C++ please. Upvote for good answers Question is located in main.cc ---------------------- bubble.h -------------------------- #include #include "cpputils/graphics/image.h" class Bubble { public: // Member functions of the Bubble class. // These member functions are called "setters" or "setter functions" // because they SET the member variables to the given input. void SetX(int x); void SetY(int y); void SetSize(int size); void SetColor(std::string color);. graphics::Color GetColor(); int GetX(); int GetY(); int GetSize(); std::string ToString(); private: // Member variables of the Bubble class. int x_ = 0; int y_ = 0; int size_ = 0; graphics::Color color_; }; --------------------------------- bubble.cc ------------------------------ #include "bubble.h" #include #include void Bubble::SetX(int x) { x_ = x; } void Bubble::SetY(int y) { y_ = y; } void Bubble::SetSize(int size) { size_ = size; } graphics::Color Bubble::GetColor() { return color_; } int Bubble::GetX() { return x_; } int Bubble::GetY() { return y_; } int Bubble::GetSize() { return size_; } void Bubble::SetColor(std::string color) { const int kMaxChannel = 255; const int kHalfChannel = 125; // Create a map from strings to graphics::Color. std::map colors; colors["red"] = graphics::Color(kMaxChannel, 0, 0); colors["orange"] = graphics::Color(kMaxChannel, kHalfChannel, 0); colors["yellow"] = graphics::Color(kMaxChannel, kMaxChannel, 0); colors["green"] = graphics::Color(0, kMaxChannel, 0); colors["teal"] = graphics::Color(0, kMaxChannel, kMaxChannel); colors["blue"] = graphics::Color(0, 0, kMaxChannel); colors["purple"] = graphics::Color(kHalfChannel, 0, kMaxChannel); if (colors.find(color) == colors.end()) { // Invalid color was passed in. Default to black. std::cout << "Invalid color: " << color << ". Defaulting to black." << std::endl; color_ = graphics::Color(0, 0, 0); } else { color_ = colors[color]; } } std::string Bubble::ToString() { return "Bubble at (" + std::to_string(x_) + ", " + std::to_string(y_) + ") with size " + std::to_string(size_); } ----------------------------------------------------------------------------------------------- main.cc ----------------- #include #include "bubble.h" #include "cpputils/graphics/image.h" int main() { // Prompts the user for input to create the Bubble image. std::cout << "Tell me about your bubble.\n" << "Valid x, y and size are between 0 and 500.\n" << "Valid colors are red, orange, yellow, green, " << "teal, blue, and purple." << std::endl; std::string color; std::cout << "What color? "; std::cin >> color; int size; std::cout << "What size? "; std::cin >> size; int x; std::cout << "What x? "; std::cin >> x; int y; std::cout << "What y? "; std::cin >> y; // ========================== Question here ============================= // TODO: Instantiate a `Bubble` object into a variable called `my_bubble`. // Then, use the member functions to set color, size, and x, y coordinates // based on the user provided input retrieved above. // ======================================================================= // Don't delete this! // This prints out your bubble to the terminal and also // draws it into an image you can view in "bubble.bmp". const int kImageSize = 500; graphics::Image image(kImageSize, kImageSize); if (image.DrawCircle(my_bubble.GetX(), my_bubble.GetY(), my_bubble.GetSize(), my_bubble.GetColor()) && image.SaveImageBmp("bubble.bmp")) { std::cout << my_bubble.ToString() << std::endl; std::cout << "Your bubble was saved to bubble.bmp" << std::endl; } return 0; }
C++ please. Upvote for good answers
Question is located in main.cc
----------------------
bubble.h
--------------------------
#include <string>
#include "cpputils/graphics/image.h"
class Bubble {
public:
// Member functions of the Bubble class.
// These member functions are called "setters" or "setter functions"
// because they SET the member variables to the given input.
void SetX(int x);
void SetY(int y);
void SetSize(int size);
void SetColor(std::string color);.
graphics::Color GetColor();
int GetX();
int GetY();
int GetSize();
std::string ToString();
private:
// Member variables of the Bubble class.
int x_ = 0;
int y_ = 0;
int size_ = 0;
graphics::Color color_;
};
---------------------------------
bubble.cc
------------------------------
#include "bubble.h"
#include <map>
#include <string>
void Bubble::SetX(int x) { x_ = x; }
void Bubble::SetY(int y) { y_ = y; }
void Bubble::SetSize(int size) { size_ = size; }
graphics::Color Bubble::GetColor() { return color_; }
int Bubble::GetX() { return x_; }
int Bubble::GetY() { return y_; }
int Bubble::GetSize() { return size_; }
void Bubble::SetColor(std::string color) {
const int kMaxChannel = 255;
const int kHalfChannel = 125;
// Create a map from strings to graphics::Color.
std::map<std::string, graphics::Color> colors;
colors["red"] = graphics::Color(kMaxChannel, 0, 0);
colors["orange"] = graphics::Color(kMaxChannel, kHalfChannel, 0);
colors["yellow"] = graphics::Color(kMaxChannel, kMaxChannel, 0);
colors["green"] = graphics::Color(0, kMaxChannel, 0);
colors["teal"] = graphics::Color(0, kMaxChannel, kMaxChannel);
colors["blue"] = graphics::Color(0, 0, kMaxChannel);
colors["purple"] = graphics::Color(kHalfChannel, 0, kMaxChannel);
if (colors.find(color) == colors.end()) {
// Invalid color was passed in. Default to black.
std::cout << "Invalid color: " << color << ". Defaulting to black."
<< std::endl;
color_ = graphics::Color(0, 0, 0);
} else {
color_ = colors[color];
}
}
std::string Bubble::ToString() {
return "Bubble at (" + std::to_string(x_) + ", " + std::to_string(y_) +
") with size " + std::to_string(size_);
}
-----------------------------------------------------------------------------------------------
main.cc
-----------------
#include <iostream>
#include "bubble.h"
#include "cpputils/graphics/image.h"
int main() {
// Prompts the user for input to create the Bubble image.
std::cout << "Tell me about your bubble.\n"
<< "Valid x, y and size are between 0 and 500.\n"
<< "Valid colors are red, orange, yellow, green, "
<< "teal, blue, and purple." << std::endl;
std::string color;
std::cout << "What color? ";
std::cin >> color;
int size;
std::cout << "What size? ";
std::cin >> size;
int x;
std::cout << "What x? ";
std::cin >> x;
int y;
std::cout << "What y? ";
std::cin >> y;
// ========================== Question here =============================
// TODO: Instantiate a `Bubble` object into a variable called `my_bubble`.
// Then, use the member functions to set color, size, and x, y coordinates
// based on the user provided input retrieved above.
// =======================================================================
// Don't delete this!
// This prints out your bubble to the terminal and also
// draws it into an image you can view in "bubble.bmp".
const int kImageSize = 500;
graphics::Image image(kImageSize, kImageSize);
if (image.DrawCircle(my_bubble.GetX(), my_bubble.GetY(), my_bubble.GetSize(),
my_bubble.GetColor()) &&
image.SaveImageBmp("bubble.bmp")) {
std::cout << my_bubble.ToString() << std::endl;
std::cout << "Your bubble was saved to bubble.bmp" << std::endl;
}
return 0;
}

Step by step
Solved in 2 steps

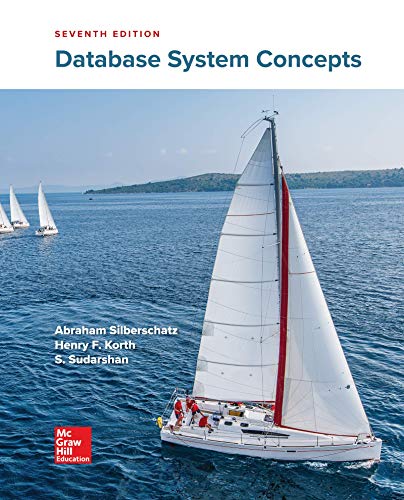
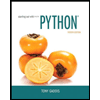
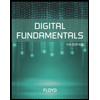
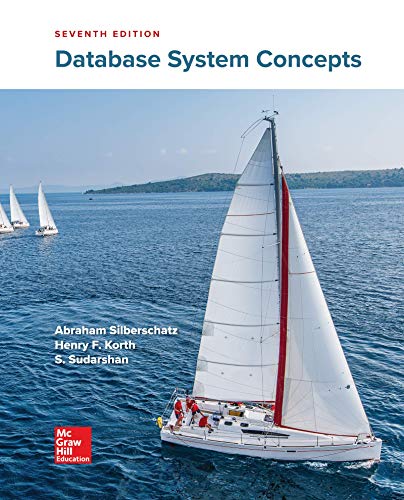
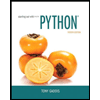
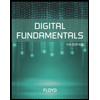
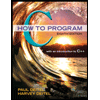
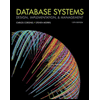
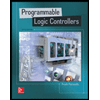