C++ - Please comment in code. Keep functions under 20 lines. Allowable libraries include: , , , , . Part 1: Text Surgeon Problem statement: You are tasked to write a program that reads in a sentence or a paragraph (max length 1023 letters) from the user, store it as a C-style string. Then the program gives the user several options for operations to perform on the text or quit. After performing an operation, the user should be prompted to select another option to operate on the original string until they select the option to quit. The program must handle all types of bad inputs from the user and recover from the errors. Detailed requirements for each choice are listed below: 1. Letter swap: the user inputs two letters, and the string is modified to replace all first letters in the text with the second letter. Example: User enters "Off we go, into the wild blue yonder!", then characters 'o' and 'i'. Program modifies the string to: Iff we gi, inti the wild blue yinder! (Note: capitalization is preserved) 2. Flip (reverse) the string: For this option, you need to create a new c-string from the heap, with exactly the size of the user's input string (not the max length 1023!), and then reverse it. Remember to clean up your heap! Example: User enters "ban ana54!"; program creates a new string and outputs: "! 45ana nab" Example: User enters "zip"; program creates a new string and outputs: "piz" 3. Words frequency: Prompt the user for an integer N, which represents for N words that the user wants to search for in the input string. Then prompt the user for these N words. Use a dynamic C++ string array allocated on the heap to store N words and output the frequency of each given words in the input string. Example: User enters "This is a test sentence, is this?", then 3 words, "this", "text", and "test". The program outputs: this: 2 text: 0 test: 1 (Note: Case insensitive, which means "word" and "WORD" are the same; All numbers and special characters in the string are ignored, which means "wor32rd", "wo]]]rd343.34", and "word" are the same) Suggested functions: a. char * purge_string (char *str); llaccepts a c-string, and returns a version where all numbers, spaces, and special characters removed (Note: the returned c-string must be on the heap, otherwise this c-string will be deleted once we leave this function) OR you may use this: void purge_string (char *str, char* str_new); llaccepts two c-strings, remove all spaces and special characters in str, and store the new string into str_new *Important note: You are not allowed to convert a C-style string to a C++ string object. In other words, you have to treat the input sentence (paragraph) as a C-style string and parse through it.
C++ - Please comment in code. Keep functions under 20 lines. Allowable libraries include: , , , , . Part 1: Text Surgeon Problem statement: You are tasked to write a program that reads in a sentence or a paragraph (max length 1023 letters) from the user, store it as a C-style string. Then the program gives the user several options for operations to perform on the text or quit. After performing an operation, the user should be prompted to select another option to operate on the original string until they select the option to quit. The program must handle all types of bad inputs from the user and recover from the errors. Detailed requirements for each choice are listed below: 1. Letter swap: the user inputs two letters, and the string is modified to replace all first letters in the text with the second letter. Example: User enters "Off we go, into the wild blue yonder!", then characters 'o' and 'i'. Program modifies the string to: Iff we gi, inti the wild blue yinder! (Note: capitalization is preserved) 2. Flip (reverse) the string: For this option, you need to create a new c-string from the heap, with exactly the size of the user's input string (not the max length 1023!), and then reverse it. Remember to clean up your heap! Example: User enters "ban ana54!"; program creates a new string and outputs: "! 45ana nab" Example: User enters "zip"; program creates a new string and outputs: "piz" 3. Words frequency: Prompt the user for an integer N, which represents for N words that the user wants to search for in the input string. Then prompt the user for these N words. Use a dynamic C++ string array allocated on the heap to store N words and output the frequency of each given words in the input string. Example: User enters "This is a test sentence, is this?", then 3 words, "this", "text", and "test". The program outputs: this: 2 text: 0 test: 1 (Note: Case insensitive, which means "word" and "WORD" are the same; All numbers and special characters in the string are ignored, which means "wor32rd", "wo]]]rd343.34", and "word" are the same) Suggested functions: a. char * purge_string (char *str); llaccepts a c-string, and returns a version where all numbers, spaces, and special characters removed (Note: the returned c-string must be on the heap, otherwise this c-string will be deleted once we leave this function) OR you may use this: void purge_string (char *str, char* str_new); llaccepts two c-strings, remove all spaces and special characters in str, and store the new string into str_new *Important note: You are not allowed to convert a C-style string to a C++ string object. In other words, you have to treat the input sentence (paragraph) as a C-style string and parse through it.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
Clearer instructions
![C++ - Please comment in code. Keep functions under
20 lines. Allowable libraries include: <iostream>,
<string>, <cstring>, <cstdlib>, <cmath>.
Part 1: Text Surgeon
Problem statement:
You are tasked to write a program that reads in a sentence or a paragraph (max length 1023 letters)
from the user, store it as a C-style string. Then the program gives the user several options for
operations to perform on the text or quit. After performing an operation, the user should be prompted to
select another option to operate on the original string until they select the option to quit. The program
must handle all types of bad inputs from the user and recover from the errors. Detailed requirements for
each choice are listed below:
1. Letter swap: the user inputs two letters, and the string is modified to replace all first letters in
the text with the second letter.
Example: User enters "Off we go, into the wild blue yonder!", then characters 'o'
and 'i'. Program modifies the string to: Iff we gi, inti the wild blue yinder!
(Note: capitalization is preserved)
2. Flip (reverse) the string: For this option, you need to create a new c-string from the heap, with
exactly the size of the user's input string (not the max length 1023!), and then reverse it.
Remember to clean up your heap!
Example: User enters "ban ana54!"; program creates a new string and outputs: "! 45ana
nab"
Example: User enters "zip"; program creates a new string and outputs: "piz"
3. Words frequency: Prompt the user for an integer N, which represents for N words that the user
wants to search for in the input string. Then prompt the user for these N words. Use a dynamic
C++ string array allocated on the heap to store N words and output the frequency of each given
words in the input string.
Example: User enters "This is a test sentence, is this?", then 3 words, "this",
"text", and "test". The program outputs:
this: 2
text: 0
test: 1
(Note: Case insensitive, which means "word" and "WORD" are the same; All numbers and special
characters in the string are ignored, which means "wor32rd", "wo]]rd343.34", and "word" are the
same)
Suggested functions:
a. char * purge_string (char *str); llaccepts a c-string, and returns a version where
all numbers, spaces, and special characters removed
(Note: the returned c-string must be on the heap, otherwise this c-string will be deleted once
we leave this function)
OR you may use this:
void purge_string (char *str, char* str_new); /laccepts two c-strings, remove
all spaces and special characters in str, and store the new string into str_new
*Important note:
You are not allowed to convert a C-style string to a C++ string object. In other words, you have to treat
the input sentence (paragraph) as a C-style string and parse through it.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8759b09c-a99c-4616-917a-1eb84cf5071d%2Fdc6bac23-dcd0-4018-a781-102d1a627220%2Ffhw1odq_processed.jpeg&w=3840&q=75)
Transcribed Image Text:C++ - Please comment in code. Keep functions under
20 lines. Allowable libraries include: <iostream>,
<string>, <cstring>, <cstdlib>, <cmath>.
Part 1: Text Surgeon
Problem statement:
You are tasked to write a program that reads in a sentence or a paragraph (max length 1023 letters)
from the user, store it as a C-style string. Then the program gives the user several options for
operations to perform on the text or quit. After performing an operation, the user should be prompted to
select another option to operate on the original string until they select the option to quit. The program
must handle all types of bad inputs from the user and recover from the errors. Detailed requirements for
each choice are listed below:
1. Letter swap: the user inputs two letters, and the string is modified to replace all first letters in
the text with the second letter.
Example: User enters "Off we go, into the wild blue yonder!", then characters 'o'
and 'i'. Program modifies the string to: Iff we gi, inti the wild blue yinder!
(Note: capitalization is preserved)
2. Flip (reverse) the string: For this option, you need to create a new c-string from the heap, with
exactly the size of the user's input string (not the max length 1023!), and then reverse it.
Remember to clean up your heap!
Example: User enters "ban ana54!"; program creates a new string and outputs: "! 45ana
nab"
Example: User enters "zip"; program creates a new string and outputs: "piz"
3. Words frequency: Prompt the user for an integer N, which represents for N words that the user
wants to search for in the input string. Then prompt the user for these N words. Use a dynamic
C++ string array allocated on the heap to store N words and output the frequency of each given
words in the input string.
Example: User enters "This is a test sentence, is this?", then 3 words, "this",
"text", and "test". The program outputs:
this: 2
text: 0
test: 1
(Note: Case insensitive, which means "word" and "WORD" are the same; All numbers and special
characters in the string are ignored, which means "wor32rd", "wo]]rd343.34", and "word" are the
same)
Suggested functions:
a. char * purge_string (char *str); llaccepts a c-string, and returns a version where
all numbers, spaces, and special characters removed
(Note: the returned c-string must be on the heap, otherwise this c-string will be deleted once
we leave this function)
OR you may use this:
void purge_string (char *str, char* str_new); /laccepts two c-strings, remove
all spaces and special characters in str, and store the new string into str_new
*Important note:
You are not allowed to convert a C-style string to a C++ string object. In other words, you have to treat
the input sentence (paragraph) as a C-style string and parse through it.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
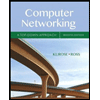
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
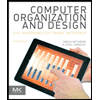
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
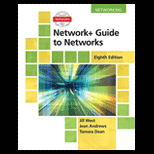
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
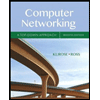
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
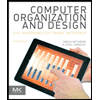
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
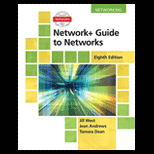
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
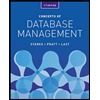
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
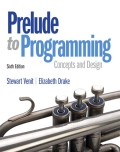
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
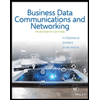
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY