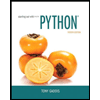
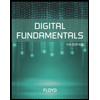
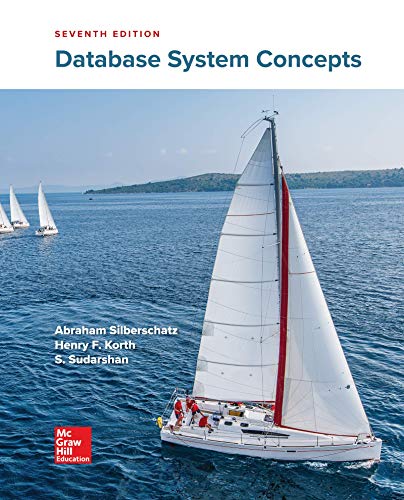
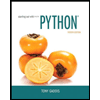
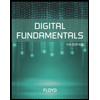
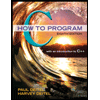
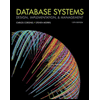
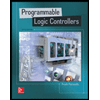